Question
Java lab infix to postfix and evaluate. Need help with error checking for the program and debugging it! My output when using Eclipse it says
Java lab infix to postfix and evaluate. Need help with error checking for the program and debugging it! My output when using Eclipse it says this
"Stack is Empty Cannot Pop"
and it stops from there I have no output or solution file for my csis.txt
Here is the infix file:
8 + 4 * 2 - 6
7 * 2 - 4 * 3 + 2 * 5
2 * 3 * 4 - 8 + 9 / 3 / 3
5 + 7 * 4 - 6
4 * ( 3 + 2 * 4 ) - 7
( 5 + 7 ) / ( 9 - 5 )
3 * ( 5 * ( 5 - 2 ) ) - 9
( ( 5 * ( 4 + 2 ) - ( 8 + 8 ) / 2 ) - 9 ) ^ 3
( ( 5 + 5 * ( 6 - 2 ) + 4 ^ 2 ) * 8 )
( ( ( 3 ^ 4 ) ) )
Here is the java code for each class:
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.*;
import java.util.Scanner;
public class Driver {
public static void main(String[] args) throws IOException {
PrintWriter pw = new PrintWriter(new FileWriter("csis.txt"));
Scanner fileScan = (new Scanner(new File("infix.txt")));
InfixToPostfix infix = new InfixToPostfix();
EvalPostfix eval = new EvalPostfix();
while (fileScan.hasNextLine()) {
String reader = fileScan.nextLine();
String converted = infix.infixToPostfix(reader);
int finalValue = eval.evalPostFix(converted);
System.out.println("Infix: " + reader + " Postfix: " + converted + " Final Value: " + finalValue);
pw.println("Infix: " + reader + "Postfix: " + converted + "Final Value: " + finalValue);
pw.flush();
}
}
}
EvalPostfix Class:
import java.io.*;
import java.util.Scanner;
import static java.lang.Math.*;
public class EvalPostfix {
private int infix;
private ObjectStack valueStack;
private ObjectStack operatorStack;
public EvalPostfix() {
valueStack = new ObjectStack();
operatorStack = new ObjectStack();
}
public int evalPostFix(String converted) {
for (char temp: converted.toCharArray()) {
if (Character.isDigit(temp)) {
int tempInt = Character.getNumericValue(temp);
valueStack.push(tempInt);
}
else if (temp == '+') {
int op1 = (Integer) (valueStack.pop());
int op2 = (Integer) valueStack.pop();
int newVal = op2 + op1;
valueStack.push(newVal);
}
else if (temp == '*') {
int op1 = (Integer) valueStack.pop();
int op2 = (Integer) valueStack.pop();
int newVal = op2 * op1;
valueStack.push(newVal);
}
else if (temp == '/') {
int op1 = (Integer) valueStack.pop();
int op2 = (Integer) valueStack.pop();
int newVal = op2 / op1;
valueStack.push(newVal);
}
else if (temp == '-') {
int op1 = (Integer) valueStack.pop();
int op2 = (Integer) valueStack.pop();
int newVal = op2 - op1;
valueStack.push(newVal);
}
else if (temp == '^') {
int op1 = (Integer) valueStack.pop();
int op2 = (Integer) valueStack.pop();
int newVal = (int) Math.pow(op2, op1);
valueStack.push(newVal);
}
}
return (Integer)valueStack.pop();
}
}
Infix to Postfix class:
import java.io.*;
import java.util.Scanner;
import static java.lang.Math.*;
public class InfixToPostfix {
private StringBuffer sb;
private ObjectStack operators;
public InfixToPostfix() {
operators = new ObjectStack();
}
public String infixToPostfix(String reader) {
sb = new StringBuffer("");
for (char temp: reader.toCharArray()) {
InfixToPostfix eval = new InfixToPostfix();
int precedenceTemp = eval.priority(temp);
if (Character.isDigit(temp)) {
sb.append(temp);
} else if (temp == '(') {
operators.push(temp);
} else if (temp == '+' || temp == '-' || temp == '*' || temp == '/' || temp == '^') {
if (operators.isEmpty() || precedenceTemp > eval.priority((Character) operators.top())) {
operators.push(temp);
} else {
while (!operators.isEmpty() && precedenceTemp <= eval.priority((Character) operators.top())
&& (Character) operators.top() != '(') {
sb.append((Character) operators.pop());
}
operators.push(temp);
}
} else if (temp == ')') {
while ((Character) operators.top() != '(') {
sb.append((Character) operators.pop());
}
operators.pop();
}
}
while (!operators.isEmpty()) {
sb.append((Character) operators.pop());
}
return sb.toString();
}
public int priority(char op) {
switch (op) {
case '^':
return 3;
case '*':
case '/':
return 2;
case '+':
case '-':
return 1;
default:
return 0;
}
}
}
ObjectStack class:
public class ObjectStack implements ObjectStackInterface {
private Object[] item;
private int top;
public ObjectStack(){
item = new Object[1];
top = -1;
}
@Override
public boolean isEmpty() {
return top == -1;
}
@Override
public void push(Object o) {
if (isFull()){
resize(2*item.length);
item[top++] = o;
}
}
@Override
public boolean isFull() {
return top == item.length-1;
}
@Override
public void clear() {
item = new Object[1];
top = -1;
}
@Override
public Object pop() {
if (isEmpty() == true){
System.out.println("Stack is Empty cannot pop ");
System.exit(1);
}
Object temp = item[top--];
if(top == item.length/4){
resize(item.length/2);
}
return temp;
}
@Override
public Object top() {
if (isEmpty() == true){
System.out.println("Stack is Empty cannot pop");
}
return item[top];
}
/** Private method to resize stack **/
private void resize(int size){
Object[] temp = new Object[size];
for(int i = 0; i <= top; i++){
temp[i] = item[i];
item = temp;
}
}
}
And Object Stack Interface:
public interface ObjectStackInterface {
public boolean isEmpty();
public boolean isFull();
public void clear();
public void push(Object o);
public Object pop();
public Object top();
}
If you can please help me by showing me in bold changes you made with the code and what class AND also showing me your output text (the csis.txt) with the correct output I will greatly appreciate it! Thanks :)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
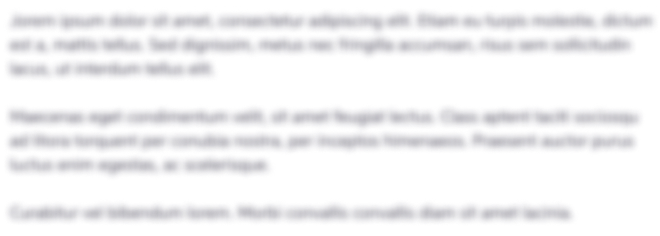
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started