Question
JAVA Part 1 Description Write a constructor for the Course class that takes a String to represent the course subject and an int to represent
JAVA
Part 1 Description
Write a constructor for the Course class that takes a String to represent the course subject and an int to represent the course number. It should ensure that the subject is at most 4 characters long and is all capitalized, and that the number is between 400 and 799 (defaulting to 401 if an invalid number was entered.)
You may find the method Character.toUpperCase helpful in this task.
Part 1 Starter Code
Copy the following start code into a file called Course.java
public class Course{ private String subject; private int number; // YOUR CODE HERE public String toString(){ return subject + " " + number; } } |
Part 2 Description
Write a non-static max method for the Point class, which takes a Point parameter p and returns a new Point object. That new Point object should have an x value which is the maximum of this point's x and p's x, and a y value which is the maximum of this point's y and p's y. It should not change the original Point objects. For example:
Point p1 = new Point( 10, 0 ); Point p2 = new Point( 20, -10 ); p1.max( p2 ); // returns a Point at ( 20.0, 0.00 ) |
Part 2 Starter Code
Copy the following start code into a file called Point.java
public class Point{ private double x; private double y; public Point( double x, double y ){ this.x = x; this.y = y; } // YOUR CODE HERE public String toString(){ return String.format("(%.2f, %.2f)", x, y); } } |
Part 3 Description
Modify the Multiples class so that it implements the Sequence interface (which is given to you already completed). It should have a constructor that takes an integer num and stores it in a field. Its nextVal method should return (as an int) the next multiple of num (num*1, num*2, num*3, etc.) The reset method should cause it to start back at the first multiple. For example:
Multiples m = new Multiples(3); m.nextVal(); // returns 3 m.nextVal(); // returns 6 m.nextVal(); // returns 9 m.reset(); m.nextVal(); // returns 3 m = new Multiples(2); m.nextVal(); // returns 2 m.nextVal(); // returns 4 |
Part 3 Starter Code
Copy the following start code into a file called Multiples.java
public class Multiples { private int num; private int counter; // YOUR CODE HERE } |
Copy the following start code into a file called Sequence.java
public interface Sequence{ public int nextVal(); public void reset(); } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
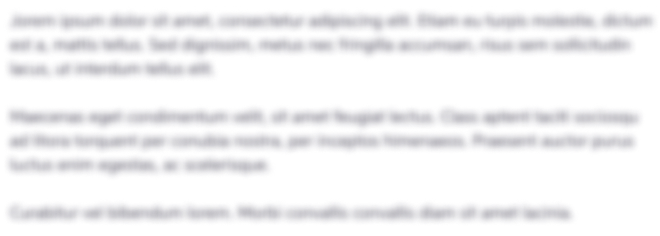
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started