Question
Java help!! trouble with stacks. use pop and push to implement this function: Card[] removeTopCard(Card theCard) throws StackEmptyException { } Write a method called removeTopCards
Java help!!
trouble with stacks. use pop and push to implement this function:
Card[] removeTopCard(Card theCard) throws StackEmptyException {
}
Write a method called removeTopCards which accepts a reference to a Card object
called theCard. This method will allow the user to request that all cards up to and including theCard be removed from the discard pile and returned in an array, thus the return-type of the method is Card[ ]. It is important that you think through the code required to accomplish this BEFORE you begin writing it. Consider the following as you plan:
You can use the equals method in the Card class to compare two cards.
You must use a while loop to find the card in the discard pile (stack).
You should throw a Stack exception if the Card is not found. There are two
reasons theCard may not be found. First, if the discard pile (stack) is empty and second, if the card is not found within the discard pile. You should throw an exception in both cases, but the message stored in the exception object should indicate the type of error that occurred.
If the card is found, remove the appropriate cards from the stack and store their references in an array.
The cards should be in order from theCard to the top of the discard pile, assuming the card has been found.
The array reference should be returned, again assuming the card has been found.
//CARD CLAS::
publicclassCard {
privateintrank;
privateintsuit;
publicCard() {
rank= (int) (Math.random() * 13);
suit= (int) (Math.random() * 4);
}
publicCard(intn) {
if(n>= 0 && n<= 51) {
rank= n% 13;
suit= n/ 13;
}
}
publicCard(intr, ints) {
if((r>= 0 && r<= 12) && (s>= 0 && s<= 3)) {
rank= r;
suit= s;
}
}
publicString toString() {
returngetRankAsString() + getSuitAsString();
}
publicvoidsetRank(intr) {
if(r>= 0 && r<= 12)
rank= r;
}
publicvoidsetSuit(ints) {
if(s>= 0 && s<= 3)
suit= s;
}
publicintgetRank() {
returnrank;
}
publicintgetSuit() {
returnsuit;
}
publicString getRankAsString() {
String[]ranks= { "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A"};
returnranks[rank];
}
publicString getSuitAsString() {
String[] suits= { " Clubs", " Diamonds", " Hearts", " Spades"};
returnsuits[suit];
}
publicbooleanequals(Card otherCard) {
return((rank== otherCard.rank) && (suit== otherCard.suit));
}
publicintcompareByRank(Card otherCard) {
returnrank- otherCard.rank;
}
publicintcompareBySuit(Card otherCard) {
returnsuit- otherCard.suit;
}
}
//STACK
publicclassStack
T[] data;
privateintsize;
privatestaticfinalintMAX_SIZE= 100;
publicStack(){
data= (T[]) newObject[MAX_SIZE];
size= 0;
}
publicStack(intsize){
data= (T[]) newObject[size];
this.size= 0;
}
publicintgetSize(){
returnsize;
}
publicString toString() {
String str= "Stack Size: "+ size+ " -- ";
for(inti= size-1; i>= 0 ; i--) {
str= str+ data[i] + " ";
}
returnstr+ "-- ";
}
publicvoidpush( T x) throwsStackFullException {
if(isFull()) {
thrownewStackFullException("Stack is Full");
}
else
data[size++] = x;
}
publicT pop() throwsStackEmptyException {
if(isEmpty()) {
thrownewStackEmptyException("Stack is Empty");
}
returndata[--size];
}
publicT peek() throwsStackEmptyException {
if(isEmpty()) {
thrownewStackEmptyException("Stack is Empty");
}
returndata[size-1];
}
publicbooleanisEmpty(){
return(size== 0);
}
publicbooleanisFull() {
return(size== data.length);
}
}
//STACK ADT
public interface StackADT
voidpush( T x) throwsStackFullException;
T pop() throwsStackEmptyException;
T peek() throwsStackEmptyException;
booleanisEmpty();
booleanisFull();
intgetSize();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
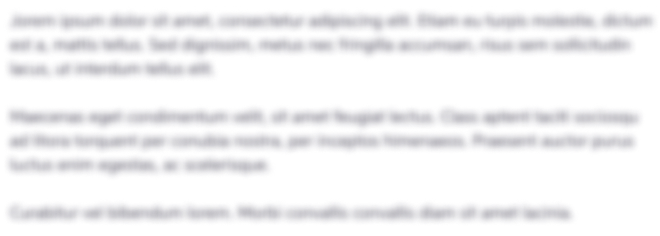
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started