Question
Java Person class (abstract) Instance variables: firstName(String), lastName(String), email(String), phoneNumber (long). Employee class will be inheriting this class. So, think about the access specifiers for
Java
Person class (abstract)
Instance variables: firstName(String), lastName(String), email(String), phoneNumber (long). Employee class will be inheriting this class. So, think about the access specifiers for the instance variables.
Constructor: parameterized constructor that gets values to set all properties of a person (Its up to you to think and decide if a no-arg constructor is needed)
Methods:
- readInfo(): accepts a Scanner object, returns nothing. Reads personal information.
- printInfo(): abstract method. Definition should be provided in the classes that inherit this class.
What I have:
Person.java import java.util.Scanner; public abstract class Person { protected String firstName, lastName, email; protected long phoneNumber; public Person(String firstName, String lastName, String email, long phoneNumber) { this.firstName = firstName; this.lastName = lastName; this.email = email; this.phoneNumber = phoneNumber; } public void readInfo(Scanner sc) { System.out.println("Enter the first name of the person: "); firstName = sc.next(); System.out.println("Enter the last name of the person: "); lastName = sc.next(); System.out.println("Enter the email of the person: "); email = sc.next(); System.out.println("Enter the phone number of the person: "); phoneNumber = sc.nextLong(); } public abstract void printInfo(); }
Employee class (extends Person)
Instance variables: employeeNumber (int)
Constructor: parameterized constructor that initializes an employee with employee number and all personal properties. As you have a parameterized constructor for Person class, invoke that to set personal properties.
Methods:
- readInfo():accepts a Scanner object, returns nothing. Reads all employee information. For reading personal information, you need to invoke readInfo() method of the super class.
- printInfo(): accepts nothing, returns nothing. This method prints details of an employee using formatted output (use printf).
What I have:
Employee.java import java.util.Scanner; public class Employee extends Person { private int employeeNumber; public Employee(String firstName, String lastName, String email, long phoneNumber, int employeeNumber) { super(firstName, lastName, email, phoneNumber); this.employeeNumber = employeeNumber; } public void readInfo(Scanner sc) { super.readInfo(sc); System.out.println("Enter the employee number: "); employeeNumber = sc.nextInt(); } @Override public void printInfo() { System.out.printf("Employee number: %d", employeeNumber); System.out.printf(" Employee name: %s %s", firstName, lastName); System.out.printf(" Email: %s", email); System.out.printf(" Phone number: %d", phoneNumber); } }
Regular class (extends Employee)
Instance variables: salary (double)
Methods:
- readInfo(): accepts a Scanner object, returns nothing. Overrides the readInfo() method. In the overridden definition, make a call to the readInfo() method of the parent class. Then, reads annual salary from the user, converts it to monthly salary and store it in salary instance variable.
- printInfo(): accepts nothing, returns nothing. Overrides the printInfo() method. In the overridden definition, make a call to the printInfo() of the parent class. Then, prints salary info (formatted output).
Contractor class (extends Employee)
Instance variables: hourlyRate (double), numHours (double)
Methods:
- readInfo(): accepts a Scanner object, returns nothing. Overrides the readInfo() method. Make a call to the readInfo() method of the parent class. Then, reads hourly rate and number of hours worked.
- printInfo(): accepts nothing, returns nothing. Overrides the printInfo() method. Make a call to the printInfo() of the parent class. Then, prints salary, which is the product of hourly rate and the number of hours worked (formatted output).
Store class
Instance variables: an array of Employee named employees
Constructor: parameterized constructor that creates the array of employees with the given size (this size will be read in main(), and will be sent here when creating the Store object)
Methods:
- readDetails(): accepts nothing, returns nothing. In a for loop, read details of all employees. First, read the type of the employee. Based on the type of the employee, corresponding array object needs to be created (Polymorphism). Then, call readInfo() method.
- printDetails(): accepts nothing, returns nothing. In a for loop, call printInfo() to print details of all employees.
- printLine(): static method that prints a line using =
- printTitle(): static method that prints the title of the output. This method gets the name of the store as a parameter, which will be used in the formatted print statement. printLine() method will be called from this method to print lines.
Lab7 class
This is the driver class (test class), which means this class has the main method.
Main method
- This method read the name of the store (example: Amazon) and the number of employees (stored in num).
- A Store object will be created with the size num.
- Until usert enters option 3, show options to read, print, quit to the user
- Based on users input, invoke corresponding methods (check output to see the flow).
Format your code with proper indentation and formatting. Your code should be properly commented.
Expected Output
Enter name of the store: Amazon
How many employees do you have? x
*****Input Mismatch Exception while reading number of employees*****
How many employees do you have? -9
How many employees do you have? 0
How many employees do you have? 3
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: y
*****Input Mismatch Exception while reading selection of process*****
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: 7
Invalid option.... please try again...
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: 1
Enter details of employee 1
- Regular
- Contractor
Enter type of employee: 1
Enter Employee Number: 1020
Enter first name: Dany
Enter last name: Roy
Enter email: Roy@test.com
Enter phone number: 123456
Enter annual salary: 85000 1. Read Employee Details
- Print Employee Details
- Quit
Enter your option: 2
================================================================================
Amazon Store Management System
================================================================================
Emp# | Name | Email | Phone | Salary|
================================================================================
1020 | Dany Roy | Roy@test.com | 123456 | 7083.33 |
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: 5
Invalid option.... please try again...
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: 1
Enter details of employee 2
1. Regular
2. Contractor
Enter type of employee: 2
Enter Employee Number: 1025
Enter first name: Tom
Enter last name: Willy
Enter email: tom@test.com
Enter phone number: 45698712
Enter hourly rate: 25
Enter number of hours worked: 40
1. Read Employee Details
2. Print Employee Details
3. Quit
Enter your option: 1
Enter details of employee 3
- Regular
- Contractor
Enter type of employee: 1
Enter Employee Number: 1028
Enter first name: Matt
Enter last name: James
Enter email: matt@test.com
Enter phone number: 321456
Enter annual salary: 75550
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: 2
================================================================================
Amazon Store Management System
================================================================================
Emp# | Name | Email | Phone | Salary|
================================================================================
1020 | Dany Roy | Roy@test.com | 123456 | 7083.33 |
1025 | Tom Willy | tom@test.com | 45698712 | 1000.00 |
1028 | Matt James | matt@test.com | 321456 | 6295.83 |
- Read Employee Details
- Print Employee Details
- Quit
Enter your option: 3
Goodbye... Have a nice day!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
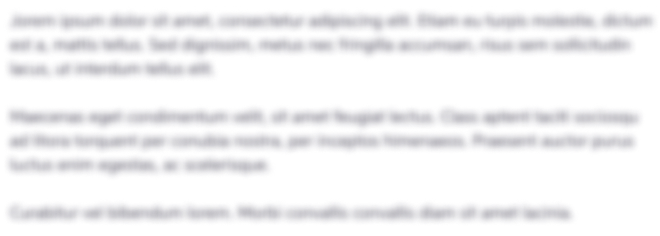
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started