Question
[JAVA] please fix errors import java.util.Arrays; import java.util.ArrayList; public class ShortestDistance { public static void main(String[] args) { double[][] points = new double [1000][2]; for(int
[JAVA]
please fix errors
import java.util.Arrays; import java.util.ArrayList; public class ShortestDistance { public static void main(String[] args) {
double[][] points = new double [1000][2];
for(int i = 0; i < points.length; i++) // i instead of ixq {
points[i][0] = Math.random()*100; points[i][1] = Math.random()*100;
}
long startTime = System.currentTimeMillis(); Pair pair = closestPairBruteForce(points);
System.out.println("shortest distance is" + pair.getDistance()); System.out.println(" "); System.out.println(pair); System.out.println(" "); long endTime = System.currentTimeMillis(); System.out.println("BFA time: " + (endTime - startTime) + "milliseconds"); startTime = System.currentTimeMillis(); Pair closestPair = getClosestPair(points); System.out.println("shortest distance is" + closestPair.getDistance() ); System.out.println(" "); System.out.println(closestPair); System.out.println(" "); endTime = System.currentTimeMillis(); System.out.println("Time spent on algorithm is " + (endTime - startTime) + "milliseconds"); }
/////////next class
public static Pair getClosestPair(double[][] points) { Point[] pointsOrderedOnX = new Point[points.length]; for(int i = 0; i < pointsOrderedOnX.length; i++) pointsOrderedOnX[i] = new Point(points[i][0], points[i][1]); return getClosestPair(pointsOrderedOnX); }
public static Pair getClosestPair(Point[] points) { Arrays.sort(points); Pair pair = checkIdentical(points); if(pair!= null) return pair;
Point[] pointsOrderedOnY = points.clone(); Arrays.sort(pointsOrderedOnY, new CompareY()); return distance(points,0,points.length -1, pointsOrderedOnY); }
public static Pair checkIdentical(Point[] pointsOrderedOnX) { Pair pair = new Pair(); for(int i = 0; i < pointsOrderedOnX.length -1; i++) { if(pointsOrderedOnX[i].compareTo(pointsOrderedOnX[i + 1]) == 0) { pair.p1 = pointsOrderedOnX[i]; pair.p2 = pointsOrderedOnX[i + 1]; return pair; } } return null; } public static Pair distance(Point[] pointsOrderedOnX, int low, int high, Point[] pointsOrderedOnY) { if(low>=high) return null; else if (low + 1 ==high)
{ Pair pair = new Pair(); pair.p1 = pointsOrderedOnX[low]; pair.p2 = pointsOrderedOnX[high]; return pair;
}
int mid =(low+high) / 2; Pair pair1 = distance(pointsOrderedOnX,low,mid,pointsOrderedOnY); Pair pair2 = distance(pointsOrderedOnX,mid + 1,high,pointsOrderedOnY); double dis; // dis instead of di Pair pair = null;
if(pair1 ==null && pair == null)
{ dis = Double.MAX_VALUE; } else if (pair1 == null)
{ dis = pair2.getDistance(); pair = pair2;
}
else if (pair2 == null)
{ dis = pair1.getDistance(); pair = pair1; }
else
{ dis = Math.min(pair1.getDistance(), pair2.getDistance()); if (pair1.getDistance() <= pair2.getDistance())
pair = pair1;
else
pair = pair2;
}
//PARTS ArrayList partOne = new ArrayList(); //partOne instead of stripA ArrayList partTwo = new ArrayList(); //partTwo instead of stripB
for(int i = 0; i < pointsOrderedOnY.length; i++)
if(pointsOrderedOnY[i].x <= pointsOrderedOnX[mid].x && pointsOrderedOnY[i].x >= pointsOrderedOnX[mid].x - dis)
partOne.add(pointsOrderedOnY[i]); else
partTwo.add(pointsOrderedOnY[i]);
double hold = dis; //hold instead of d4
int ftw = 0; //wtf instead of ki for(int i = 0; i < partOne.size(); i++)
{ while ( ftw < partTwo.size() && partOne.get(i).y > partTwo.get(ftw).y + dis) ftw++;
int omg = ftw;
while ( omg < partTwo.size() && partTwo.get(omg).y <= partOne.get(i).y + dis)
{
if ( hold > distance(partOne.get(i), partTwo.get(omg))) {
hold = distance(partOne.get(i), partTwo.get(omg)); pair.p1 = partOne.get(i); pair.p2 = partTwo.get(omg); } omg++; } } return pair; }
public static double distance(Point p1, Point p2) { return distance(p1.x, p1.y, p2.x, p2.y);
} public static double distance(double x1, double y1, double x2, double y2) { return Math.sqrt((x2 - x1) * (x2 - x1) + (y2 - y1) * (y2 - y1));
}
static class Point implements Comparable
{ double x; double y;
Point(double x, double y) { this.x = x; this.y = y;
}
@Override public int compareTo(Point p2)
{ if(this.x < p2.x) return -1; else if(this.x == p2.x)
{ if(this.y < p2.y) return -1; else if(this.y == p2.y) return 0; else return 1; } else return 1; } }
static class CompareY implements java.util.Comparator {
public int compare(Point p1, Point p2) { if (p1.y < p2.y ) return -1; else if (p1.y == p2.y)
{ if (p1.x < p2.x) return -1; else if (p1.x == p2.x) return 0; else return 1;
} else return 1; } }
public static class Pair { Point p1; Point p2;
public double getDistance() { return distance(p1,p2);
}
@Override public String toString() { return "(" + p1.x + "," + p1.y + ") and " + "(" + p2.x + "," + p2.y + ")";
} }
public static Pair closestPairBruteForce(double[][] points) { Point[] loca = new Point[points.length]; //pq = loca for (int i = 0; i < loca.length; i++) loca[i] = new Point(points[i][0], points[i][1]); return closestPairBruteforce(loca);
}
public static Pair closestPairBruteForce(Point[] points) { Pair pair = new Pair(); if (points.length < 2) return null; pair.p1 = points[0]; pair.p2 = points[1];
double shortestDistances = distance(pair.p1, pair.p2);
for(int i = 0; i < points.length; i++) { for(int j = i + 1; j < points.length; j++)
{
double distance = distance(points[i], points[j]); if (distance < shortestDistances)
{ pair.p1 = points[i]; pair.p2 = points[j];
shortestDistances = distance;
} } }
return pair;
} }// final bracket
Step by Step Solution
There are 3 Steps involved in it
Step: 1
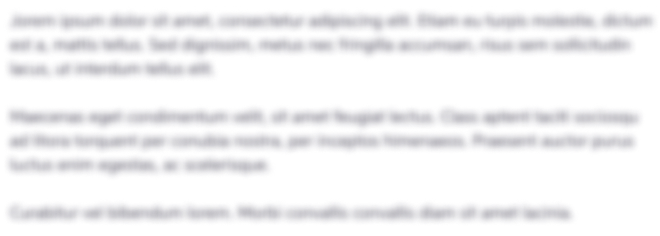
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started