Question
Java Foundations Write a Java program that will read in an image from a file, rotate the image, and then write it out to a
Java Foundations
Write a Java program that will read in an image from a file, rotate the image, and then write it out to a file.
Work with the ASCII PPM image format. There are several details to the format, including:
The first line should contain "P3"
The second line (ignoring comment lines) should contain two integers, that correspond to the 'width' and 'height' of the image in number of pixels
The third line should be "255" as the maximum value of any pixel component.
After these lines, there will be N integers between 0 and 255. Where N = 3 * width * height. These integers can each be on one line, or multiple on the same line.
The first three numbers represent the "Red", "Green", and "Blue" component of the first pixel (top left, or at [0][0] ). The next three numbers are the components for the second pixel (top, 2nd from left, or at [0][1] ).
Create 3 classes "PPMImage", "Pixel", and "Main".
For the "PPMImage" class:
The PPMImage class should have a 2D array of Pixel objects ([height][width]).
The class should have a constructor that takes a filename as a parameter.
It should have a "rotate" instance method that rotates the image 90 degrees clockwise.
It should have a "write" instance method that takes a filename as a parameter and writes a valid .ppm file.
An InputMismatchException should be thrown by the constructor if it detects that the file given is not a properly formatted PPM image. Your Main method should catch this exception and react appropriately.
For the "Pixel" class:
The Pixel class should have three integer instance variables for "red", "green" and "blue".
It should have a constructor that takes three integers for these variables.
It should also have a "toString()" method that returns the "red", "green" and "blue" values (separated by a space or newline) as a String.
For the "Main" class:
The Main class should have the "main()" method and any private helper methods you want to make.
The main method should prompt the user for an input filename.
It should then instantiate a PPMImage object with that filename as input.
If the file does not exist, it should prompt the user to input the filename again.
If the file is not in PPM format, the program should state that, and ask for the filename again.
If the file is read in correctly, it should call "rotate()" on the image. Then prompt the user for an output filename, and call 'write()' on the image to output the file.
Hint: when reading in the pixel component values from the .ppm file, you can use 'Scanner.nextInt()'. You can also detect if the next line is a comment line by using the 'Scanner.hasNextInt()' function, if it is false then you could use 'Scanner.nextLine()' will read to the end of the line.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
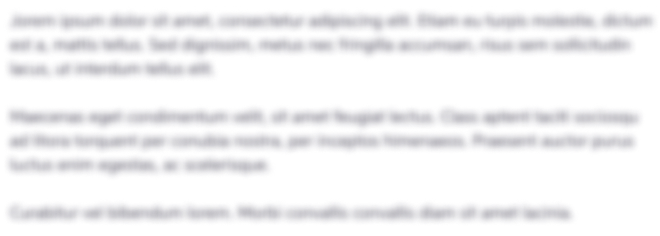
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started