Question
Java only. Please look @ the example before.. thanks! These questions involve choosing the right abstraction (Collection, Set, List, Queue, Deque, SortedSet, Map, or SortedMap)
Java only. Please look @ the example before.. thanks!
These questions involve choosing the right abstraction (Collection, Set, List, Queue, Deque, SortedSet, Map, or SortedMap) to EFFICIENTLY accomplish the task at hand. The best way to do these is to read the question and then think about what type of Collection is best to use to solve it. There are only a few lines of code you need to write to solve each of them.
Unless specified otherwise, sorted order refers to the natural sorted order on Strings, as defined by String.compareTo(s).
DONT MODIFY MAIN
- [5 marks] Read the input one line at a time and output only the last 9999 lines in the order they appear. If there are fewer than 9999 lines, output them all. For full marks, your code should be fast and should never store more than 9999 lines.
package comp2402a1; import java.io.BufferedReader; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class Part1 { /** * @param r the reader to read from * @param w the writer to write to * @throws IOException */ public static void doIt(BufferedReader r, PrintWriter w) throws IOException { //Code goes here } /** * The driver. Open a BufferedReader and a PrintWriter, either from System.in * and System.out or from filenames specified on the command line, then call doIt. * @param args */ public static void main(String[] args) { try { BufferedReader r; PrintWriter w; if (args.length == 0) { r = new BufferedReader(new InputStreamReader(System.in)); w = new PrintWriter(System.out); } else if (args.length == 1) { r = new BufferedReader(new FileReader(args[0])); w = new PrintWriter(System.out); } else { r = new BufferedReader(new FileReader(args[0])); w = new PrintWriter(new FileWriter(args[1])); } long start = System.nanoTime(); doIt(r, w); w.flush(); long stop = System.nanoTime(); System.out.println("Execution time: " + 10e-9 * (stop-start)); } catch (IOException e) { System.err.println(e); System.exit(-1); } } }
EXAMPLE:
package comp2402a1; import java.io.BufferedReader; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class Part0 { /** * Read lines one at a time from r. After reading all lines, output * all lines to w, outputting duplicate lines only once. Note: the order * of the output is unspecified and may have nothing to do with the order * that lines appear in r. * @param r the reader to read from * @param w the writer to write to * @throws IOException */ public static void doIt(BufferedReader r, PrintWriter w) throws IOException { Sets = new HashSet<>(); for (String line = r.readLine(); line != null; line = r.readLine()) { s.add(line); } for (String text : s) { w.println(text); } } /** * The driver. Open a BufferedReader and a PrintWriter, either from System.in * and System.out or from filenames specified on the command line, then call doIt. * @param args */ public static void main(String[] args) { try { BufferedReader r; PrintWriter w; if (args.length == 0) { r = new BufferedReader(new InputStreamReader(System.in)); w = new PrintWriter(System.out); } else if (args.length == 1) { r = new BufferedReader(new FileReader(args[0])); w = new PrintWriter(System.out); } else { r = new BufferedReader(new FileReader(args[0])); w = new PrintWriter(new FileWriter(args[1])); } long start = System.nanoTime(); doIt(r, w); w.flush(); long stop = System.nanoTime(); System.out.println("Execution time: " + 10e-9 * (stop-start)); } catch (IOException e) { System.err.println(e); System.exit(-1); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
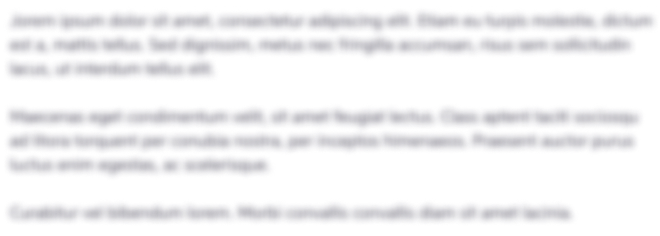
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started