Question
Java help pleaseIm almost there with the code..I just need a little help pushing me over the finish line. Im working on a Java project
Java help pleaseIm almost there with the code..I just need a little help pushing me over the finish line.
Im working on a Java project that asks the user to stock inventory in a store using an ArrayList. In my original program I had initialized an array to 2 (e.g. private int size = 2; //initialized value to 2) for test case purposes. Now I am modifying the program to allow unlimited items to be placed into inventory using the ArrayList.
I have an Inventory class that does NOT need to be modified, and the StoreInventoryMain class which does NOT need to be modified.
The only class that needs to be modified is the Store class, which will use an ArrayList of unknown size. The original program used a For loop to go through the array to add the two inventory items.
In this new version I need to ask the user if they want to continue adding more inventory items and repeat that work if the answer is Y or y.
For this new version I will need to use the get method, (e.g.items.get(i)), and will need to chain other methods to it like the sell method, and the findItem method.
So, when the program runs, it should ask the user for inventory information, and ask if they want to continue to add more inventory, instead of asking for 2 items and no more. Thus, the user can enter any number of items.
Here is my Store class which I know will need to be modified;
import java.util.*;
public class Store {
//instance variables private ArrayList
//method for stocked inventory private void stockThisInventory(Scanner stdIn) { //local variables String name; String choice = "Y"; double price; int qty;
for (int i = 0; i
//method to display and item using a For-Each loop public void display() { for (int i = 0; i
//method to find an item private int findItem(String name) { for (int i = 0; i
//method to sell an item, first find item then call the method //in the Inventory class to sell an item, return price public double sell(String name, int qty) { int index = findItem(name); double price; if (index != -1) { price = items[index].sell(qty); } else { price = -1; }//end for return price; }//end method sell
}//end class Store
Here is my Inventory class which will NOT need to be modified;
public class Inventory {
//instance variables private String name; private double price; private int quantity; //**************************************************************************
//Constructor with 3 parameters for each instance variable public Inventory(String name, double price, int quantity) { this.name = name; this.price = price; this.quantity = quantity; }//end constructor Inventory //**************************************************************************
//methods for name, price, quantity; return each public String getName() { return name; }//end method getName
public double getPrice() { return price; }//end method getPrice
public int getQty() { return quantity; }//end method getQty //**************************************************************************
//method to display item,with name, price, and quantity public String display() { return "Item: " + name + "\t" + "Price: $" + price + "\t" + "Quantity: " + quantity; }//end method display //**************************************************************************
//method to sell with parameter for quantity of item purchased public double sell(int qty) { double pr = 0; if (qty
}//end class Inventory
Here is my StoreInventoryMain class which will NOT need to be modified;
public static void main(String[] args) {
//instance variables String name; int qty; double totPrice; Scanner stdIn = new Scanner(System.in); String choice = "Y";
//instantiate Store variable to call constructor in Store class Store store = new Store(); //call method to display inventory store.display(); //**********************************************************************
//while loop ask user which item to purchase and quantity while (choice.equalsIgnoreCase("y")) { System.out.print("Which item? "); name = stdIn.nextLine(); System.out.print("How many? "); qty = Integer.parseInt(stdIn.nextLine()); //call the sell method in the Store class //which will need the name of the item and quantity totPrice = store.sell(name, qty); if (totPrice == -1) { System.out.println("Can't find this item, sorry "); } else if (totPrice == 0) { System.out.println("We don't have that quantity to sell. "); } else { System.out.printf("Cost: $%.2f ", totPrice); } System.out.println("Inventory Listing"); store.display(); System.out.print("Continue? Y/N "); choice = stdIn.nextLine(); System.out.println(); }//end while }//end class main
}//end class StoreInventoryMain
Here is a sample of the final output;
Thank you so much for any thoughts you are able to provide.
Stock thi inventory: Please add an item to inventory Name of inventory item: stapler Price of that item: 10.79 Quantity of that item: 10 Contine? Y/N Y Name of inventory item: hole punch Price of that item: 8.29 Quantity of that item: 20 Continue? Y/N y Name of inventory item: pen Price of that item: 2.50 Quantity of that item: 100 Continue? Y/N y Name of inventory item: envelope Price of that item:.75 Quantity of that item: 200 Continue? Y/N n Inventory Listing Item: stapler Price: $10.79 Quantity: 10 Item: hole punch Price: $8.29 Quantity: 20 Item: pen Price: $2.5 Quantity: 100 Item: envelope Price: $0.75 Quantity: 200 Item 1 Item 2 Item 3 Item 4Step by Step Solution
There are 3 Steps involved in it
Step: 1
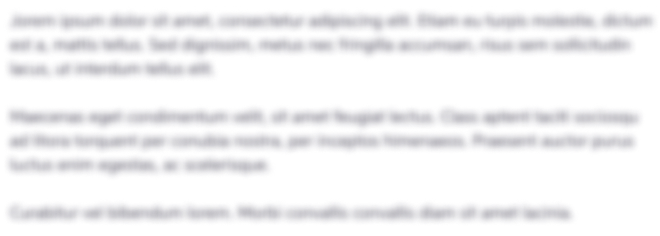
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started