Question
Java: In this assignment we will create a sorting program that provides the user with a large assortment of sorting methods and options. The user
Java:
In this assignment we will create a sorting program that provides the user with a large assortment of sorting methods and options. The user should be able to choose from a menu to select which Sort they would like to use.
For each Sort, be sure to sort four arrays (*see note below), and list the time it took to sort each of the four arrays either in milliseconds or nanoseconds.
For each sorting algorithm, write a brief description of how the sort works, and be sure to note the time complexity. You can include both in the code as comments.
The user should be able to choose from the following sort algorithms (Be sure to implement all). If you use any other resource (including online) you need to cite the source in your code.
Bogo Sort **
Selection Sort
Insertion Sort
Bubble Sort
Quick Sort
Shell Sort
Merge Sort
Gnome Sort
Cocktail Sort
Radix Sort
** One more Sort of your choice**
*Note: For the assignment, you will need to create several arrays of integers of the following sizes {20,100, 500, 1000}. Fill the values of these arrays with random values between the number 0 and 999. You may need to reinitialize these arrays if the user decides to run another sort. You may also need values of 1000 to 9999 if you want to use Radix from the in-class example.
**For Bogo Sort, due to the speed you may want to give the user a choice of the array size so that it may finish the sort in a somewhat timely manner.
I have all the code, but it is not working as it has to according to the instructions: Help me please
package labs4;
import java.util.*;
import java.util.Scanner;
import java.io.*;
public class Sorting {
private static final Random RAND = new Random(42);
public static void main(String args[]){
// Populating all four arrays
int[] len = {20,100, 500, 1000};
int[] arr1 = new int[len[0]];
int[] arr2 = new int[len[1]];
int[] arr3 = new int[len[2]];
int[] arr4 = new int[len[3]];
Random rand = new Random();
for(int i=0;i arr1[i] = rand.nextInt(999); } for(int i=0;i arr2[i] = rand.nextInt(999); } for(int i=0;i arr3[i] = rand.nextInt(999); } for(int i=0;i arr4[i] = rand.nextInt(999); } System.out.println("Enter 1 for Bogo Sort" +" 2 for Selection Sort" +" 3 for Insertion Sort" +" 4 for Bubble Sort" +" 5 for Quick Sort" +" 6 for Shell Sort" + "7 for Merge Sort" +" 8 for Gnome Sort" +" 9 for Cocktail Sort" +" 10 for Radix Sort"); Scanner sc = new Scanner(System.in); int choice = sc.nextInt(); switch(choice){ case 1: // break; case 2: // break; case 3: // break; case 5: quickSort1(arr1); quickSort1(arr2); quickSort1(arr3); quickSort1(arr4); break; // Same way apply for other cases default: System.out.println("Enter correct choice"); } // Printing all the arrays for(int i=0;i System.out.print(arr1[i]+" "); } System.out.println(); for(int i=0;i System.out.print(arr2[i]+" "); } System.out.println(); for(int i=0;i System.out.print(arr3[i]+" "); } System.out.println(); for(int i=0;i System.out.print(arr4[i]+" "); } System.out.println(); } public static void quickSort1(int[] a) { quickSort1(a, 0, a.length - 1); } private static void quickSort1(int[] a, int min, int max) { if (min >= max) { return; } int pivot = a[min]; swap1(a, min, max); int i = min; int j = max - 1; while (i <= j) { while (i <= j && a[i] < pivot) { i++; } while (i <= j && a[j] > pivot) { j--; } if (i <= j) { swap1(a, i, j); i++; j--; } } swap1(a, max, i); quickSort1(a, min, i - 1); quickSort1(a, i + 1, max); } private static final void swap1(int[] a, int i, int j) { if (i != j) { int temp = a[i]; a[i] = a[j]; a[j] = temp; } } { int LENGTH = 1000; int RUNS = 17; for (int i = 0; i < RUNS; i++) { int[] a = createRandomArray(LENGTH); long startTime1 = System.currentTimeMillis(); quickSort(a); long endTime1 = System.currentTimeMillis(); if (!isSorted(a)) { throw new RuntimeException("not sorted afterward: " + Arrays.toString(a)); } System.out.printf("%10d elements => %6d ms ", LENGTH, endTime1 - startTime1); LENGTH *= 2; } } // Quick Sort public static void quickSort(int[] a) { quickSort1(a, 0, a.length - 1); } private static void quickSort(int[] a, int min, int max) { if (min >= max) { return; } int pivot = a[min]; swap1(a, min, max); int i = min; int j = max - 1; while (i <= j) { while (i <= j && a[i] < pivot) { i++; } while (i <= j && a[j] > pivot) { j--; } if (i <= j) { swap1(a, i, j); i++; j--; } } swap1(a, max, i); quickSort1(a, min, i - 1); quickSort1(a, i + 1, max); } //Merge Sort public static void mergeSort(int[] a) { if (a.length >= 2) { int[] left = Arrays.copyOfRange(a, 0, a.length / 2); int[] right = Arrays.copyOfRange(a, a.length / 2, a.length); mergeSort(left); mergeSort(right); int i1 = 0; int i2 = 0; for (int i = 0; i < a.length; i++) { if (i2 >= right.length ||(i1 < left.length && left[i1] < right[i2])) { a[i] = left[i1]; i1++; } else { a[i] = right[i2]; i2++; } } } } //Shell Sort public static void shellSort(int[] a) { for (int gap = a.length / 2; gap >= 1; gap = gap / 2) { for (int i = gap; i < a.length; i++) { int temp = a[i]; int j = i; while (j >= gap && a[j - gap] > temp) { a[j] = a[j - gap]; j -= gap; } a[j] = temp; } } } //Insertion Sort public static void insertionSort(int[] a) { for (int i = 1; i < a.length; i++) { int temp = a[i]; int j = i; while (j >= 1 && a[j - 1] > temp) { a[j] = a[j - 1]; j--; } a[j] = temp; } } //Selection Sort public static void selectionSort(int[] a) { for (int pass = 0; pass < a.length; pass++) { int min = pass; for (int j = pass + 1; j < a.length; j++) { if (a[j] < a[min]) { min = j; } } swap1(a, pass, min); } } //Bubble Sort public static void bubbleSort(int[] a) { for (int pass = 0; pass < a.length; pass++) { boolean changed = false; for (int i = 0; i < a.length - 1 - pass; i++) { if (a[i] > a[i + 1]) { swap1(a, i, i + 1); changed = true; } } if (!changed) { return; } } } //Bogo Sort public static void bogoSort(int[] a) { while (!isSorted(a)) { shuffle(a); } } public static void heapSort(int[] a) { for (int i = a.length / 2; i >= 0; i--) { bubbleDown(a, i, a.length - 1); } for (int i = a.length - 1; i > 0; i--) { swap1(a, 0, i); bubbleDown(a, 0, i - 1); } } private static void bubbleDown(int[] a, int hole, int max) { int temp = a[hole]; while (hole * 2 <= max) { int child = hole * 2; if (child != max && a[child + 1] > a[child]) { child++; } if (a[child] > temp) { a[hole] = a[child]; } else { break; } hole = child; } a[hole] = temp; } //Radix Sort public static void radixSort(int[] a) { Queue for (int i = 0; i < buckets.length; i++) { buckets[i] = new ArrayDeque } int digit = 1; while (toBuckets(a, digit, buckets)) { fromBuckets(a, buckets); digit++; } } private static boolean toBuckets(int[] a, int digit, Queue { boolean nonZero = false; for (int element : a) { int which = kthDigit(element, digit); buckets[which].add(element); if (which != 0) { nonZero = true; } } return nonZero; } private static final int kthDigit(int element, int k) { for (int i = 1; i <= k - 1; i++) { element = element / 10; } return element % 10; } private static void fromBuckets(int[] a, Queue { int i = 0; for (int j = 0; j < buckets.length; j++) { while (!buckets[j].isEmpty()) { a[i] = buckets[j].remove(); i++; } } } //Bucket sort public static void bucketSort(int[] a) { int min = Integer.MAX_VALUE; int max = Integer.MIN_VALUE; for (int k : a) { max = Math.max(max, k); min = Math.min(min, k); } int[] counters = new int[max - min + 1]; for (int k : a) { counters[k - min]++; } int i = 0; for (int j = 0; j < counters.length; j++) { for (int k = 0; k < counters[j]; k++) { a[i] = j + min; i++; } } } /// Stooge Sort public static void stoogeSort(int[] a) { stoogeSort(a, 0, a.length - 1); } private static void stoogeSort(int[] a, int min, int max) { if (min < max) { if (a[min] > a[max]) { swap1(a, min, max); } int oneThird = (max - min + 1) / 3; if (oneThird >= 1) { stoogeSort(a, min, max - oneThird); stoogeSort(a, min + oneThird, max); stoogeSort(a, min, max - oneThird); } } } private static final void swap(int[] a, int i, int j) { if (i != j) { int temp = a[i]; a[i] = a[j]; a[j] = temp; } } private static void shuffle(int[] a) { for (int i = 0; i < a.length; i++) { int randomIndex = (int) (Math.random() * a.length - i); swap1(a, i, i + randomIndex); } } private static boolean isSorted(int[] a) { for (int i = 0; i < a.length - 1; i++) { if (a[i] > a[i + 1]) { return false; } } return true; } public static int[] createRandomArray(int length) { int[] a = new int[length]; for (int i = 0; i < a.length; i++) { a[i] = RAND.nextInt(1000000); } return a; } public static int[] createAscendingArray(int length) { int[] a = new int[length]; for (int i = 0; i < a.length; i++) { a[i] = i; } return a; } public static int[] createDescendingArray(int length) { int[] a = new int[length]; for (int i = 0; i < a.length; i++) { a[i] = a.length - 1 - i; } return a; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
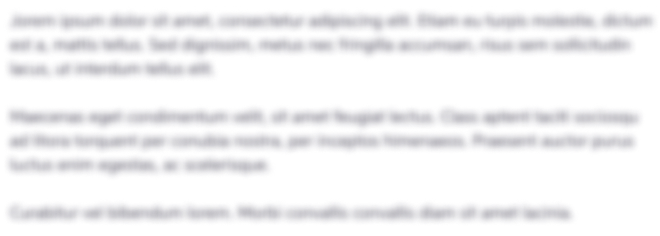
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started