Question
Java passing method help Make a copy of the printAll method called getAll. Instead of printing the genes found, this method should create and return
Java passing method help
Make a copy of the printAll method called getAll. Instead of printing the genes found, this method should create and return a StorageResource containing the genes found. Remember to import the edu.duke libraries otherwise you will get an error message cannot find the class StorageResource.
Here is what I have.
import edu.duke.*;
public class Part1 {
public int findStop(String dnaStr, int startIndex,
String stopCodon){
//Find stop codon from end of ATG
int currIndex=dnaStr.indexOf(stopCodon, startIndex+3);
//as long as curr index not -1
while(currIndex !=-1){
//check for multiple of 3
if((currIndex - startIndex) % 3==0){
//if so, text between is substring
return currIndex;
}
else{
//if not,Update currIndex
currIndex=dnaStr.indexOf(stopCodon,currIndex+1);
}
}
return dnaStr.length();
}
public String findGene(String dna){
//First occurrence of ATG
int startIndex =dna.indexOf("ATG");
//if no ATG is found return null
if(startIndex == -1){
return "";
}
//stop codons to look for in index of dna string
int taaIndex=findStop(dna, startIndex, "TAA");
int tagIndex=findStop(dna, startIndex, "TAG");
int tgaIndex=findStop(dna, startIndex, "TGA");
//store smallest index of ending codon values
int min1;
int min2=0;
if(taaIndex == -1 ||(tgaIndex!=-1 &&
tgaIndex< taaIndex)){
min2 = tgaIndex;
}
else{
min2 = taaIndex;
}
if(min2 ==-1 ||(tagIndex!=-1 && tagIndex < min2)){
min2=tagIndex;
}
//is full dna string none were found so print null
if(min2 == -1){
return "";
}
else{
return dna.substring(startIndex, min2+3);
}
}
public void printGenes(String dna) {
//set start index
int startIndex =0;
//loop
while (true){
//find next gene after start index
String currGene =findGene(dna);
// no gene found leave loop
if(currGene.isEmpty()){
break;
}
//Print gene out
System.out.println(currGene);
//Set start index to 1 past end of last gene
startIndex= dna.indexOf(currGene, startIndex) +
currGene.length();
}
}
public void testfindStop(){
String dna="ACTATGGAAGCATAA";
System.out.println("DNA strand is "+ dna);
int gene=findStop(dna,0,"TAA");
System.out.println("Gene is "+ gene);
dna="ACTATGTAAGCATGATAA";
System.out.println("DNA strand is "+ dna);
gene=findStop(dna,0,"TGA");
System.out.println("Gene is "+ gene);
dna="ACTATGTACCATAACTAG";
System.out.println("DNA strand is "+ dna);
gene=findStop(dna,0,"TAG");
System.out.println("Gene is "+ gene);
}
public void testfindGene(){
String dna = "ATTATGGTCTAGTAA";
System.out.println("DNA strand is "+ dna);
String gene= findGene(dna);
if(gene.isEmpty()){
System.out.println ("");}
else{
System.out.println("Gene is "+ gene);
}
}
public void testprintGenes(String dna){
System.out.println("DNA strand is "+ dna);
printGenes(dna);
}
public void printAll(String dna){
int index=0;
while(true){
int startIndex=dna.indexOf("ATG",index);
if(startIndex!=-1)
System.out.println("start:" + startIndex);
index+=startIndex+3;
if(startIndex ==-1){
System.out.println("");
break;
}
}
}
public StorageResource getAll(String dna){
StorageResource store = new StorageResource();
int index=0;
while(true){
int startIndex=dna.indexOf("ATG",index);
if(startIndex!=-1)
System.out.println("start:" + startIndex);
index += startIndex+3;
if(startIndex ==-1){
System.out.println("");
break;
}
int stopIndex = findStop.(dna,startIndex+3 );
System.out.println("STOP: " + (stopIndex));
// If stop codon is found, save gene as a variable
// and add gene to StorageResource object
if (stopIndex != -1) {
String gene = dna.substring(startIndex, stopIndex + 3);
System.out.println(gene);
store.add(gene);
// Update region to dna to look for codon in,
//setting it to the index right after the stop codon
index = stopIndex + 3;
} else {
// Update region of dna to look for codon in
index = startIndex + 1;
}
}
return store;
}
public void testprintAll(){
String dna = "ATGGAATAGTCGATGGCTTAA";
System.out.println("DNA is "+ dna);
printAll(dna);
}
public void testgetAll(){
String dna = "ATGGAATAGTCGATGGCTTAA";
System.out.println("DNA is "+ dna);
getAll(dna);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
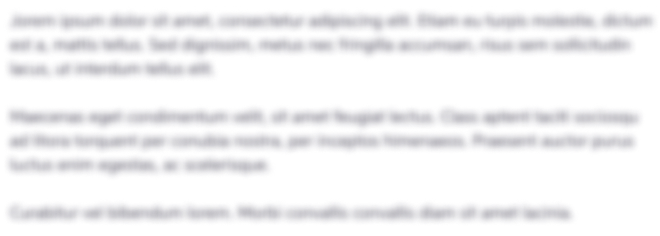
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started