Question
JAVA HELP!!!!!! Requirements Specification and System Design This lab has you create a MyHashMap object for storing the frequency counts for each individual character found
JAVA HELP!!!!!!
Requirements Specification and System Design
This lab has you create a MyHashMap object for storing the frequency counts for each individual character found in a String. The MyHashMap key is a FileChar object holding the character and the MyHashMap value is a HuffElement object holding the character, the character frequency, and the character code. These two classes will be carried over to Project 2.
The book, the slides and given Lesson 7 Source Code provide most of the code needed for this program, including the MyMap interface and the MyHashMap class. You must create two additional classes, one to represent the map key (FileChar) and the other to represent the map value (HuffElement). In addition, you must provide half of the code for the TestMyHashMap driver program.
Most of the code for this driver class will also be used in both the VigHuff driver class (given to you for Project 2) and the Huffman class (which you must supply for Project 2).
The tables below show you the API for the FileChar and HuffElement classes. You must supply the method implementations. The TestMyHashMap class has the main method and a second method that reads in a file that is provided: FDREconomics.txt.
The TestMyHashMap class main method should follow the following algorithm:
Create a MyHashMap object that has FileChar keys and HuffElement values.
Read the FDREconomics.txt file into a String object using the provided readFile method.
Walk through the String object, character-by-character, to record the character frequency in the String object:
For each new different character encountered you must instantiate a new FileChar object and add the character to it. Then you must instantiate a HuffElement object and add the character to it and increment the character count in it. This map entry pair is then added to the HashMap object you created.
For each character previously encountered you must use the FileChar key object for it to retrieve the HuffElement value object and increment the character count in it.
Once you have completely read the whole file, you must retrieve the key set from the hash map and iterate through the key set using a for each loop and for each key, retrieve the character from the FileChar key and the count from the HuffElement value and print these, as follows:
Sample Output:
Char: D Count: 1
Char: E Count: 1
Char: F Count: 2
Char: A Count: 4
Char: d Count: 49
Char: Count: 271
Char: e Count: 149
Char: b Count: 12
Char: c Count: 42
Char: ' Count: 2
Table 1: FileChar API
Data & Methods | Description |
char ch | Character from the file string. |
FileChar () | Constructor: initializes ch to the blank character. |
FileChar (char c) | Constructor: initializes ch to the incoming char c. |
char getChar() | Returns ch. |
void setChar (char c) | Sets ch to the incoming char c. |
int hashCode() | Returns an int version of ch. |
int compareTo (FileChar ch) | Returns -1, 0, or 1: See implementing Comparable Interface |
boolean equals (Object chr) | Compares chr.ch to ch: See overriding equals in book or Google |
String toString() | Return string version of ch |
Table 2: HuffElement API
Data & Methods | Description |
char ch | Character from the file string. |
int chCount | Character frequency in file string |
String code | Binary character code for compression |
HuffElement ()
| Constructor: initializes ch to the blank character chCount to 0 code to the empty string |
HuffElement (char c) | Constructor: initializes ch to the incoming char c. chCount to 0 code to the empty string |
char getChar() | Returns ch. |
void setChar (char c) | Sets ch to the incoming char c. |
int getCount() | Returns chCount. |
void setCount (int c) | Sets chCount to the incoming count c. |
void incCount() | Increments chCount |
String getCode() | Returns code. |
void setCode (String c) | Sets code to the incoming String c. |
int compareTo (HuffElement he) | Returns -1, 0, or 1: Compare the counts. See implementing Comparable Interface |
String toString() | Return string version of the three data members as: Char: y Code: Count: 15 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
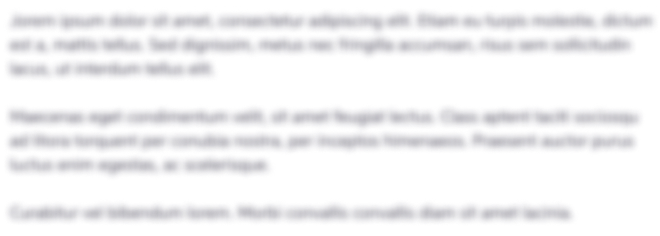
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started