Question
Java: modify the below code so that it also shows the total cost. It currently shows path only. import java.util.PriorityQueue; import java.util.HashSet; import java.util.Set; import
Java: modify the below code so that it also shows the total cost. It currently shows path only.
import java.util.PriorityQueue;
import java.util.HashSet;
import java.util.Set;
import java.util.Collections;
import java.util.List;
import java.util.ArrayList;
import java.util.Comparator;
//Uniform Cost Search
public class UniformCostSearchAlgo{
public static void main(String[] args){
//
Node n1 = new Node("1");
Node n2 = new Node("2");
Node n3 = new Node("3");
Node n4 = new Node("4");
Node n5 = new Node("5");
Node n6 = new Node("6");
Node n7 = new Node("7");
Node n8 = new Node("8");
Node n9 = new Node("9");
Node n10 = new Node("10");
Node n11 = new Node("11");
Node n12 = new Node("12");
Node n13 = new Node("13");
Node n14 = new Node("14");
//Paths
//1
n1.adjacencies = new Path[]{
new Path(n2,75),
new Path(n4,140),
new Path(n8,118)
};
//2
n2.adjacencies = new Path[]{
new Path(n1,75),
new Path(n3,71)
};
//3
n3.adjacencies = new Path[]{
new Path(n2,71),
new Path(n4,151)
};
//4
n4.adjacencies = new Path[]{
new Path(n1,140),
new Path(n5,99),
new Path(n3,151),
new Path(n6,80),
};
//5
n5.adjacencies = new Path[]{
new Path(n4,99),
//178
new Path(n13,211)
};
//6
n6.adjacencies = new Path[]{
new Path(n4,80),
new Path(n7,97),
new Path(n12,146)
};
//7
n7.adjacencies = new Path[]{
new Path(n6,97),
new Path(n13,101),
new Path(n12,138)
};
//8
n8.adjacencies = new Path[]{
new Path(n1,118),
new Path(n9,111)
};
//9
n9.adjacencies = new Path[]{
new Path(n8,111),
new Path(n10,70)
};
//10
n10.adjacencies = new Path[]{
new Path(n9,70),
new Path(n11,75)
};
//11
n11.adjacencies = new Path[]{
new Path(n10,75),
new Path(n12,120)
};
//12
n12.adjacencies = new Path[]{
new Path(n11,120),
new Path(n6,146),
new Path(n7,138)
};
//13
n13.adjacencies = new Path[]{
new Path(n7,101),
new Path(n14,90),
new Path(n5,211)
};
//14
n14.adjacencies = new Path[]{
new Path(n13,90)
};
UniformCostSearch(n1,n13);
List path = printPath(n13);
System.out.println("Path: " + path);
}
public static void UniformCostSearch(Node source, Node goal){
source.pathCost = 0;
PriorityQueue queue = new PriorityQueue(20,
new Comparator(){
//Overrides compare method
public int compare(Node i, Node j){
if(i.pathCost > j.pathCost){
return 1;
}
else if (i.pathCost < j.pathCost){
return -1;
}
else{
return 0;
}
}
}
);
queue.add(source);
Set explored = new HashSet();
boolean found = false;
//While frontier isn't empty
do{
Node current = queue.poll();
explored.add(current);
//Ends if the path is found
if(current.value.equals(goal.value)){
found = true;
}
for(Path e: current.adjacencies){
Node child = e.target;
double cost = e.cost;
//Adds node to queue if the node hasn't been explored
if(!explored.contains(child) && !queue.contains(child)){
child.pathCost = current.pathCost + cost;
child.parent = current;
queue.add(child);
}
//Current path is shorter than the previous path found
else if((queue.contains(child))&&(child.pathCost>(current.pathCost+cost))){
child.parent=current;
child.pathCost = current.pathCost + cost;
queue.remove(child);
queue.add(child);
}
}
}while(!queue.isEmpty()&& (found==false));
}
public static List printPath(Node target){
List path = new ArrayList();
for(Node node = target; node!=null; node = node.parent){
path.add(node);
}
Collections.reverse(path);
return path;
}
}
class Node{
public final String value;
public double pathCost;
public Path[] adjacencies;
public Node parent;
public Node(String val){
value = val;
}
public String toString(){
return value;
}
}
class Path{
public final double cost;
public final Node target;
public Path(Node targetNode, double costVal){
cost = costVal;
target = targetNode;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
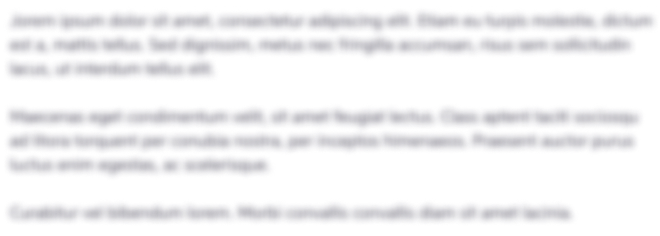
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started