Question
JAVA (PLEASE SHOW YOUR MATH) Loop and Recursive Factorial Function: We want to measure the elapsed time for these computations, so heres some timer code:
JAVA (PLEASE SHOW YOUR MATH) Loop and Recursive Factorial Function:
We want to measure the elapsed time for these computations, so heres some timer code:
long t1 = System.currentTimeMillis();
// do something that requires a lot of time
long t2 = System.currentTimeMillis();
System.out.println("The elapsed time is " + ( t2 - t1 ) / 1000. + " seconds.");
The two methods are
public int factorial_loop(int n){}
public int factorial_recursive(int n){}
Determine the largest input value n for which a factorial can be computed using int.
What was the largest result for factorial using int? n = ______________
n! = ______________
What was the elapsed time computing this factorial using loops? t = _______________
Change the data type to long and repeat the above tests. n = ______________
n! = _____________
What was the elapsed time computing this factorial using loops? t = _______________
What is the elapsed time computing this factorial using recursion? t = _______________
Are these times significantly different? Why or why not? Answer in the space below:
Change the data type to BigInteger. Can you find a value for which it requires 1.0 seconds to compute the factorial? Give the number of digits in v, not the number itself.
Number of digits in v =
Loop and Recursive Fibonacci Function:
Write two functions to compute Fibonacci numbers: one using a loop, and the other recursive as described in class. The two methods are
public long fibonacci_loop(int f){}
public long fibonacci_recursive(int f){}
How much time is required to compute the following Fibonacci numbers using a loop?
n = 10 time required: ___________
n = 50 time required: ___________
n = 90 time required: ___________
How much time is required to compute the following Fibonacci numbers using recursion?
n = 30 time required: ___________
n = 40 time required: ___________
n = 41 time required: ___________
n = 42 time required: ___________
n = 43 time required: ___________
n = 44 time required: ___________
n = 45 time required: ___________
n = 46 time required: ___________
n = 47 time required: ___________
n = 48 time required: ___________
n = 49 time required: ___________
n = 50 time required: ___________
Determine the largest values of n for which the corresponding Fibonacci Numbers can be computed within the given time.
n = _____ time required: less than 10 seconds
n = _____ time required: less than 30 seconds
n = _____ time required: less than 1 minute
n = _____ time required: less than 5 minutes
Estimate the value of n for the largest value of fib(n) that can be computed using recursion in one hour on your computer. Explain in detail how you estimated this value. Also describe any additional data you took to arrive at this value. Attach any additional work required.
n = ______ for time required = 1 hour (3600 seconds)
Repeat this problem for the largest value of n for which the corresponding Fibonacci number can be computed in one day (86,400 seconds).
n = ______ for time required = 1 day (86,400 seconds)
Repeat this problem for the largest value of n for which the corresponding Fibonacci number can be computed in one year.
n = ______ for time required = 1 year.
Repeat this problem for the largest value of n for which the corresponding Fibonacci number can be computed in one million years (106 years).
n = ______ for time required = 1 million years.
Memoization
The time complexity is exponential so this simplistic recursive approach becomes intractable when we get into the fifties or so. We can greatly increase our time performance with memoization that is, every time we compute a value we make a note (or memo) of it and check that memo list before computing.
Add memoization to your Fibonacci program. At what point does the computation time become more than one second?
Fixed-Width Integers
Now our program is fast; the next problem is that in the mid-nineties the size of the Fibonacci number exceeds the capacity of the long data type.
What is the largest Fibonacci Number that you can successfully compute using long?
n = _________
To compute larger Fibonacci numbers we must make use of arbitrary-length integers: the BigInteger class. Modify your program to use BigInteger and compute the following Fibonacci numbers:
n = 50 value =
n = 90 value =
n = 100 value =
n = 150 value =
n = 200 value =
n = 250 value =
n = 300 value =
Note: you can verify your results by looking up the values online.
At what point does your code require more than one second to run? Test with powers of ten; compute Fibonacci Numbers 100, 1000, 10000, 100000, and so on until you exceed one second. Then home in on the particular value that requires right at one second (1000 ms) to compute.
Palindromes
A palindrome is a word or sentence that reads the same forwards as it does backwards. Letter case and punctuation are always ignored. Spaces are ignored in ordinary palindromes but not in strict ones.
racecar a word that is a palindrome.
Able was I, ere I saw Elba! a strict palindrome (spaces count) attributed to Napoleon.
A man, a plan, a canal: Panama! an ordinary palindrome; spaces are ignored.
2445442 a palindromic number.
There are lots of ways to see if a String is or is not a palindrome. What would be a recursive algorithm to determine whether or not a String is a palindrome?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
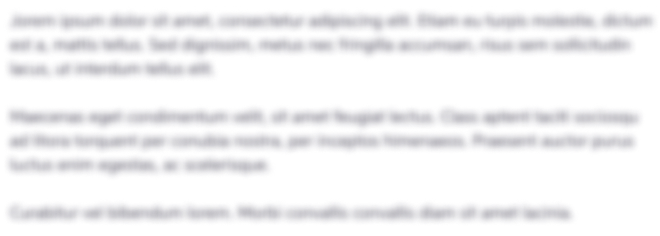
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started