Question
Java problem needs help: Create a Dot class Some specific requirements: Follow good coding practices (private instance variables, code reuse, and so on) and style
Java problem needs help:
Create a Dot classSome specific requirements:
-
Follow good coding practices (private instance variables, code reuse, and so on) and style guidelines (use your IDE's tools for fixing your code formatting before submitting).
-
The six-parameter constructor (the "workhorse constructor") should be the constructor with the most code in it. The other constructors should be made shorter by calling on the workhorse constructor.
-
If you add additional methods to the Dot class, they must be made private.
-
Document each Dot method, Dot constructor, and the Dot class itself. You are free to copy and paste the documentation you are given, or you can type documentation yourself.
--------------------------------------------------------------------------------------------------------
Class Dot
- Object
-
- Dot
-
public class Dot extends Object
Implements a dot class. A dot has a location (x, y) and radius, as well as a color specified by red, green, and blue components.
-
Constructor Summary
Constructors Constructor Description Dot() Constructs a black dot at (0.0, 0.0) with radius 1.0
Dot(double x, double y) Constructs a black dot with radius 1.0 at the given location
Dot(double x, double y, double radius) Constructs a black dot at the given location and radius
Dot(double x, double y, double radius, int r, int g, int b) Constructs a dot at the given location and radius, and a color specified by r, g, b values.
-
Method Summary
All MethodsInstance MethodsConcrete Methods Modifier and Type Method Description void brighten() Brightens this dot's color by doubling its red, green, and blue components.
void darken() Darkens this dot's color by cutting its red, green, and blue components in half.
int getBlue() Gets the blue component of this dot's color
int getBrightness() Returns the brightness of a dot, defined by the average of the red, green, and blue components of the dot
double getDistanceTo(double x, double y) Returns the distance between this dot's center and a point specified by (x, y)
double getDistanceTo(Dot that) Returns the distance between two dots' centers
double getDistanceToOrigin() Returns the distance between this dot's center and the point (0, 0)
int getGreen() Gets the green component of this dot's color
Dot getMidDot(Dot that) Returns a new dot that is halfway between this dot and the specified dot.
double getRadius() Gets the radius
int getRed() Gets the red component of this dot's color
double getX() Returns the x-coordinate
double getY() Gets the y-coordinate
void grow() Doubles the radius of this dot.
void setColor(int r, int g, int b) Sets the color of this point.
void setRadius(double newRadius) Sets the radius to a specified value
void setX(double x) Sets the x-coordinate
void setY(double y) Sets the y-coordinate
void shrink() Halves the radius of this dot.
String toString() Returns a String representation of this Dot in the form: (47.375, 18.500), radius=72.139, color=(255, 0, 0) Note that all double values are rounded to three places.
void translate(double dx, double dy) Translates this dot by increasing the x- and y-coordinates by dx and dy, respectively.
-
Methods inherited from class Object
equals, getClass, hashCode, notify, notifyAll, wait, wait, wait
-
-
Constructor Detail
-
Dot
public Dot(double x, double y, double radius, int r, int g, int b)
Constructs a dot at the given location and radius, and a color specified by r, g, b values.
Parameters:
x - the dot's x-coordinate
y - the dot's y-coordinate
radius - the dot's radius
r - the red component of the dot's color
g - the green component of the dot's color
b - the blue component of the dot's color
Throws:
IllegalArgumentException - if r, g, or b are not in the range 0 to 255 or if the radius is less than or equal to 0.
-
Dot
public Dot(double x, double y, double radius)
Constructs a black dot at the given location and radius
Parameters:
x - the x-coordinate of the dot
y - the y-coordinate of the dot
radius - the of the dot
-
Dot
public Dot(double x, double y)
Constructs a black dot with radius 1.0 at the given location
Parameters:
x - the x-coordinate of the dot
y - the y-coordinate of the dot
-
Dot
public Dot()
Constructs a black dot at (0.0, 0.0) with radius 1.0
-
-
Method Detail
-
getX
public double getX()
Returns the x-coordinate
Returns:
the x-coordinate
-
setX
public void setX(double x)
Sets the x-coordinate
Parameters:
x - the new x-coordinate
-
getY
public double getY()
Gets the y-coordinate
Returns:
the y-coordinate
-
setY
public void setY(double y)
Sets the y-coordinate
Parameters:
y - the new y-coordinate
-
getRadius
public double getRadius()
Gets the radius
Returns:
the radius
-
setRadius
public void setRadius(double newRadius)
Sets the radius to a specified value
Parameters:
newRadius - the new radius for the point
-
getRed
public int getRed()
Gets the red component of this dot's color
Returns:
the red component
-
getGreen
public int getGreen()
Gets the green component of this dot's color
Returns:
the green component
-
getBlue
public int getBlue()
Gets the blue component of this dot's color
Returns:
the blue component
-
getBrightness
public int getBrightness()
Returns the brightness of a dot, defined by the average of the red, green, and blue components of the dot
Returns:
the average of the dot's red, green, and blue components
-
setColor
public void setColor(int r, int g, int b)
Sets the color of this point. Throws an IllegalArgumentException if the r, g, or b are out of range.
