Answered step by step
Verified Expert Solution
Question
1 Approved Answer
java please follow all steps Objective In this assignment, you are asked to implement a bag collection using a linked list and, in order to
java please follow all steps
Objective In this assignment, you are asked to implement a bag collection using a linked list and, in order to focus on the linked list implementation details, we will implement the collection to store only one type of object of your choice (i.e., not generic). You can use the object you created for program #2. IMPORTANT: You may not use the LinkedList class from the java library. Instead, you must implement your own linked list as explained below. Requirements Implement the following four classes. "Thing": you may use the same "Thing" you created for the previous assignment. But make sure to look for the requirements below and change your previous implementation as necessary. ThingNode; a linked list Node where the data part is of type "Thing". ThingLinkedBag: a collection of "Things" in which the elements are stored in an unordered linked list. ThingLinkedBagDriver: includes a main method to test the functionality of your collection. Class "Thing" You may use the same "Thing" class you created in Assignment 2 but make sure all the following requirements are met. Has exactly 3 instance variables such that: o One instance variable must be an integer (i.e, int) and this variable will be used to find aggregate information about "Things" that are stored in a collection class as will be explained later. o One instance variable must be of type String and this variable will be used as a search key. o The third variable can be any type based on the semantics of your "Thing". An implementation of the toString() method where a "Thing" is represented in one line. Implement the equals() methods to test the equality of two things. Implement the Comparable interface and include the comparete () method. Note 1: Do not name your class Thing.java. Give your class a name that is indicative to the type of elements that you are maintaining. Also, the name of your class should be singular not plural (e.g, Car not Cars) because this class is used to represent only one element. Note 2: the name of all the other classes should be changed to indicate the type of things you are maintaining. For example, if you choose to implement a collection of cars, then your classes should be named Car, CarNode, CarlinkedBag, and CardinkedBagdriver class "Thingdede." This class represents a node in the linked list. The node class should include the following: Two private instance variables: o data of type "Thing". The data part must be of type "Thing". Do not use a generic node. o link of type "ThingNode" A constructor that takes two input parameters and use them to initialize the two instance variables. The following instance methods which are the same as in the IntNode class that we discussed in class and also are discussed in the text book (Chapter 4, Section 4.2). Change the methods as appropriate to reflect your new type of data. o getData(), gettink(), se trata(), settinko, add adeAfter), removedadeAfter (). The following static methods that are the same as those in the IntNode class. Change the methods to accommodate your "Thing" data type as appropriate. o display(), histlesition (), Listhength() and ListSearch(). class ThingLinkedBag This class is used to manage a collection of things where the things are stored in a linked list (not an array). This is discussed in Section 4.4 of the text book. The requirements for this class are as follows: Two instance variables: o head of type ThingNode: this represents the head of the linked list that stores your collection. o many items of type int; this represent the size of the linked list. Implement the following instance methods in your collection class. Note that all the method headers below are written in terms of Thing, however, your implementation should replace each Thing with your specific data type. 1. A constructor to create an empty linked list. int size(): returns the number of nodes in the list. void display ( ) : displays the contents of the collection such that each element is displayed on one line. Note that this method displays the list on the screen and does NOT return a String representation of the list. void add (Thing element): a method to add a thing to the collection. 5. void add (int, index, Thing element): a method to add an element at a specific index in the collection assuming the first node (referenced by head) is at index 1. If the index is greater than the collection length, then the element is added as the last element in the collection. The method does not do anything if index is negative. baslean remove (Thing target): this method removes one occurrence of the target from the list if any such thing exists in the list. The method returns true if an item is removed and false otherwise. 9. 7. baalean remove(int index): this method removes the element that is located at position index in the linked list where the first node (referenced by head) is at index 1. The method returns true if an item is removed and false if no element is removed because index is negative or beyond the list length. 8. int countRange (Thing start, Thing end): this method counts and returns how many element in the collection fall in the range between start and end inclusive. Note that equality of things are compared using the comparete method from your "Thing" class. Thing grab (int index): returns the element of the node located at position index in the list or null if index is past the end of the list. Note that this method does not remove the element from the list. 10. int indexOf(Thing target): returns the index of the node that contains the target where the first node (referenced by head) is at index 1. If the target is not found, the method returns -1 and if there is more than one occurrence, then the method returns the index of the first occurrence. 