Question
JAVA problem Task 5: More Complex Array Usage public static boolean anyDuplicates(int[] xs) Given an array of integers, if the same value occurs twice anywhere,
JAVA problem
Task 5: More Complex Array Usage
public static boolean anyDuplicates(int[] xs)
Given an array of integers, if the same value occurs twice anywhere, return true; if not, return false.
reminder: Although Python used upper-case words (True and False), we're not writing Python! In Java, the boolean values are written all lowercase: true, false.
public static int largestAscent(int[] xs)
Given an array of integers, we can conceptually split it into separate non-decreasing sequences. Each sequence has a first and last item, and thus spans some range of values. Find the sequence that has the largest gap from its beginning low-point to its ending high-point, and return this distance.
Examples:
sequence | largest ascent | reasoning |
---|---|---|
1,2,3,4,2,10 | 8 | (10-2) is greater than (4-1) |
1,5,5,10,2,9 | 9 | 1,5,5,10 counts as one ascent. |
5 | 0 | degenerate case; (5-5)==0. |
Manual Inspection Criteria(5%) Solve this one with a single loop (any kind).
Solutions go here:
public class Task5 { /* given an array of ints, are there any duplicates in the list? */ public static boolean anyDuplicates(int[] xs){ // delete this when you implement it. throw new RuntimeException("not implemented!"); return false; } /* given an array of ints, split into non-descending subsequences, * what is the largest difference from first to last? */ public static int largestAscent(int[] xs){ // delete this when you implement it. throw new RuntimeException("not implemented!"); } }
Tester:
import org.junit.*; import static org.junit.Assert.*; import java.util.*; public class Task5Tests { public static void main(String args[]){ org.junit.runner.JUnitCore.main("Task5Tests"); } @Test public void task5_anyDuplicates_01() { assertEquals( false, Task5.anyDuplicates( new int[]{} )); } @Test public void task5_anyDuplicates_02() { assertEquals( false, Task5.anyDuplicates( new int[]{5} )); } @Test public void task5_anyDuplicates_03() { assertEquals( false, Task5.anyDuplicates( new int[]{1,2,3,4} )); } @Test public void task5_anyDuplicates_04() { assertEquals( true , Task5.anyDuplicates( new int[]{3,4,5,6,7,4,8} )); } @Test public void task5_anyDuplicates_05() { assertEquals( true , Task5.anyDuplicates( new int[]{7,6,5,2,2} )); } @Test public void task5_anyDuplicates_06() { assertEquals( true , Task5.anyDuplicates( new int[]{1,1,1,1,1,1,1,1,1} )); } @Test public void task5_anyDuplicates_07() { assertEquals( false, Task5.anyDuplicates( new int[]{6,4} )); } @Test public void task5_anyDuplicates_08() { assertEquals( true , Task5.anyDuplicates( new int[]{1,2,3,2,0} )); } @Test public void task5_anyDuplicates_09() { assertEquals( false, Task5.anyDuplicates( new int[]{1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20} )); } @Test public void task5_anyDuplicates_10() { assertEquals( true , Task5.anyDuplicates( new int[]{-1,-2,-3,-4,-5,-3,-2,-1} )); }
@Test public void task5_largestAscent_01() { assertEquals( 8, Task5.largestAscent( new int[]{1,2,3,4,2,10} )); } @Test public void task5_largestAscent_02() { assertEquals( 9, Task5.largestAscent( new int[]{1,5,5,10,2,9} )); } @Test public void task5_largestAscent_03() { assertEquals( 0, Task5.largestAscent( new int[]{5} )); } @Test public void task5_largestAscent_04() { assertEquals( 0, Task5.largestAscent( new int[]{5,4,3,2,1} )); } @Test public void task5_largestAscent_05() { assertEquals( 4, Task5.largestAscent( new int[]{1,2,2,3,4,5,4,5,6,7} )); } @Test public void task5_largestAscent_06() { assertEquals( 4050, Task5.largestAscent( new int[]{1,10,100,3000,450,4500} )); } @Test public void task5_largestAscent_07() { assertEquals( 8, Task5.largestAscent( new int[]{-10,-2,-3,1,0,5} )); } @Test public void task5_largestAscent_08() { assertEquals( 100, Task5.largestAscent( new int[]{-100,-50,-75,25,1,60} )); } @Test public void task5_largestAscent_09() { assertEquals( 500, Task5.largestAscent( new int[]{0,500} )); } @Test public void task5_largestAscent_10() { assertEquals( 0, Task5.largestAscent( new int[]{} )); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
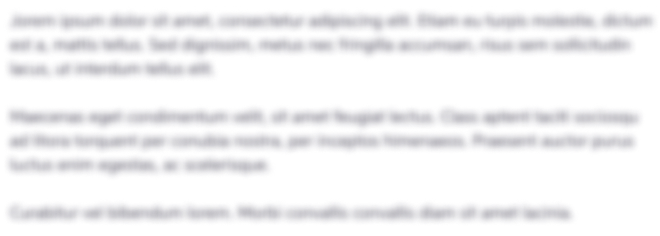
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started