Question
JAVA I am using jGRASP editor, please highlight or bold changes. below are the attachment //******************************************************************** // Equation.java Authors: Lewis/Loftus // // Solution to Programming
JAVA
I am using jGRASP editor, please highlight or bold changes. below are the attachment
//********************************************************************
// Equation.java Authors: Lewis/Loftus
//
// Solution to Programming Project 10.9
//********************************************************************
public class Equation
{
private int a, b, c;
public final String SQUARED = "\u00B2";
//------------------------------------------------------------------
// Sets up the default coeficients of this equation:
// a*x^2 + b*x + c
//------------------------------------------------------------------
public Equation()
{
a = b = c = 0;
}
//------------------------------------------------------------------
// Sets up the coeficients of this equation as specified.
//------------------------------------------------------------------
public Equation (int aValue, int bValue, int cValue)
{
a = aValue;
b = bValue;
c = cValue;
}
//------------------------------------------------------------------
// Computes the current value of this equation.
//------------------------------------------------------------------
public double computeValue(double x)
{
return a*x*x + b*x + c;
}
//------------------------------------------------------------------
// Returns a string representation of this equation.
//------------------------------------------------------------------
public String toString()
{
StringBuffer equation = new StringBuffer();
if (a==0 && b==0 && c==0)
equation.append("0");
else
{
if (a != 0)
{
if (a==-1)
equation.append("-");
else
if (a!=1)
equation.append(a);
equation.append( "x" + SQUARED);
}
if (b != 0)
{
if (b
{
if (a==0)
equation.append("-");
else
equation.append(" - ");
b = -b;
}
else
equation.append(" + ");
if (b!=1 && b!= -1)
equation.append(b);
equation.append("x");
}
if (c != 0)
{
if (c
{
if (a!=0 || b!=0)
equation.append(" - ");
else
equation.append("-");
c = c;
}
else
equation.append(" + ");
equation.append(c);
}
}
// erase leading +
if (equation.length() > 2)
{
char ch = equation.charAt(1);
if (ch=='+')
{
equation.deleteCharAt(0);
equation.deleteCharAt(0);
}
}
return equation.toString();
}
}
==============================================================================================
//********************************************************************
// EquationGraph.java Author: Lewis/Loftus
//
// Solution to Programming Project 10.9
//********************************************************************
import javax.swing.*;
public class EquationGraph
{
//-----------------------------------------------------------------
// Creates and presents the program frame.
//-----------------------------------------------------------------
public static void main(String[] args)
{
JFrame frame = new JFrame("Equation Graph");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(new EquationGraphPanel());
frame.pack();
frame.setVisible(true);
}
}
//********************************************************************
// EquationGraphPanel.java Authors: Lewis/Loftus
//
// Solution to Programming Project 10.9
//********************************************************************
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.*;
import javax.swing.border.LineBorder;
public class EquationGraphPanel extends JPanel
{
private JSlider aSlider;
private JSlider bSlider;
private JSlider cSlider;
private EquationViewportPanel display;
private JLabel equationLabel;
private final String EQUATION_SUBSTRING = "Graph of equation y = ";
private final int MIN_VALUE = -5;
private final int MAX_VALUE = 5;
private final int INIT_VALUE = 0;
private final int TICK_SPACING = 5;
private final int MINOR_TICK_SPACING = 1;
//-----------------------------------------------------------------
// Sets up the panel.
//-----------------------------------------------------------------
public EquationGraphPanel()
{
setLayout(new BorderLayout());
add(getSliderPanel(), BorderLayout.WEST);
display = new EquationViewportPanel();
add(display, BorderLayout.CENTER);
equationLabel = new JLabel(EQUATION_SUBSTRING + 0);
equationLabel.setHorizontalAlignment(SwingConstants.CENTER);
equationLabel.setFont(new Font("Dialog", Font.BOLD, 16));
add(equationLabel, BorderLayout.NORTH);
}
//-----------------------------------------------------------------
// Creates the sliders and slider panel.
//-------------------------------------------------------------
private JPanel getSliderPanel()
{
SliderMouseListener changed = new SliderMouseListener();
aSlider = new JSlider(JSlider.HORIZONTAL, MIN_VALUE, MAX_VALUE, INIT_VALUE);
aSlider.setPaintTicks(true);
aSlider.setPaintLabels(true);
aSlider.setMajorTickSpacing(TICK_SPACING);
aSlider.setMinorTickSpacing(MINOR_TICK_SPACING);
aSlider.setSnapToTicks(true);
aSlider.addMouseListener(changed);
bSlider = new JSlider(JSlider.HORIZONTAL, MIN_VALUE, MAX_VALUE, INIT_VALUE);
bSlider.setPaintTicks(true);
bSlider.setPaintLabels(true);
bSlider.setMajorTickSpacing(TICK_SPACING);
bSlider.setMinorTickSpacing(MINOR_TICK_SPACING);
bSlider.setSnapToTicks(true);
bSlider.addMouseListener(changed);
cSlider = new JSlider(JSlider.HORIZONTAL, MIN_VALUE, MAX_VALUE, INIT_VALUE);
cSlider.setPaintTicks(true);
cSlider.setPaintLabels(true);
cSlider.setMajorTickSpacing(TICK_SPACING);
cSlider.setMinorTickSpacing(MINOR_TICK_SPACING);
cSlider.setSnapToTicks(true);
cSlider.addMouseListener(changed);
JPanel aPanel = new JPanel();
aPanel.setLayout(new BoxLayout(aPanel, BoxLayout.Y_AXIS));
aPanel.setBorder(new LineBorder(Color.black));
aPanel.add(new JLabel("value of 'a'"));
aPanel.add(aSlider);
JPanel bPanel = new JPanel();
bPanel.setLayout(new BoxLayout(bPanel, BoxLayout.Y_AXIS));
bPanel.setBorder(new LineBorder(Color.black));
bPanel.add(new JLabel("value of 'b'"));
bPanel.add(bSlider);
JPanel cPanel = new JPanel();
cPanel.setLayout(new BoxLayout(cPanel, BoxLayout.Y_AXIS));
cPanel.setBorder(new LineBorder(Color.black));
cPanel.add(new JLabel("value of 'c'"));
cPanel.add(cSlider);
JPanel sliderPanel = new JPanel();
sliderPanel.setLayout(new BoxLayout(sliderPanel, BoxLayout.Y_AXIS));
JLabel title = new JLabel("ax\u00B2 + bx + c");
title.setFont(new Font("Dialog", Font.BOLD, 16));
title.setHorizontalAlignment(SwingConstants.CENTER);
sliderPanel.add(title);
sliderPanel.add(new JLabel("Select values for each coefficient:"));
sliderPanel.add(Box.createVerticalGlue());
sliderPanel.add(aPanel);
sliderPanel.add(Box.createVerticalGlue());
sliderPanel.add(bPanel);
sliderPanel.add(Box.createVerticalGlue());
sliderPanel.add(cPanel);
return sliderPanel;
}
//-----------------------------------------------------------------
// Update and equation label.
