Question
Java Period ADT(only need help with the first class provided. A period is an extent of historical time. The toString() method should return a string
Java Period ADT(only need help with the first class provided.
A period is an extent of historical time. The toString() method should return a string of the form [time; duration].
A period has a start time, a length of time and a stop time, but only two of these are neccessary to a specifc period. you should write three constructors: one omitting the stop, one the length and one the start time. You may use two or three fields in your class, but these fields should be instances of the other classes(provided below as Duraction, and Time, they should not be long fields.
getStart() Return the time that this period begins.
getStrop() Return the time that this period ends.
getLength() Return the length of time of this period.
------------------------Period.java-------------------------------------- /** * A time period within historical time. */ public class Period { // TODO: Fields (should be final) // Use the ADTs created already /** * Construct a period given the start time and length. * @param s start time, must not be null * @param l length, must not be null */ public Period(Time s, Duration l) { // TODO } /** * Construct a period given the start and end time * @param from start time, must not be null * @param to end time, must not be null or before the start time */ public Period(Time from, Time to) { // TODO } /** * Construct a period given the length and end time. * @param len length of the period, must not be null * @param end end time of the period. */ public Period(Duration len, Time end) { // TODO } /** * Return the start time of the period. * @return beginning time */ public Time getStart() { return null; // TODO } /** * Return the stop time of the period. * @return end time */ public Time getStop() { return null; // TODO } /** * Return the length of the period. * @return the amount of time in this period */ public Duration getLength() { return null; // TODO } @Override public boolean equals(Object x) { return false; // TODO // NB: If you find yourself failing tests using assertEquals, // watch out that you defined this method correctly // Remember that for immutable ADTs, one shouldn't use ==. } @Override public int hashCode() { return 0; // TDDO: return Objects.hash(...all the fields...); } @Override public String toString() { return null; // TODO } }
------------------Duration.java------------------------------
import java.util.Objects;
/** * An amount of time, always positive. * To create a duration, scale an existing duration. */ public class Duration { private final long extent; // this must remain private. Do NOT add a getter! // this constructor must remain private: private Duration(long milliseconds) { extent = milliseconds; } // TODO: For all constants, have a line: // public static final Duration CONSTANT = new Duration(...);
public static final Duration INSTANTANEOUS = new Duration(0); public static final Duration MILLISECOND = new Duration(1); public static final Duration SECOND = new Duration(1000); // A long literal needs a "L" at the end, as in 1234567890L
// If you are overriding a method from a super class, always // annotate it "@Override" as here, overriding Object#equals(Object) @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; Duration other = (Duration) obj; if (extent != other.extent) return false; return true; } @Override public int hashCode() { // the recommended way to define hashCode: // call Objects.hash(...) listing all the fields. // The "hash" method takes multiple parameters return Objects.hash(extent); } // If you are overriding a method from an interface, then Java 5 // says you CANNOT use Override, but anything later says you MAY. // Please always do unless you write a javadoc comment. @Override public String toString() { return null; // TODO: print different things depending on the extent } // Methods that are not standard or private must have a documentation comment: /** * Add two durations together to get a larger duration. * @param d duration being added to this, must not be null * @return new duration equal to the combined duration of this and the argument. * @throws NullPointerException if d is null */ public Duration add(Duration d) { // return null; // TODO (one single line of code) // Remember that you can access the fields of "d" // even though they are private, because this is your class! if(d == null){ throw new NullPointerException(); } return new Duration(this.extent + d.extent); } /** * Return the duration remaining if the argument duration * 'is removed. The argument must not be longer than this. * @param d duration to remove, must not be null, must not be bigger than this * @return remaining duration after subtracting d * @throws NullPointerException if d is null * @throws ArithmeticException if d is too big */ public Duration subtract(Duration d) { if(d == null){ throw new NullPointerException(); } long buffer = this.extent - d.extent; if(buffer > 0) return new Duration(buffer); else throw new ArithmeticException(); }
/** * Return the duration by scaling this duration by the given amount. * The result is rounded. * @param d amount to scale by, must not be negative. * @return new scaled duration * @throws IllegalArumentException if scale factor is negative. */ public Duration scale(double d) { //return null; // TODO (2 lines of code) // Read the Javadoc if you find yourself failing tests. if(d < 0){ throw new IllegalArgumentException(); } return new Duration(new Double(this.extent - d).longValue()); } /** * Return the scale of this duration against the argument. * @param d duration to divide by, must not be null or instantaneous * @return how many of the argument fit in this duration * @throws NullPointerException if d is null * @throws ArithmeticException if d is instantaneous */ public double divide(Duration d) { //return null; // TODO (2 lines of code) //Please give a more clear example return 0d; } }
--------------------Time.java-------------------------------------------
import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Calendar; import java.util.Date; import java.util.Objects; import java.util.TimeZone; import java.time.Duration; import java.util.Calendar;
/** * A point in time. */ public class Time { // representing milliseconds since the Java epoch. // The solution also has a private constructor, // which is very useful -- we recommend you declare it here. final long milliseconds; /** * Create a time for now. */ public Time() { this.milliseconds = System.currentTimeMillis(); }
/** * Create a time according to the time parameter. * @param c calendar object representing a time, must not be null */ public Time(Calendar c) { this.milliseconds = c.getTimeInMillis(); }
// Override/implement four methods standard for immutable classes.
/** * Return the difference between two time points. * The order doesn't matter --- the difference is always a * (positive) Duration. * @param t time point to compute difference with * @return duration between two time points. */ public Duration difference(Time t) { Duration duration= Duration.ofMillis(Math.abs(this.milliseconds - t.milliseconds)); return duration; // Duration has an easy way to create 'n' times some constant duration }
/** * Return the time point after a particular duration. * If the point advances too far into the future, * more than a hundred million years from now, this * method may malfunction. * @param d duration to advance, must not be null * @return new time after given duration */ public Time add(Duration d) {
Time currentTime = new Time(); // Add duration to current time. long millisec = currentTime.milliseconds + d.getSeconds() * 1000; // to convert seconds to millis multiply by 1000 Calendar calendar = Calendar.getInstance(); calendar.setTimeInMillis(millisec); Time time = new Time(calendar); return time; // TODO (one line of code) // Duration has an easy way to ask how many of some constant Duration it is. }
/** * Return the time point before a particular duration. * If a point regresses too far into the past, * more than a hundred million years from now, * this method may malfunction. * @param d duration to regress, must not be null * @return new time before this one by the given duration. */ public Time subtract(Duration d) {
Time currentTime = new Time(); // Subtract duration to current time. long millisec = currentTime.milliseconds - d.getSeconds() * 1000; // to convert seconds to millis multiply by 1000 Calendar calendar = Calendar.getInstance(); calendar.setTimeInMillis(millisec); Time time = new Time(calendar); return time; // Very similar to add } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
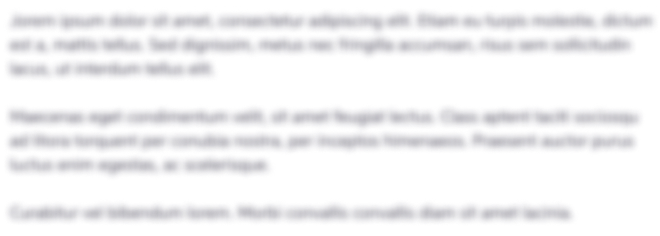
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started