11. void set (int, index, Thing element): replaces the Thing element at position index with the input element. If index is negative or beyond the length of the list, then the method does not do anything. barnean replace (Thing andthing Thing newthing): this method takes two Thing inputs, oldthing and newthing. The method then searches the collection for an element that is equal to oldthing, If found, the method replaces (only one occurrence of) oldthing with newThing while maintaining the linked list order (i.e., don't delete and then re-insert). On the other hand, if oidThing is not found in the collection, the method does not do anything. The method then returns true or false based on whether a replacement took place or not. 13. int tatalValue(): this method returns the sum of all the integer values of all things in the list (remember that it was required that each "Thing" has an integer attribute). 14. ThingNode lessthan (Thing element): this method takes one "Thing" as input and returns as output a linked list that includes all elements that are less than or equal to the input element. Note that "Things" are ordered based on the comparete () method from the Thing class. 15. ThingNode greaterThan (Thing element): similar to the less than method, this method takes one thing as input and returns as output a linked list that includes all elements that are greater than the input element. Note that "Things" are ordered based on the comparete () method from the thing class. 16. Thing getMax(): this method returns the maximum "Thing" in the linked list where things are ordered based on the comparete () method. 17. Thing getMin(): this method returns the minimum "Thing" in the linked list where things are ordered based on the comparete () method. class ThingLinkedragerixen Write a driver class to test ALL the methods that you implemented in the ThingNode class and the Thingtinkedrag class. Display the results of each operation after it is performed. Do not use a Scanner to read any inputs from the user. Instead, use hard coded values for the different methods' inputs. Other Requirements Comment the ThingLinkedrag class with lavadocs.style comments. Include the invariant in the comments of the ThingLinkedBag, class. Use good coding style throughout including o correct private/public access modifiers o consistent indenting o meaningful variable names o normal capitalization conventions o other aspects of easy-to-read code Document the source of any borrowed code Factor the classes as described above - Thing, Collection, Driver are all in separate classes No UML diagrams are required for this program Objective In this assignment, you are asked to implement a bag collection using a linked list and, in order to focus on the linked list implementation details, we will implement the collection to store only one type of object of your choice (i.e., not generic). You can use the object you created for program #2. IMPORTANT: You may not use the LinkedList class from the java library. Instead, you must implement your own linked list as explained below. Requirements Implement the following four classes. "Thing": you may use the same "Thing" you created for the previous assignment. But make sure to look for the requirements below and change your previous implementation as necessary. ThingNode; a linked list Node where the data part is of type "Thing". ThingLinkedBag: a collection of "Things" in which the elements are stored in an unordered linked list. ThingLinkedBagDriver: includes a main method to test the functionality of your collection. Class "Thing" You may use the same "Thing" class you created in Assignment 2 but make sure all the following requirements are met. Has exactly 3 instance variables such that: o One instance variable must be an integer (i.e, int) and this variable will be used to find aggregate information about "Things" that are stored in a collection class as will be explained later. o One instance variable must be of type String and this variable will be used as a search key. o The third variable can be any type based on the semantics of your "Thing". An implementation of the toString() method where a "Thing" is represented in one line. Implement the equals() methods to test the equality of two things. Implement the Comparable interface and include the comparete () method. Note 1: Do not name your class Thing.java. Give your class a name that is indicative to the type of elements that you are maintaining. Also, the name of your class should be singular not plural (e.g, Car not Cars) because this class is used to represent only one element. Note 2: the name of all the other classes should be changed to indicate the type of things you are maintaining. For example, if you choose to implement a collection of cars, then your classes should be named Car, CarNode, CarlinkedBag, and CardinkedBagdriver class "Thingdede." This class represents a node in the linked list. The node class should include the following: Two private instance variables: o data of type "Thing". The data part must be of type "Thing". Do not use a generic node. o link of type "ThingNode" A constructor that takes two input parameters and use them to initialize the two instance variables. The following instance methods which are the same as in the IntNode class that we discussed in class and also are discussed in the text book (Chapter 4, Section 4.2). Change the methods as appropriate to reflect your new