Question
Java Logical statements if if ... else nested if switch let the user enter any digit from 1 to 10 and let the program generate
Java Logical statements if if ... else nested if
switch
let the user enter any digit from 1 to 10 and let the program generate a random number between 1 to 10. if the user hits the lucky number print Congratulations you won if not print Sorry, you lost
public static void main(String[] args) { // TODO Auto-generated method stub Scanner sc = new Scanner(System.in); System.out.println("Please enter the your lucky number between 1 to 10 :"); int number = sc.nextInt(); Random rand = new Random(); int luckyNumber = rand.nextInt(10)+1; System.out.println(luckyNumber); if (number == luckyNumber) System.out.println("Congratulations! you WON"); else System.out.println("Hardluck sorry you lost"); }
Let the user enters an employee salary and the rank of the employee which is 1, 2, 3, or 4 Then upon the rank we will add bonus to the salary as following: Rank Bonus 1 20% 2 15% 3 10% 4 5% and then find the total salary
Using if: public static void main(String[] args) { // TODO Auto-generated method stub Scanner kb = new Scanner(System.in); System.out.println("Enter the salary:"); double salary = kb.nextDouble(); System.out.println("Enter the rank :"); int rank = kb.nextInt(); double bonus = 0; if (rank == 1) bonus = 0.2; else if (rank == 2) bonus = 0.15; else if (rank == 3) bonus = 0.1; else if (rank == 4) bonus = 0.05; else System.out.println("Sorry this is a wrong rank"); double totalSalary = salary + salary * bonus; System.out.println("The total salary = "+totalSalary); }
Using switch
public static void main(String[] args) { // TODO Auto-generated method stub Scanner kb = new Scanner(System.in); System.out.println("Enter the salary:"); double salary = kb.nextDouble(); System.out.println("Enter the rank :"); int rank = kb.nextInt(); double bonus = 0; switch (rank) { case 1: bonus = 0.2; break; case 2: bonus = 0.15; break; case 3: bonus = 0.1; break; case 4: bonus = 0.05; break; default: System.out.println("Sorry this is a wrong rank!"); } double totalSalary = salary + salary * bonus; System.out.println("The total salary ="+totalSalary); }
Suppose we have like a hotel system let the user enter how many nights spend in the hotel and enter the room class of the room as there are 5 classes 1,2,3,4,5 and then find the total amount the guest has to pay
room class price of room per night 1 500 2 420 3 390 or 400 4 360 5 300
but for room class 3 we have different prices upon number of nights its 390 if nights more than 3 nights and 400 if nights are less than or equal 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
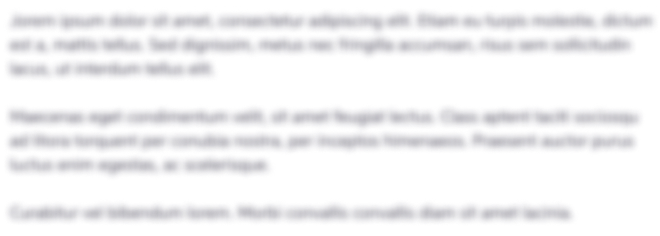
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started