Question
Java Problem: Write a Temperature class that represents temperatures in degrees in both Celsius and Fahrenheit. Use a floating-point number for the temperature and a
Java Problem: Write a Temperature class that represents temperatures in degrees in both Celsius and Fahrenheit. Use a floating-point number for the temperature and a character for the scale: either 'C' for Celsius or 'F' for Fahrenheit. The class should have - Four constructors : one for the number of degrees, one for the scale, one for both the degrees and the scale, and a default constructor . For each of these constructors , assume zero degrees if no value is specified and Celsius if no scale is given . - Two accessor methods : one to return the temperature in degrees Cel- sius, the other to return it in degrees Fahrenheit. Use the formulas from Practice Program 5 of Chapter 3 and round to the nearest tenth of a degree. - Three set methods : one to set the number of degrees, one to set the scale, and one to set both. - Three comparison methods : one to test whether two temperatures are equal , one to test whether one temperature is greater than another, and one to test whether one temperature is less than another. Write a driver program that tests all the methods . Be sure to invoke each of the constructors , to include at least one true and one false case for each comparison method , and to test at least the following three temperature pairs for equality: 0.0 degrees C and 32.0 degrees F, ?40.0 degrees C and ?40.0 degrees F, and 100.0 degrees C and 212.0 degrees F.
SAMPLE RUN #4: java Temperature
Interactive Session Standard Input
Invokingdefaultconstructor:Temperatureobj1; getTempC=0.0 getTempF=32.0 getScale=C Enteratemperaturetocreateanobjectwiththesecondconstructor:23 getTempC=23.0 getTempF=73.4 getScale=C EnteratemperaturespaceForCtocreateanobjectwiththethirdconstructor:72F getTempC=22.2 getTempF=72.0 getScale=F EnteratemperaturespaceForCtocreateafourthobjectwhosemutatorswillbetested:22F HereisthestateofthenewTemperatureobject: getTempC=-5.6 getTempF=22.0 getScale=F executingobj4.setTemp(55.99) Nowtheobj4'sstateis: getTempC=13.3 getTempF=56.0 getScale=F executingobj4.setScale('C') Nowtheobj4'sstateis: getTempC=56.0 getTempF=132.8 getScale=C executingobj4.setTempAndScale(32,'F') Nowtheobj4'sstateis: getTempC=0.0 getTempF=32.0 getScale=F Createdobjects0.0C,20.0C,32.0F,-40.0C,-40.0F,212.0F,100.0Ctotestequalitymethodofobjects. 0.0C<100.0C=true 0.0C<32.0F=false 20.0C>212.0F=false 20.0C>0.0C=true Allofthefollowingequalitytestsusingtheobjectmethodsshouldyield'true' 0.0C=32.0F=true -40.0C=-40.0F=true 100.0C=212.0F=true
My solution:
public class Temperature { private double degrees; private char scale; public Temperature() { degrees = 0.0; scale = 'C'; } public Temperature(double newDegrees) { degrees = newDegrees; scale = 'C'; } public Temperature(char newScale) { degrees = 0.0; scale = newScale; } public Temperature(double newDegrees, char newScale) { degrees = newDegrees; scale = newScale; }
public double getDegreesC () { double val = scale == 'C' ? degrees : 5 * (degrees - 32) / 9; return Math.round (val * 10.0) / 10.0; } public double getDegreesF () { double val = scale == 'F' ? degrees : 9 * (degrees / 5) + 32; return Math.round (val * 10.0) / 10.0; } public void setDegrees(double newDegrees) { degrees = newDegrees; } public void setScale (char newScale) { scale = newScale;; } public void setDegreesAndScale (double newDegrees, char newScale) { degrees = newDegrees; scale = newScale; }
public boolean isEqualTo (Temperature newTemp) { if(scale == 'C') { if(newTemp.scale == 'C') return(degrees == newTemp.degrees); else return(degrees == newTemp.getDegreesC()); } else { if(newTemp.scale == 'F') return(degrees == newTemp.degrees); else return(degrees == newTemp.getDegreesF()); } } public boolean isGreaterThan (Temperature newTemp) { if(scale == 'C') { if(newTemp.scale == 'C') return(degrees > newTemp.degrees); else return(degrees > newTemp.getDegreesC()); } else { if(newTemp.scale == 'F') return(degrees > newTemp.degrees); else return(degrees > newTemp.getDegreesF()); } }