type of data. o getData(), gettink(), se trata(), settinko, add adeAfter), removedadeAfter (). The following static methods that are the same as those in the IntNode class. Change the methods to accommodate your "Thing" data type as appropriate. o display(), histlesition (), Listhength() and ListSearch(). class ThingLinkedBag This class is used to manage a collection of things where the things are stored in a linked list (not an array). This is discussed in Section 4.4 of the text book. The requirements for this class are as follows: Two instance variables: o head of type ThingNode: this represents the head of the linked list that stores your collection. o many items of type int; this represent the size of the linked list. Implement the following instance methods in your collection class. Note that all the method headers below are written in terms of Thing, however, your implementation should replace each Thing with your specific data type. 1. A constructor to create an empty linked list. int size(): returns the number of nodes in the list. void display ( ) : displays the contents of the collection such that each element is displayed on one line. Note that this method displays the list on the screen and does NOT return a String representation of the list. void add (Thing element): a method to add a thing to the collection. 5. void add (int, index, Thing element): a method to add an element at a specific index in the collection assuming the first node (referenced by head) is at index 1. If the index is greater than the collection length, then the element is added as the last element in the collection. The method does not do anything if index is negative. baslean remove (Thing target): this method removes one occurrence of the target from the list if any such thing exists in the list. The method returns true if an item is removed and false otherwise. 9. 7. baalean remove(int index): this method removes the element that is located at position index in the linked list where the first node (referenced by head) is at index 1. The method returns true if an item is removed and false if no element is removed because index is negative or beyond the list length. 8. int countRange (Thing start, Thing end): this method counts and returns how many element in the collection fall in the range between start and end inclusive. Note that equality of things are compared using the comparete method from your "Thing" class. Thing grab (int index): returns the element of the node located at position index in the list or null if index is past the end of the list. Note that this method does not remove the element from the list. 10. int indexOf(Thing target): returns the index of the node that contains the target where the first node (referenced by head) is at index 1. If the target is not found, the method returns -1 and if there is more than one occurrence, then the method returns the index of the first occurrence. 11. void set (int, index, Thing element): replaces the Thing element at position index with the input element. If index is negative or beyond the length of the list, then the method does not do anything. barnean replace (Thing andthing Thing newthing): this method takes two Thing inputs, oldthing and newthing. The method then searches the collection for an element that is equal to oldthing, If found, the method replaces (only one occurrence of) oldthing with newThing while maintaining the linked list order (i.e., don't delete and then re-insert). On the other hand, if oidThing is not found in the collection, the method does not do anything. The method then returns true or false based on whether a replacement took place or not. 13. int tatalValue(): this method returns the sum of all the integer values of all things in the list (remember that it was required that each "Thing" has an integer attribute). 14. ThingNode lessthan (Thing element): this method takes one "Thing" as input and returns as output a linked list that includes all elements that are less than or equal to the input element. Note that "Things" are ordered based on the comparete () method from the Thing class. 15. ThingNode greaterThan (Thing element): similar to the less than method, this method takes one thing as input and returns as output a linked list that includes all elements that are greater than the input element. Note that "Things" are ordered based on the comparete () method from the thing class. 16. Thing getMax(): this method returns the maximum "Thing" in the linked list where things are ordered based on the comparete () method. 17. Thing getMin(): this method returns the minimum "Thing" in the linked list where things are ordered based on the comparete () method. class ThingLinkedragerixen Write a driver class to test ALL the methods that you implemented in the ThingNode class and the Thingtinkedrag class. Display the results of each operation after it is performed. Do not use a Scanner to read any inputs from the user. Instead, use hard coded values for the different methods' inputs. Other Requirements Comment the ThingLinkedrag class with lavadocs.style comments. Include the invariant in the comments of the ThingLinkedBag, class. Use good coding style throughout including o correct private/public access modifiers o consistent indenting o meaningful variable names o normal capitalization conventions o other aspects of easy-to-read code Document the source of any borrowed code Factor the classes as described above - Thing, Collection, Driver are all in separate classes No UML diagrams are required for this programStep by Step Solution
There are 3 Steps involved in it
Step: 1
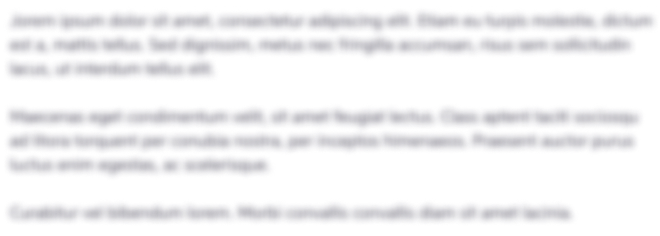
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started