Question
java, must pass all test please, all info is here. Must create GameCharacter.java, ShieldMaiden.java, and Dragon.java, main is provided below You are provided with Main.java
java, must pass all test please, all info is here. Must create GameCharacter.java, ShieldMaiden.java, and Dragon.java, main is provided below
You are provided with Main.java that is a client for this program.
You will create THREE files-- GameCharacter.java, ShieldMaiden.java, and Dragon.java
First: You must Create an interface called GameCharacter WITH JUST PROTOTYPES, NO IMPLEMENTATIONS
A GameCharacter has a few functions associated with it
1) takeHit: decreases the character's health. It should return an int representing damage taken (hit points)
2) heal: increases the character's health. Should return an int representing amount healed (i.e. hit points)
3) getHealth: returns the total current health the character has (i.e. hit points)
4) isAlive: determines if the character is dead. Should return true if the character is alive, and false if it is dead.
Second: Then create two classes. One should be named Dragon, and the other should be named ShieldMaiden (i.e. warrior) that IMPLEMENT Game Character. Give them each private variables for health. The constructor should take in an initial amount to set health to.
Third: Implement the interface functions for these two classes (Dragon and ShieldMaiden). To make the game more interesting, the dragon's takeHit should hurt the dragon a random number from 10 to 20 inclusive and the ShieldMaiden's takeHit should hurt the shieldMaiden a random number from 15 to 25 inclusive. Furthermore, the dragon's heal should only heal the dragon a random number from 1 to 10 inclusive but the ShieldMaiden's heal should heal the ShieldMaiden a random number from 8 to 20 inclusive. A character is dead when they get negative health.
Fourth: There are unit tests that check for all of these requirements. Try your best to pass all the test cases!
A sample output of this game is below (note all the writing is supplied by Main.java)
Jenny the Dragon and Joan Of Arc meet on a dusty mountaintop overlooking a village ** ** Joans Hit Points: 100 Dragon's Hit Points: 100 ** Dragon Attacks! Joan takes: 23 Damage! Joan Heals! 16 health restored ** Joans Hit Points: 93 Dragon's Hit Points: 100 ** Dragon Attacks! Joan takes: 18 Damage! Joan Heals! 13 health restored ** Joans Hit Points: 88 Dragon's Hit Points: 100 ** Joan Attacks! Dragon takes: 16 Damage! Dragon Heals! 9 health restored ** Joans Hit Points: 88 Dragon's Hit Points: 93 ** Joan Attacks! Dragon takes: 15 Damage! Dragon Heals! 6 health restored ** Joans Hit Points: 88 Dragon's Hit Points: 84 ** Dragon Attacks! Joan takes: 18 Damage! Joan Heals! 20 health restored ** Joans Hit Points: 90 Dragon's Hit Points: 84 ** Dragon Attacks! Joan takes: 20 Damage! Joan Heals! 14 health restored ** Joans Hit Points: 84 Dragon's Hit Points: 84 ** Joan Attacks! Dragon takes: 17 Damage! Dragon Heals! 7 health restored ** Joans Hit Points: 84 Dragon's Hit Points: 74 ** Dragon Attacks! Joan takes: 18 Damage! Joan Heals! 16 health restored ** Joans Hit Points: 82 Dragon's Hit Points: 74 ** Joan Attacks! Dragon takes: 17 Damage! Dragon Heals! 10 health restored ** Joans Hit Points: 82 Dragon's Hit Points: 67 ** Joan Attacks! Dragon takes: 19 Damage! Dragon Heals! 2 health restored ** Joans Hit Points: 82 Dragon's Hit Points: 50 ** Joan Attacks! Dragon takes: 14 Damage! Dragon Heals! 8 health restored ** Joans Hit Points: 82 Dragon's Hit Points: 44 ** Dragon Attacks! Joan takes: 18 Damage! Joan Heals! 13 health restored ** Joans Hit Points: 77 Dragon's Hit Points: 44 ** Dragon Attacks! Joan takes: 15 Damage! Joan Heals! 17 health restored ** Joans Hit Points: 79 Dragon's Hit Points: 44 ** Dragon Attacks! Joan takes: 19 Damage! Joan Heals! 