Question
*Java language using eclipse and this assignment is related to the assignment in bottom 1) Add two additional private member variables to the LinkedInUser class:
*Java language using eclipse and this assignment is related to the assignment in bottom
1) Add two additional private member variables to the LinkedInUser class:
// holds the last date/time that this user logged in java.util.Date lastLoginDate;
// holds the number of times this user has logged in int loginCounter;
2) Add and implement the following public methods to the LinkedInUser class:
/** * Increments the number of times this user has logged in and assigns today's * date to the last login date. */
public void incrementLoginCounter() { }
// hint: to assign todays date to a Date type variable, simply create a new // instance of Date. Date today = new Date(); /** * Returns the number of times this user has logged in. * @return the login counter. */
public int getLoginCounter() { }
/** * Returns the Date that this used last logged in. * @return the Date. */
public Date getLastLoginDate() { }
LinkedInCLI Create a class called, LinkedInCLI which will serve as the command line interface between the user and your software. Define the following properties.
/** * Holds the list of users in the system. */
private List users;
/** * Holds the logged in user. */
private LinkedInUser loggedInUser;
public LinkedInCLI() { users = new ArrayList(); }
The LinkedInCLI class should have methods to list all the users (i.e. print out their usernames), sign up a new user, delete a user, print who the logged in user is, sign off, and quit.
1. Create a public static void main method which prints the following menu to the console and awaits user input. Upon entry the application should do the following:
a. Load any previously saved data. See the Serializing and Deserializing section below for the details
b. If there are no users in the system, do the following
i. prompt the user to establish the root user and password.
ii. Default the LinkedInUser type to P for premier and call the newly added incrementLoginCounter() method to establish that this user has logged in once.
iii. Add the newly created user to the list of users defined within the constructor.
iv. Assign the newly created user to loggedInUser property on the class to indicate that this new user is the logged in user.
v.Display the Welcome menu (step 2 below).
c. If there is at least one user in the system, do the following:
i. Prompt the user to login by supplying a user name and password.
ii. If the user name doesnt exist, display the message, There is no user with that user name and re-display the login prompt.
iii. If the user does exist and the password is incorrect, display the message, Password mismatch and re-display the login prompt.
iv. If both username and password are valid:
1. Call the newly added incrementLoginCounter() to increment the number of times this user has logged in.
2. Assign the user to loggedInUser property on the class to indicate that this is the logged in user.
3. display the Welcome menu (step 2 below).
2. Display the Welcome menu.
Welcome
1. List all Users,
2. Sign up a New User,
3. Delete a User,
4. Who am I
5. Sign Off
6. Quit
(substitute the actual logged in user name for the text).
Read the users choice and call the appropriate method on the LinkedInCLI object. This should continue until the user chooses to Quit. (See the below sections for the details on each menu choice)
Serializing and De-serializing
We want the data entered within our LinkedIn CLI to persist between program executions. To do this, serialize your list of LinkedInUser objects before the program terminates (see the Quit section below for the details on this) AND after each action that modifies the data. When the program starts up again, de-serialize the list of LinkedInUser objects rather than making the user always create new ones.
Remember: to serialize and de-serialize the LinkedInUser class, it (along with its super class) must implement Serializable.
Menu Options:
List all Users:
Loop through all the LinkedIn users and print their user name to the console.
Sign up a New User:
Prompt the user for the user name to add. Check if the supplied user name already exists in the users list. If it does, display an error message to the console and re-display the menu.
If the supplied user name is unique, do the following
A) prompt the user to enter a password and what type of user he/she is
* Valid types are S for Standard and P for Premier. If the user enters any other value, display the message, Invalid user type. Valid types are P or S and redisplay the menu.
B)construct a LinkedInUser instance and add the instance to the users list on the LinkedInCLI class.
C) Serialize your list of LinkedInUser objects to save the newly added user.
D) Afterwards, re-display the menu.
Delete a User:
Prompt for the user name to delete. Check if the supplied user name exists in the user list.
If the user does NOT exist, display an error message to the console and re-display the menu.
If the user does exist, prompt for the password of the user being deleted.
If the password is not correct, display an error message to the console and re-display the menu.
If the password is correct, remove the user from the users list, serialize your list of LinkedInUser objects to save the new list, and re-display the menu.
If the deleted user was the logged in user, null out the loggedInUser property on the LinkedInCLI class and re-present the user interface.
// Since the logged in user is now null, the application should act the same as the application did upon start up (either making the user establish a root user or login with an existing user). See step 1 b.
Who am I:
Display the following logged in user information
The user name
The user type
The last logged in date/time. Use the java.text.SimpleDateFormat class to format the date/time to be printable.
