Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java JGrasp Assignment You will modify the EncryptionApplication program that is pasted below these instructions. You will modify the program to throw an Exception when
Java JGrasp Assignment
- You will modify the EncryptionApplication program that is pasted below these instructions.
- You will modify the program to throw an Exception when it encounters a character that is not a - z or a space.
- You will include a try - catch clause that will handle the Exception.
- The code for exception processing should be: display an error dialog.
**EncryptionApplication**
import javafx.application.*; import javafx.event.*; import javafx.geometry.*; import javafx.scene.*; import javafx.scene.control.*; import javafx.scene.layout.*; import javafx.stage.*; public class EncryptionApplication extends Application { public void start(Stage primaryStage) { // Create addressable controls // Reachable from the event handlers TextArea taInput = new TextArea("Input text here"); TextArea taOutput = new TextArea("Output Text:"); // Create the GridPane pane GridPane pane = new GridPane(); pane.setPadding(new Insets(10, 10, 10, 10)); pane.setVgap(5); // Place nodes in the GridPane pane pane.add(new Label("Input Text:"), 0, 0); pane.add(taInput, 0, 1); // Create FlowPane pane FlowPane btnPane = new FlowPane(); btnPane.setAlignment(Pos.CENTER); pane.setHgap(5); // Place nodes in the FlowPane pane and place // pane in the GridPane pane btnPane.setPadding(new Insets(10, 10, 10, 10)); btnPane.setHgap(10); // Create buttons and event handlers // Encrypt Button Button btnEncrypt = new Button("Encrypt"); btnEncrypt.setOnAction(new EventHandler() { public void handle(ActionEvent e) { // Get input field and create EncryptString object EncryptString eObj = new EncryptString(taInput.getText()); // Output the encrypted text taOutput.setText(eObj.encryptString()); } }); // Clear Button Button btnClear = new Button("Clear"); btnClear.setOnAction(new EventHandler () { public void handle(ActionEvent e) { taInput.setText(""); taOutput.setText(""); } }); // Decrypt Button Button btnDecrypt = new Button("Decrypt"); btnDecrypt.setOnAction(new EventHandler () { public void handle(ActionEvent e) { // Get the encrypted string from input field String eString = taInput.getText(); // Output the encrypted text taOutput.setText(EncryptString.decryptString(eString)); } }); // Place Buttons on the FlowPane and place FlowPane on GridPane btnPane.getChildren().addAll(btnEncrypt, btnClear, btnDecrypt); pane.add(btnPane, 0, 2); // Place nodes in the GridPane pane pane.add(new Label("Output Text:"), 0, 3); pane.add(taOutput, 0, 4); //Create scene and place it on the stage Scene scene = new Scene(pane); primaryStage.setTitle("CPT 236 Encryption Application"); primaryStage.setScene(scene); primaryStage.show(); } /** * The main method is only needed for the IDE with limited * JavaFX support. Not needed for running from the command line. */ public static void main(String[] args) { launch(args); } }
EncryptString
public class EncryptString extends AnyString{ // constructor public EncryptString(String str) { super(str); } // constructor public EncryptString(char[] charArray) { super(charArray); } // object/instance encryption method public String encryptString() { String resultString = ""; String lowerString = str.toLowerCase(); for(int i = 0; i < lowerString.length(); i++) { resultString += (char)(lowerString.charAt(i) + 1); } return resultString; } // static/class encryption method public static String encryptString(String str) { String resultString = ""; String lowerString = str.toLowerCase(); for(int i = 0; i < lowerString.length(); i++) { resultString += (char)(lowerString.charAt(i) + 1); } return resultString; } // static/class decryption method public static String decryptString(String str) { String resultString = ""; String lowerString = str.toLowerCase(); for(int i = 0; i < lowerString.length(); i++) { resultString += (char)(lowerString.charAt(i) - 1); } return resultString; } // main method to test class code public static void main(String[] args) { // input a String Scanner input = new Scanner(System.in); System.out.print("Enter a string: "); String in = input.nextLine(); // create an object EncryptString obj = new EncryptString(in); //output object string System.out.println(); System.out.println("***** STRING OBJECT OUTPUT *****"); System.out.println(); System.out.println("String input: " + in); System.out.println("Object string: " + obj.getString()); // output encrypted string String encrypt = obj.encryptString(); System.out.println("Encrypted object method:" + encrypt); // output decrypted string String decrypt = EncryptString.decryptString(encrypt); System.out.println("Decrypted class method:" + decrypt); // output encrypted string encrypt = EncryptString.encryptString(in); System.out.println("Encrypted class method:" + encrypt); // output decrypted string decrypt = EncryptString.decryptString(encrypt); System.out.println("Decrypted class method:" + decrypt); // create an object using character array char[] charArray = {'a', 'b', 'c'}; obj = new EncryptString(charArray); //output object string System.out.println(); System.out.println("***** ARRAY OBJECT OUTPUT *****"); System.out.println(); System.out.println("Object string: " + obj.getString()); // output encrypted string encrypt = obj.encryptString(); System.out.println("Encrypted object method:" + encrypt); // output decrypted string decrypt = EncryptString.decryptString(encrypt); System.out.println("Decrypted class method:" + decrypt); // output encrypted string encrypt = EncryptString.encryptString(in); System.out.println("Encrypted class method:" + encrypt); // output decrypted string decrypt = EncryptString.decryptString(encrypt); System.out.println("Decrypted class method:" + decrypt); } }
**AnyString**
public class AnyString { // declare object variables protected String str; // constructor - initialize object variable public AnyString(String s) { str = s; } // constructor - initialize object variable public AnyString(char[] chArray) { str = new String(chArray); } // return the object variable public String getString() { return str; } // return lowercase version of object varaible public String lowercase() { return str.toLowerCase(); } // return uppercase version of the object variable public String uppercase() { return str.toUpperCase(); } // return the length of the object variable public int getLength() { return str.length(); } // return the object variable public String toString() { return str; } // determine if two AnyString objects are equal public boolean equals(AnyString obj) { return str.equals(obj.getString()); } // determine if object variable is all letters public boolean isLetters() { boolean letters = true; for(int i = 0; i < str.length(); i++) { if(!Character.isLetter(str.charAt(i))) { letters = false; break; } } return letters; } // determine if object variable is all letters public boolean isNumeric() { boolean digits = true; for(int i = 0; i < str.length(); i++) { if(!Character.isDigit(str.charAt(i))) { digits = false; break; } } return digits; } /* // main method to test class code public static void main(String[] args) { // get string from keyboard Scanner input = new Scanner(System.in); System.out.print("Enter a string: "); String in = input.nextLine(); // create object using the string AnyString aString = new AnyString(in); // output the results from each method call System.out.println(); System.out.println("***** OUTPUT - STRING *****"); System.out.println("String input: " + in); System.out.println("String from: " + aString.getString()); System.out.println("String lowercase from: " + aString.lowercase()); System.out.println("String uppercase from: " + aString.uppercase()); System.out.println("String toString: " + aString.toString()); System.out.println("String isLetters: " + aString.isLetters()); System.out.println("String isNumeric: " + aString.isNumeric()); char[] charArray = {'a', 'b', 'c'}; AnyString aString2 = new AnyString(charArray); System.out.println("***** OUTPUT - CHAR ARRAY *****"); System.out.println("String from: " + aString2.getString()); System.out.println("String lowercase from: " + aString2.lowercase()); System.out.println("String uppercase from: " + aString2.uppercase()); System.out.println("String toString: " + aString2.toString()); System.out.println("String isLetters: " + aString2.isLetters()); System.out.println("String isNumeric: " + aString2.isNumeric()); System.out.println("***** TEST EQUALS *****"); System.out.println("Strings equal: " + aString.equals(aString2)); } */ }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
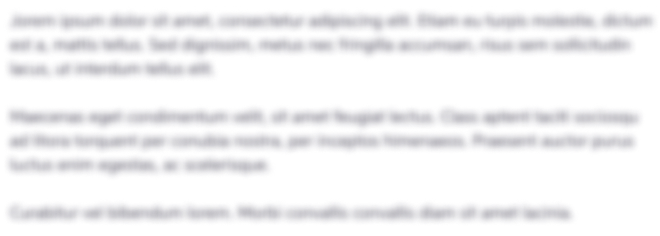
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started