Question
Java FX Application The purpose of this assignment is to get you familiar with the basics of the JavaFX Events. In particular you will need
Java FX Application
The purpose of this assignment is to get you familiar with the basics of the JavaFX Events. In particular you will need to be familiar with ActionEvents and MouseClicked events This game begins with all three cards showing the card back and it being right's turn to select a card. A card is selected by clicking the mouse on the middle card image. A card is randomly generated and assignment to the right label. The next card selected is assigned to the left. The two cards are then compared and the score updated on which ever card had a higher value. Ties are awarded no points but I should point out that in the deck the Ace of diamonds has a smaller value than the ace of spades. We will fix this later on. For now let's get the basic game going. | ![]() |
The Layout
The layout for this assignment uses a few different panes. To begin with, the root pane is set to a BorderPane. This will allow you to place other panes in top bottom, center, left or right. For this assignment only the top, center, and bottom are going to be used.
At the top of the game is the Labels and TextFields that hold the information about the progress of the game. You are going to need to create a GridPane for this. You can call it anything that you wish but topPane seems like a good name.
In the middle of the root layout is where the three card images are being displayed. This will also need to be a GridPane. Again, name this whatever you like but cardPane is what I will be referring to it as.
In the bottom of the root layout the reset button. It will simply be added without being apart of any other layout.
The Top Pane
In the field you are going to create all of the GUI components for the game. This needs to be done so that they can be referenced from the inner classes that make up the events.
To begin with you need to create a Label for the score. let's call it lblScore. It should have the text "Score:" inside it. Create a Font and a Color for the Label. You will apply these in the start method because you cannot do anything outside of creation of components in the field.
Create two TextFields one to hold Left's score and one to hold Right's score. Give them a meaningful name like tfLeft and tfRight.
In the start method for each of the TextFields call the setPrefWidth method giving a value of 50. Also call the setDisable method passing a value of true. Call the setText method and set the text to "0"
Also In the start method create the topPane (Remember, this is a GridPane) add lblScore to row 0 column 0. Don't forget to apply the Font and Color to it.
Add an informational anonymous label to the top topPane that says "Left: " to row 1 column 0. Add the TextField tfLeft to row 1 column 1.
Add an informational anonymous label to the top topPane that says "Right: " to row 1 column 2. Add the TextField tfRight to row 1 column 3.
Finally, set the Hgap of the topPane to 20 and the Vgap to 10 and add the topPane to the top of the root pane
The Center Pane
The cardPane has three Labels in it that hold each of the card images. Create three Labels in the field. You might want to consider calling them lblCardLeft, lblCardRight, and lblCardDeck. The middle card (lblCardDeck) is only going to hold the card back.
In the start method create the cardPane as a GridPane. Set the Hgap of the cardPane to 20.0. Add lblCardLeft to row 0 column 0, lblCardDeck to row 0 column 1, and lblCard right to row 0 column 2.
Set the alignment of cardPane to Pos.CENTER
Add the cardPane to the center of the root pane.
The Button
In the field create a Button called btnReset. Add the button to the bottom of the root pane. Don't worry about the event at this point we will come back to it.
Load Default Images
Create a new method called resetCardImages. Inside this method create three Images: imgCardLeft, imgCardRight, and imgCardDeck. Use the constructor to load image 155.gif for each of these. Call the setGraphic method on lblCardLeft, lblCardRight, and lblCardDeck. Set the graphic of each of them to a new ImageView. It should be something like this:
lblCardLeft.setGraphic(new ImageView(imgCardLeft));
Of course this needs to be done for all three cards
Inside the start method call the resetCardImages method you just completed. You are probably going to want to call this method before adding panes to the root pane.
Layout Check
At this point your layout should be done and your application should look like the one in the picture. Or at the very least similar depending on the creative license you took.
If your application does not look like the image above or does not run go back an fix any issues with if before moving on. If you cannot fix these issues then post questions in the discussion board. Be as specific as you can.
Mouse Click Event
At the top of the start method you are going to have to call the setOnMouseClicked method on lblCardDeck and set a new event handler to handle the game. The call should look something like this:
lblCardDeck.setOnMouseClicked(new EventHandler
}
});
Once this is done the variables to handle the logistics need to be added. In the field create variables called rightVal, leftVal, and score. Set each these to 0. You will also need a variable to handle who's turn it is. Create a boolean variable called rigthtsTrun and set it equal to true.
Inside the handle method generate a random number between 101 and 152 (don't forget to import java.util.Random) Construct the card name as you did in assignment 3 using the random number you just generated.
The logic then is as follows:
If rightsTurn is true
Set rightVal = to the random number generated for the card number
create a new Image from the card name you constructed
Set the graphic on lblCardRight to a new ImageView using the image you just created
else
Set leftVal = to the random number generated for the card number
create a new Image from the card name you constructed
Set the graphic on lblCardLeft to a new ImageView using the image you just created
if rightVal > leftVal
set score equal to value stored in tfRight
increment score by 1
Set the text in tfRight to the new score
else if leftVal > rightVal
set score equal to value stored in tfLeft
increment score by 1
Set the text in tfLeft to the new score
Flip who's turn it is by setting rightsTurn = !rightsTurn.
Note:
The else if is used here to get around the tie. If we simply used else a tie would go to the left
At this point you should have a working game with the exception of the Reset button. If your game does not work go back through the logic and see if you can correct things.
If the logic completely escapes you then please ask on the discussion board. Be as specific as possible.
The Reset Button
At the top of the start method you need to call the setOnAction method and setup a new event handler. Button events are covered in the lecture material.
Inside the handle method you need to set the following
rightVal to 0
leftVal to 0
score to 0
tfRight to "0"
tfLeft to "0"
rigthTurn to true
Finally call the resetCardImages method
Finishing Up
At this point you should have a working game. If not then you need go back over your code and work on any bugs that you may have. If you get stuck then you need to ask questions on the discussion board. Please note the stating I am completely lost does not tell us anything about where you are having problems with this.
Make sure you post the solution as a .java file before the due date.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
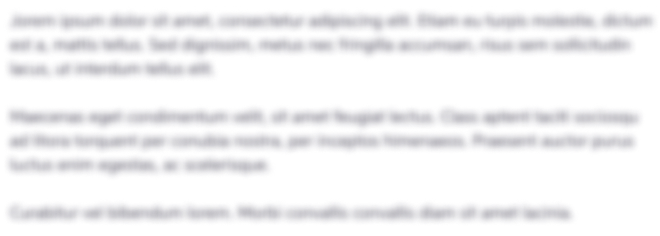
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started