The login count
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); String lastLoggedInDate = formatter.format(loggedInUser.getLastLoginDate()); System.out.println("Last Logged In: " + lastLoggedInDate)
Sign off:
Null out the loggedInUser property and re-present the user interface.
Since the logged in user is now null, the application should act the same as the application does upon start up (either making the user establish a root user or login with an existing user). See step 1 b.
Quit:
private static final String PATH = System.getProperty("user.home") + File.separator + "sinclair" + File.separator;
private static final String FILE_NAME = "LinkedInUsers.dat";
Place the above static final fields in your LinkedInCLI class.
Using the above static final fields, serialize the users list to the PATH + FILE_NAME location.
1- new FileOutputStream(PATH + FILE_NAME);
2- The PATH constant will point to your computers home directory.
3-The File.separator constant will contain the directory separator character for your operating system. / for linux or macs \ for windows
If any of the folders in the PATH do not exist, create them.
new File(PATH).mkdirs();
If an existing LinkedInUsers.dat file already exists, delete it and recreate within this save process.
Write Goodbye to the system.out console.
How You Are Graded
1. Your LinkedInCLI implementation prompts the user to establish a root user and password if no users exist, behaving as specified on this assignment (1 point)
2. Your LinkedInCLI implementation prompts the user to login if at least one user exists and properly authenticates the entered user/password (1 point)
3. Upon initial create of the root user/password or proper authentication through the login process has occurred, the Welcome menu is properly displayed as specified in this assignment (1 point)
4. The application properly serializes and deserializes the entered data (3 points)
5. The application properly signs up a new user as specified in this assignment (1 point)
6. The application properly deletes a user as specified in this assignment (1 point)
7. The Who Am I is properly displayed as specified in this assignment (1 point)
8. The user can sign off as specified in this assignment (1 point) Notes
1. Since a big part of this assignment involves user interaction, we will not be writing JUnit tests. 2. If your program does not compile, you will receive a score of 0 on the entire assignment 3. If your program compiles but does not run, you will receive a score of 0 on the entire assignment 4. If your Eclipse project is not exported and uploaded to the eLearn drop box correctly, you will receive a score of 0 on the entire assignment 5. If you do not submit code that solves the problem for this assignment, you will not receive any points for the programs compiling or the programs running.
********/////****The last assignment answer was like this and this assignment is connected to it*****///************
//filename//UserAccount.java
package edu.sinclair;
abstract class UserAccount {
// User Account Data Fields
private String username;
private String password;
// User Account Constructor
public UserAccount(String username, String password) { this.username = username; this.password = password; }
// Generated Getters and Setters for the Data Fields
public String getUsername() { return username; }
public boolean isPasswordCorrect(String password) { return this.password == null ? false : this.password.equals(password); } @Override
public int hashCode() { final int prime = 31; int result = 1; result = prime * result + ((username == null) ? 0 : username.hashCode()); return result; }
@Override
public boolean equals(Object o) { if (this == o) return true; if (o == null) return false; if (getClass() != o.getClass()) return false; UserAccount other = (UserAccount) o; if (username == null) { if (other.username != null) return false; } else if (!username.equals(other.username)) return false; return true; } @Override public String toString() { String str = ""; str += "User Name: " + username; return str; } public abstract void setType(String type); }
//file name//LinkedInUser.java
package edu.sinclair;
import java.util.ArrayList; import java.util.Iterator; import java.util.List;
class LinkedInUser extends UserAccount implements Comparable {
private String type;
private List connections = new ArrayList();
public LinkedInUser(String username, String password) { super(username, password);
public String getType() { return this.type; }
@Override
public void setType(String type) { this.type = type; }
public void addConnection(LinkedInUser user) throws LinkedInException {
for(LinkedInUser linkedInUser : this.connections) {
if(linkedInUser.equals(user)) { throw new LinkedInException("You are already connected with this user"); } } this.connections.add(user); }