13 health restored ** Joans Hit Points: 73 Dragon's Hit Points: 44 ** Joan Attacks! Dragon takes: 11 Damage! Dragon Heals! 10 health restored ** Joans Hit Points: 73 Dragon's Hit Points: 43 ** Joan Attacks! Dragon takes: 10 Damage! Dragon Heals! 1 health restored ** Joans Hit Points: 73 Dragon's Hit Points: 34 ** Joan Attacks! Dragon takes: 13 Damage! Dragon Heals! 10 health restored ** Joans Hit Points: 73 Dragon's Hit Points: 31 ** Joan Attacks! Dragon takes: 18 Damage! Dragon Heals! 3 health restored ** Joans Hit Points: 73 Dragon's Hit Points: 16 ** Dragon Attacks! Joan takes: 18 Damage! Joan Heals! 19 health restored ** Joans Hit Points: 74 Dragon's Hit Points: 16 ** Dragon Attacks! Joan takes: 22 Damage! Joan Heals! 14 health restored ** Joans Hit Points: 66 Dragon's Hit Points: 16 ** Dragon Attacks! Joan takes: 17 Damage! Joan Heals! 20 health restored ** Joans Hit Points: 69 Dragon's Hit Points: 16 ** Joan Attacks! Dragon takes: 13 Damage! Dragon Heals! 7 health restored ** Joans Hit Points: 69 Dragon's Hit Points: 10 Joan Attacks! Dragon takes: 14 Damage! Dragon Heals! 3 health restored The dust settles on this valiant fight and...Joan is left standing in glory
import java.util.Random;
import org.junit.*;
import org.junit.runner.*;
import static org.junit.Assert.*;
public class Main {
public static void main(String[] args) {
//*** UNCOMMENT THIS AFTER YOU CREATE YOUR 3 FILES
Random rand = new Random();
GameCharacter joanOfArc;
GameCharacter jennyTheDragon;
joanOfArc= new ShieldMaiden(100);
jennyTheDragon= new Dragon (100);
System.out.println("**Jenny the Dragon and Joan Of Arc meet on a dusty mountaintop overlooking a village **");
while(joanOfArc.isAlive() && jennyTheDragon.isAlive())
{
System.out.println("** Joans Hit Points: "+joanOfArc.getHealth()+" Dragon's Hit Points: "+jennyTheDragon.getHealth()+" **");
int n = rand.nextInt(2) + 1;
if(n==1)
{
System.out.println("Joan Attacks! Dragon takes: "+jennyTheDragon.takeHit()+" Damage!");
System.out.println("Dragon Heals! "+ jennyTheDragon.heal() + " health restored");
}
else
{
System.out.println("Dragon Attacks! Joan takes: "+ joanOfArc.takeHit()+ " Damage!");
System.out.println("Joan Heals! "+ joanOfArc.heal()+" health restored");
}
}
if(joanOfArc.isAlive())
{
System.out.println("The dust settles on this valiant fight and...Joan is left standing in glory ");
}
else
{
System.out.println("The dust settles on this valiant fight and...The Dragon flies off victories ");
}
//*******UNCOMMENT THE FOLLOWING LINE AND THE TEST CASES BELOW WHEN YOU ARE READY TO TEST******//
//org.junit.runner.JUnitCore.main("Main");
}
//***UNCOMMENT THESE TESTS WHEN YOU ARE READY TO TEST!****//
/*
@Test
public void checkDragonHeal() {
// Failure message:
// Dragon Heal failed
int jennyOldHealth= jennyTheDragon.getHealth();
jennyTheDragon.heal();
assertTrue("Dragon heal failed!", jennyTheDragon.getHealth()>jennyOldHealth);
}
@Test
public void checkShieldTakeHit() {
// Failure message:
// ShieldMaiden takeHit function failed
int joanOldHealth= joanOfArc.getHealth();
joanOfArc.takeHit();
assertTrue("ShieldMaiden takeHit function failed!", joanOfArc.getHealth()
}
@Test
public void checkDragonTakeHit() {
// Failure message:
// Dragon takeHit function failed
int jennyOldHealth= jennyTheDragon.getHealth();
jennyTheDragon.takeHit();
assertTrue("Dragon takeHit failed!", jennyTheDragon.getHealth()
}
@Test
public void checkShieldHeal() {
// Failure message:
// ShieldMaiden Heal function failed
int joanOldHealth= joanOfArc.getHealth();
joanOfArc.heal();
assertTrue("ShieldMaiden heal function failed!", joanOfArc.getHealth()>joanOldHealth);
}
@Test
public void checkShieldConstructor() {
// Failure message:
// ShieldMaiden constructor or getHealth failed!
