Question
JAVA Language Problem: A hotel wants to develop an application that allows visitors to view their available rooms and easily Reserve one to stay in.
JAVA Language
Problem:
A hotel wants to develop an application that allows visitors to view their available rooms and easily Reserve one to stay in.
You will need to create three classes.
- The first class is your driver for the application and contains the main method. Name this class HotelApp.
- The second class will process reservations for rooms. Name this class RoomReservation.
- The third class is a helper class that stores information about a room. Name this class Room.
Design:
- The classes should be placed in a package with the name
- Your non-application classes RoomReservation and Room should have complete Javadoc comments
HotelApp class
This is the starting point for the application which is the only class to contain a main method.
This driver class will be used to control the user interactions, which include displaying the welcome message, displaying the room inventory, prompting the prospective visitor to select a room from inventory, and finally displaying a receipt. The class should include the following:
- Follow the sample interaction on what to display for prompts, labels, messages and the flow for screen input and outputs.
- Use both the RoomReservation and Room classes
- Three methods:
- A static method, main, that will:
- Initiate the program, manage the flow of execution, and display the Reservation receipt
- A static method, selectRoom, that will:
- Display a welcome message and the room inventory
- Prompt the user to select a room
- A static method, selectExtras, that will:
- Display extra services available
- Prompt the user to select services
- A static method, printReservation, that will:
- displays the reservation receipt
- A static method, main, that will:
RoomReservation class
This class keeps track of the information for each customer Reservation. This class should include the following:
- Named Constants
- Prices
- Breakfast = $9.99
- Crib = $14.50
- Late Checkout = $29.99
- Rates
- sales tax = 6%
- Service fee = 8%
- Prices
- Instance Variables
- 7 instance variables: customer name, reservation date, pretax total, final total, number of visitors, room number, breakfast selected, crib selected, late checkout selected, and extras
- Methods
- Two constructors
- A default constructor that initializes the reservation date to the current date/time
- A constructor that accepts the customer name, room number and room price
- Getters for number of visitors, customer name, room number, room price, breakfast selected, crib selected, late checkout selected, and extras
- Setters for number of visitors, customer name, room number, room, price breakfast selected, crib selected, late checkout selected, and extras
- Two constructors
- setPreTaxTotal:
- extras= breakfast if selected+ crib if selected+ late checkout if selected
- pretax total = room + extras
- setTotalPrice
- reservation sales tax = pretax total * sales tax
- round this value to 2 decimal places prior to storing
- reservation service fee = pretax total * service fee
- round this value to 2 decimal places prior to storing
- final total = pretax total + reservation sales tax + reservation service fee
- reservation sales tax = pretax total * sales tax
- Override the toString method to display the reservation receipt
Room class
This class is helper class and is useful for storing Room information for the room inventory. This class should include the following:
- Instance Variables
- 5 instance variables: room number, capacity, number of beds, number of bathrooms, and price
- Methods
- One constructor that accepts all the instance variables
- Getters for each instance variable
- Setters for price and capacity
- Override the toString method to display the room description in the form of room number, beds, price (e.g. 201, 2 beds, $200.00)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
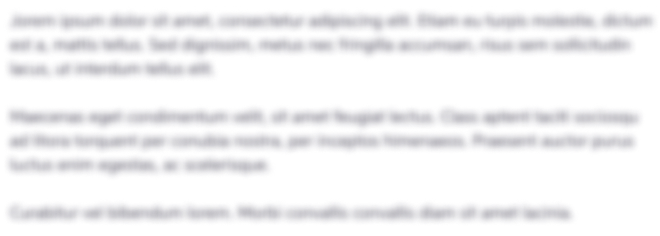
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started