public void removeConnection(LinkedInUser user) throws LinkedInException {
Iterator connectionsIterator = this.connections.iterator();
while(connectionsIterator.hasNext()) { LinkedInUser linkedInUser = connectionsIterator.next();
if(linkedInUser.equals(user)) {
this.connections.remove(user); return; } }
throw new LinkedInException("You are NOT connected with this user"); }
public List getConnections() {
return new ArrayList(this.connections); }
@Override
public int compareTo(LinkedInUser user) { r
eturn this.getUsername().compareToIgnoreCase(user.getUsername()); } }
//fillename//LinkedInException.java
package edu.sinclair;
public class LinkedInException extends Exception {
private static final long serialVersionUID = 1L;
LinkedInException() { super(); } LinkedInException(String message) { super(message); }
LinkedInException(String message, Throwable cause) { super(message, cause); }
LinkedInException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) { super(message, cause, enableSuppression, writableStackTrace); }
LinkedInException(Throwable cause) { super(cause); } }
//filename//LinkedInUserTest.java (JUnit Test)
package edu.sinclair;
import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.Collections; import java.util.List; import org.junit.Rule; import org.junit.Test; import org.junit.rules.ExpectedException;
public class LinkedInUserTest {
@Rule
public ExpectedException exceptionRule = ExpectedException.none();
@Test
public void addRetrieveAndDeleteTest() throws LinkedInException { LinkedInUser han = new LinkedInUser("han", "hanPwd"); LinkedInUser luke = new LinkedInUser("luke", "lukePwd"); LinkedInUser leia = new LinkedInUser("leia", "leiaPwd"); han.addConnection(luke); han.addConnection(leia); assertEquals(han.getConnections().size(), 2); assertTrue(han.getConnections().get(0).equals(luke)); assertTrue(han.getConnections().get(1).equals(leia)); han.removeConnection(leia); assertEquals(han.getConnections().size(), 1); assertTrue(han.getConnections().get(0).equals(luke)); }
@Test public void linkedInUserTypeTest() { LinkedInUser linkedInUser = new LinkedInUser("user", "password"); linkedInUser.setType("P"); assertTrue(linkedInUser.getType().equals("P")); }
@Test public void errorCheckAddingAConnectionTest() throws LinkedInException { LinkedInUser han = new LinkedInUser("han", "hanPwd"); LinkedInUser luke = new LinkedInUser("luke", "lukePwd"); han.addConnection(luke); exceptionRule.expect(LinkedInException.class); exceptionRule.expectMessage("You are already connected with this user"); han.addConnection(luke); }
@Test public void errorCheckRemovingAConnectionTest() throws LinkedInException { LinkedInUser han = new LinkedInUser("han", "hanPwd"); LinkedInUser luke = new LinkedInUser("luke", "lukePwd"); LinkedInUser leia = new LinkedInUser("leia", "leiaPwd"); han.addConnection(luke); exceptionRule.expect(LinkedInException.class); exceptionRule.expectMessage("You are NOT connected with this user"); han.removeConnection(leia); }
@Test public void sortingTest() throws LinkedInException { LinkedInUser Han = new LinkedInUser("Han", "hanPwd"); LinkedInUser LUKE = new LinkedInUser("LUKE", "LUKEPWD"); LinkedInUser leia = new LinkedInUser("leia", "leiaPwd"); Han.addConnection(LUKE); Han.addConnection(leia); List hansConnections = Han.getConnections(); Collections.sort(hansConnections); hansConnections.get(0).equals(leia); hansConnections.get(1).equals(LUKE); } }
There are no users in the system. Please establish a root user and password user name: doug password: doug Welcome doug 1. List all Users 2. Sign up a New User 3. Delete a User 4. Who am I 5. Sign off 6. Quit Please login to continue. User name doug Please supply the users password doug Enter a username gwen Enter a password gwen Enter the type of user (P or s) Invalid user type. Valid types are P or s Welcome doug 1. List all Users 2. Sign up a New User 3. Delete a User 4. Who am I 5. Sign off 6. Quit User name: doug Type: P Last Logged In: 2019-01-27 15:04:05 Login Count: 5 There are no users in the system. Please establish a root user and password user name: doug password: doug Welcome doug 1. List all Users 2. Sign up a New User 3. Delete a User 4. Who am I 5. Sign off 6. Quit Please login to continue. User name doug Please supply the users password doug Enter a username gwen Enter a password gwen Enter the type of user (P or s) Invalid user type. Valid types are P or s Welcome doug 1. List all Users 2. Sign up a New User 3. Delete a User 4. Who am I 5. Sign off 6. Quit User name: doug Type: P Last Logged In: 2019-01-27 15:04:05 Login Count: 5Step by Step Solution
There are 3 Steps involved in it
Step: 1
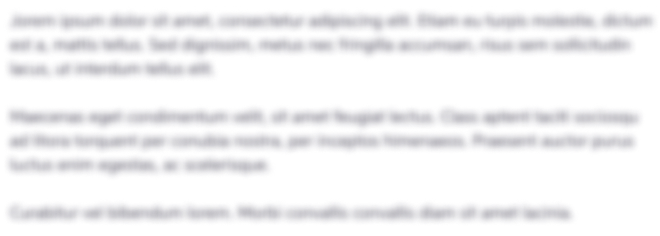
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started