public boolean isLessThan (Temperature newTemp) { if(scale == 'C') { if(newTemp.scale == 'C') return(degrees < newTemp.degrees); else return(degrees < newTemp.getDegreesC()); } else { if(newTemp.scale == 'F') return(degrees < newTemp.degrees); else return(degrees < newTemp.getDegreesF()); } }
public String toString() { return degrees + "\u00b0" + scale; }
}
TemperatureTest.java import java.util.Scanner; public class TemperatureTest { public static void main(String[] args) { System.out.print("Invoking default constructor: Temperature obj1;"); Temperature obj1 = new Temperature(); System.out.println("getTempC = " +obj1.getDegreesC()); System.out.println("getTempF = " +obj1.getDegreesF()); System.out.println("getScale = " +obj1.getScale()); Scanner userChoice = new Scanner (System.in); System.out.print("Enter the temperature to create an object with the second constructor: "); Temperature obj2 = new Temperature(userChoice.nextDouble()); System.out.println("getTempC = " +obj2.getDegreesC()); System.out.println("getTempF = " +obj2.getDegreesF()); System.out.println("getScale = " +obj2.getScale()); System.out.print("Enter the temperature space F or C to create an object with the third constructor: "); Scanner sc = new Scanner (System.in).useDelimiter("\\s");; double temp = sc.nextDouble(); //it will not leave until user enters data. String scale = sc.next(); //we can read specific data. Temperature obj3 = new Temperature(temp, scale.charAt(0)); System.out.println("getTempC = " +obj3.getDegreesC()); System.out.println("getTempF = " +obj3.getDegreesF()); System.out.println("getScale = " +obj3.getScale()); System.out.print("Enter the temperature space F or C to create an object with the fourth object whose mutators will be tested:Here is the state of the new Temperature object: "); Scanner sc1 = new Scanner (System.in).useDelimiter("\\s");; double temp1 = sc1.nextDouble(); //it will not leave until user enters data. String scale = sc1.next(); //we can read specific data. Temperature obj4 = new Temperature(temp1, scale1.charAt(0)); System.out.println("getTempC = " +obj4.getDegreesC()); System.out.println("getTempF = " +obj4.getDegreesF()); System.out.println("getScale = " +obj4.getScale());
Temperature temp1 = new Temperature(0.0, 'C'); Temperature temp2 = new Temperature(32.0, 'F'); Temperature temp3 = new Temperature(-40.0); Temperature temp4 = new Temperature(-40.0, 'F'); Temperature temp5 = new Temperature(20.0, 'C'); Temperature temp6 = new Temperature(100.0, 'C'); Temperature temp7 = new Temperature(212.0); temp3.setScale('C'); temp7.setScale('F'); System.out.print("Created objects 0.0 C, 20.0 C, 32.0 F, -40.0 C, -40.0 F, 212.0 F, 100.0 C to test equality method of objects."); System.out.println("Temperature " + temp1.toString() + "<" + temp6.toString() + "=true" + temp1.isLessThan(temp6)); System.out.println("Temperature " + temp1.toString() + "<" + temp2.toString() + "=false" + temp1.isLessThan(temp2)); System.out.println("Temperature " + temp5.toString() + ">" + temp7.toString() + "=false" + temp5.isGreaterThan(temp7)); System.out.println("Temperature " + temp5.toString() + ">" + temp1.toString() + "=false" + temp5.isGreaterThan(temp1));
System.out.print("All of the following equality tests using the object methods should yield 'true'"); System.out.println("Temperature " + temp1.toString() + "=" + temp2.toString() + "=true" + temp1.isEqualTo(temp2)); System.out.println("Temperature " + temp3.toString() + "=" + temp4.toString() + "=true" + temp3.isEqualTo(temp4)); System.out.println("Temperature " + temp6.toString() + "=" + temp7.toString() + "=true" + temp6.isEqualTo(temp7)); } }
Error message:
COMPILER ERROR MESSAGES
Temperature.java:112: error: class, interface, or enum expected TemperatureTest.java ^ 1 error
Question: How can I correct the code? I feel like I am close. Any ideas?
Thanks!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
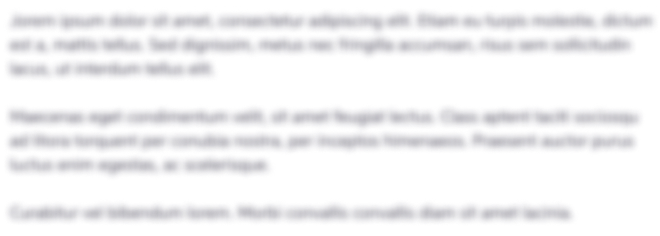
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started