Java language Don't copy others' answers. A new code needed to be provided. Don't use the cities.csv file attached to that assignment. Program Specifications: One
Java language
Don't copy others' answers. A new code needed to be provided.
Don't use the cities.csv file attached to that assignment.
Program Specifications:
One of the most interesting implementations of the dictionary ADT is using a hash table, which, on average, can be very, very fast. Due to the O(1) average insert, remove, and search, hash tables are one of the most popular data structures to store information.
First, you will implement a dictionary using a simple hash function using the sum of the ASCII values of each character in the string key.
This version does NOT need to deal with collisions. In other words, if multiple keys map to the same hash value, your map will NOT allow the additional items to be inserted. The next assignment will implement collision resolution.
Secondly, implement a simple test program that tests all the public methods for the dictionary. Since this implementation of the dictionary will not deal with collisions, you do not need to test for that.
Notes:
The hash function will simply be the sum of each character based on their ASCII values.
For example, "Sudoku" should be inserted into the index 635 since (83+117+100+111+107+117).
ASCII table/values: https://www.ascii-code.com/
The values of each character of the word "Sudoku" are:
S = 83
u = 117
d = 100
o = 111
k = 107
u = 117
To Do:
Design and implement the dictionary/map using a hash table implementation without collision resolution according to the map interface.
Write test code to verify all methods work correctly:
Create at least two map objects and insert at least five key-value pairs into each
Use at least one custom class as a key, and implement the equals method.
Map Interface:/* Based on: java.util.Map interface https://github.com/AdoptOpenJDK/openjdk-jdk11/blob/master/src/java.base/share/classes/java/util/Map.java */ interface Map More formally, if this map contains a mapping from a key * {@code k} to a value {@code v} such that * {@code Objects.equals(key, k)}, * then this method returns {@code v}; otherwise * it returns {@code null}. (There can be at most one such mapping.) * * @param key the key whose associated value is to be returned * @return the value to which the specified key is mapped, or * {@code null} if this map contains no mapping for the key */ V get(Object key); /** * Associates the specified value with the specified key in this map * (optional operation). If the map previously contained a mapping for * the key, the old value is replaced by the specified value. * * @param key key with which the specified value is to be associated * @param value value to be associated with the specified key * @return the previous value associated with {@code key}, or * {@code null} if there was no mapping for {@code key}. * (A {@code null} return can also indicate that the map * previously associated {@code null} with {@code key}, * if the implementation supports {@code null} values.) */ V put(K key, V value); /** * Returns the number of key-value mappings in this map. If the * map contains more than {@code Integer.MAX_VALUE} elements, returns * {@code Integer.MAX_VALUE}. * * @return the number of key-value mappings in this map */ int size(); /** * Returns {@code true} if this map contains no key-value mappings. * * @return {@code true} if this map contains no key-value mappings */ boolean isEmpty(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
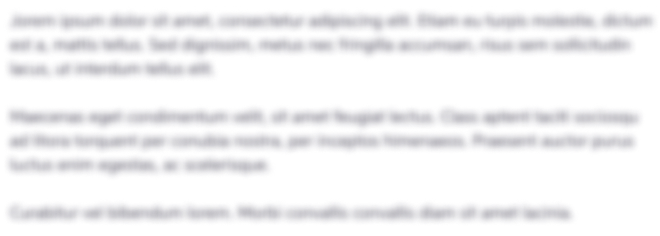
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started