assertEquals("ShieldMaiden getHealth or constructor failed!",joanOfArc.getHealth(), 100);
}
@Test
public void testDragonDeath() {
// Failure message:
// Dragon isAlive function failed when Dragon dies
for(int i=0; i<200; i++)
{
jennyTheDragon.takeHit();
}
assertTrue("Dragon isAlive failed", !jennyTheDragon.isAlive());
}
@Test
public void testShieldDeath() {
// Failure message:
// ShieldMaiden isALive function failed Maiden dies
for(int i=0; i<200; i++)
{
joanOfArc.takeHit();
}
assertTrue("joanOfArc isAlive failed", !joanOfArc.isAlive());
}
@Test
public void checkDragonConstructor() {
// Failure message:
// Dragon constructor or getHealth function failed
//jennyTheDragon= new Dragon(100);
assertEquals("Dragon getHealth or constructor failed!",jennyTheDragon.getHealth(), 100);
}
@Test
public void ShieldTakeHitRange() {
// Failure message:
// ShieldMaiden takeHit not between 15 to 25 inclusive
for(int i=0; i<100; i++)
{
assertTrue("ShieldMaiden takeHit not between 15 to 25", joanOfArc.takeHit()>14 && joanOfArc.takeHit()<26);
}
}
@Test
public void dragonTakeHitRange() {
// Failure message:
// Dragon Take Hit Not Between 10 and 20 inclusive
for(int i=0; i<100; i++)
{
assertTrue("Dragon Take Hit Failed", jennyTheDragon.takeHit()>9 && jennyTheDragon.takeHit()<21);
}
}
@Test
public void dragonHealRange() {
// Failure message:
// Dragon Heal not between 1 to 10 inclusive
for(int i=0; i<100; i++)
{
assertTrue("Dragon Take Hit Failed", jennyTheDragon.heal()>0 && jennyTheDragon.heal()<11);
}
}
@Test
public void ShieldHealRange() {
// Failure message:
// ShieldMaiden Heal not between 8 to 20 inclusive
for(int i=0; i<100; i++)
{
assertTrue("Dragon Take Hit Failed", joanOfArc.heal()>7 && joanOfArc.heal()<21);
}
}
*/
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
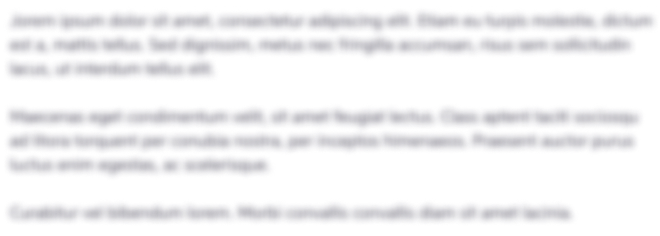
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started