Parameters:
r - the red component, where r is at least 0 and at most 255
g - the green component, where g is at least 0 and at most 255
b - the blue component, where b is at least 0 and at most 255
-
darken
public void darken()
Darkens this dot's color by cutting its red, green, and blue components in half.
-
brighten
public void brighten()
Brightens this dot's color by doubling its red, green, and blue components. If any of the components exceed 255, they will be set at 255.
-
getDistanceTo
public double getDistanceTo(Dot that)
Returns the distance between two dots' centers
Parameters:
that - the other dot
Returns:
the Euclidean distance between this dot's center and the other dot's center
-
getDistanceTo
public double getDistanceTo(double x, double y)
Returns the distance between this dot's center and a point specified by (x, y)
Parameters:
x - the x-coordinate of the point
y - the y-coordinate of the point
Returns:
the Euclidean distance between this dot's center and the point (x, y)
-
getDistanceToOrigin
public double getDistanceToOrigin()
Returns the distance between this dot's center and the point (0, 0)
Returns:
the Euclidean distance between this dot's center and the point (0, 0)
-
shrink
public void shrink()
Halves the radius of this dot. If halving the radius would cause it to be smaller than 1.0, sets the radius to 1.0.
-
grow
public void grow()
Doubles the radius of this dot. If doubling the radius would cause it to exceed 100, sets the radius to 100.
-
translate
public void translate(double dx, double dy)
Translates this dot by increasing the x- and y-coordinates by dx and dy, respectively.
Parameters:
dx - the amount by which to translate this dot in the x direction
dy - the amount by which to translate this dot in the y direction
-
getMidDot
public Dot getMidDot(Dot that)
Returns a new dot that is halfway between this dot and the specified dot. The color of the new dot will be the average of the two dots' colors. The radius of the new dot will be the average of the two dots' radii. This method does not change this or the other dot. It simply instantiates and returns a new dot.
Parameters:
that - the other dot that will be used with this dot to compute the mid-dot
Returns:
a new dot that is halfway between this dot and that dot, and with a radius and color that are the average of the radii and colors of this dot and that dot.
-
toString
public String toString()
Returns a String representation of this Dot in the form: (47.375, 18.500), radius=72.139, color=(255, 0, 0) Note that all double values are rounded to three places.
Overrides:
toString in class Object
-
---------------------------------------------------------------------------------------
-
In a separate class, DotStuff, write methods that use your Dot class. At first, you could use the main method of your class as the place to test each method and constructor as you work. But when you are done testing, you should implement the following 4 methods:
-
public static void darkDotModification(ArrayList list)
-
Takes an ArrayList of Dot objects, and modifies them as follows: each dot in the list with a brightness of less than 128 should have its radius changed to 5, and its location changed to be halfway between its current location and the origin (0, 0). So, for example, if a dot is at (200, 300), has a radius of 27, and its brightness is 100, then its radius would be changed to 5, and its location would be changed to (100, 150).
-
public static void changeDotsNear(ArrayList list, Dot center, double distance)
Takes an ArrayList of Dot objects, brightens and shrinks all the dots that are less than or equal to the specified distance away from the specified center Dot, and darkens and grows all other dots.
-
public static void writeDotsToFile(ArrayList list, String filename)
Given an arraylist of Dot objects, uses a PrintWriter to write the dot data to a file with the given filename, where each line of the output file will contain a space-separated list of data for a dot object.
The output line should be as follows for each dot: x y radius r g b
For example, if a dot is located at (6.5, 7.5) with a radius of 10.0, and a color (127, 0, 127)
Then the data in one line of the file should be: 6.5 7.5 10.0 127 0 127
-
public static ArrayList readDotsFromFile(String filename)
Given a String representing the name of a file, reads in the Dot data from that file into an ArrayList using a Scanner object. Then, returns the resulting ArrayList. Assumes the data is arranged in the same way as described in the writeDotsToFile method.
-
In the main method of DotStuff, use the provided "before.txt" data file and run the following code.
ArrayList listOne = readDotsFromFile("before.txt");
ArrayList listTwo = readDotsFromFile("before.txt");
darkDotModification(listOne);
changeDotsNear(listTwo, new Dot(500, 300), 200);
writeDotsToFile(listOne, "dark.txt");
writeDotsToFile(listTwo, "change.txt");
Step by Step Solution
There are 3 Steps involved in it
Step: 1
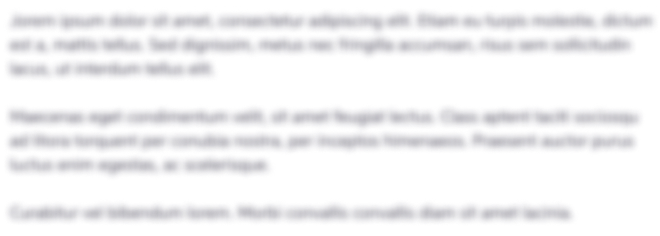
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started