//-------------------------------------------------------------
private void updateEquationLabel (Equation equation)
{
equationLabel.setText (EQUATION_SUBSTRING + equation.toString());
}
//********************************************************************
// Represents the mouse listener class for the sliders.
//********************************************************************
private class SliderMouseListener extends MouseAdapter
{
//-----------------------------------------------------------------
// Redraws the graph.
//-----------------------------------------------------------------
public void mouseReleased(MouseEvent event)
{
Equation equation = new Equation(aSlider.getValue(),
bSlider.getValue(), cSlider.getValue());
display.setEquation(equation);
updateEquationLabel(equation);
repaint();
}
}
}
//********************************************************************
// EquationViewportPanel.java Authors: Lewis/Loftus
//
// Solution to Programming Project 10.9
//********************************************************************
import java.awt.*;
import javax.swing.JPanel;
import javax.swing.border.LineBorder;
public class EquationViewportPanel extends JPanel
{
private final int X_MIN = -10, Y_MIN = -10, X_MAX = 10, Y_MAX = 10;
private final int REAL_WORLD_WIDTH = X_MAX - X_MIN;
private final int REAL_WORLD_HEIGHT = Y_MAX - Y_MIN;
private final double TICK_LENGTH = 0.2;
private Equation equation;
//-----------------------------------------------------------------
// Constructor: Sets up this panel.
//-----------------------------------------------------------------
public EquationViewportPanel()
{
equation = new Equation();
setBorder(new LineBorder(Color.black, 4));
setPreferredSize(new Dimension(500, 500));
}
//-----------------------------------------------------------------
// Sets the equation.
//-----------------------------------------------------------------
void setEquation(Equation newEquation)
{
equation = newEquation;
}
//-----------------------------------------------------------------
// Converts world X coordinate to screen X coordinate.
//-----------------------------------------------------------------
private int convertX(double x)
{
double offset = x - X_MIN;
double result = offset * getSize().width / REAL_WORLD_WIDTH;
return (int) Math.round(result);
}
//-----------------------------------------------------------------
// Converts world Y coordinate to screen Y coordinate.
//-----------------------------------------------------------------
private int convertY(double y)
{
double offset = Y_MAX - y;
double result = offset * getSize().height / REAL_WORLD_HEIGHT;
return (int) Math.round(result);
}
//-----------------------------------------------------------------
// Draws a line in world coordinates on the screen.
//-----------------------------------------------------------------
private void drawScreenLine(Graphics page, double xMin, double yMin,
double xMax, double yMax)
{
page.drawLine(convertX(xMin), convertY(yMin), convertX(xMax), convertY(yMax));
}
//-----------------------------------------------------------------
// Draws a point in world coordinates on the screen.
//-----------------------------------------------------------------
private void drawScreenPoint(Graphics page, double x, double y)
{
page.drawLine(convertX(x), convertY(y), convertX(x), convertY(y));
}
//-----------------------------------------------------------------
// Draws the graph axes and equation.
//-----------------------------------------------------------------
public void paintComponent(Graphics page)
{
page.setColor(Color.white);
page.fillRect(0,0,getSize().width, getSize().height);
// draw the x and y axis
page.setColor(Color.pink);
drawScreenLine(page, X_MIN, 0, X_MAX, 0);
drawScreenLine(page, 0, Y_MIN, 0, Y_MAX);
// draw tick marks
for (int x=X_MIN; x drawScreenLine(page, x, -TICK_LENGTH, x, TICK_LENGTH); for (int y=Y_MIN; y drawScreenLine(page, -TICK_LENGTH, y, TICK_LENGTH, y); // draw the graph of the equation page.setColor(Color.black); double x = X_MIN; double y; double stepSize = (double)(X_MAX - X_MIN) / getSize().width; int screenX = getSize().width; for (int i = 0; i { y = equation.computeValue(x); drawScreenPoint(page, x, y); x += stepSize; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
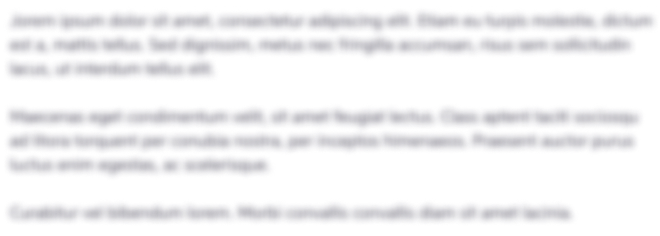
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started