Question
JAVA PROGRAM : Bank Accounts: Using ArrayLists You have been hired as a programmer by a major bank. Your first project is a small banking
JAVA PROGRAM : Bank Accounts: Using ArrayLists
You have been hired as a programmer by a major bank. Your first project is a small banking transaction system. Each account consists of a number and a balance. The user of the program (the teller) can create a new account, as well as perform deposits, withdrawals, balance inquiries, close accounts, etc.(Note: Some additional features have been added for this assignment.)
For this assignment, you must use Classes and move functionality into the classes. Specifically, you should have at least the following classes:
1. A Bank class which consists of an ArrayList of Accounts currently active or closed.
2. An Account class which consists of a Depositor, an account number, an account type, account status (open or closed), account balance, an ArrayList of Transactions performed on the account. (Note: creating an account is considered a transaction.)
3. A Depositor class which has a Name and a social security number.
4. A Name class which consists of first and last names.
5. A DateInfo class which consists of year, month, and dayOfMonth data members.
6. A Transaction class which consists of a transaction type (e.g., create, deposit, withdrawal, balance, etc.), a transaction amount (where applicable), a transaction date, a success indicator, and if unsuccessful, areason for failure.
As before, you must implement appropriate methods in each class so as to implement the functionality required. New methods are to be added as necessary.
The data members of each class must be private. As such, you may need to provide accessor and mutator methods as necessary.
Remember, all I/O should be done only in the methods of the class that contains the main() method.
As in previous assignments, initially, the account information of existing customers is to be read into the database. (Note: the ArrayList of Accounts will grow dynamically as each account is created.) The program keeps tracks of the actual number of currently active accounts. A table of the initial database of active accounts should be printed.
As before, the program then allows the user to select from the following menu of transactions:
Select one of the following:
W - Withdrawal
D - Deposit
C - Clear Check
N - New account (NOTE: the ArrayList of Accounts will grow when you create a new Account)
B - Balance
I - Account Info (without transaction history) (NOTE: include at least one depositor who has multiple accounts)
H - Account Info plus Account Transaction History
S - Close Account (close (shut), but do not delete the account) (Note: no transactions are allowed on a closed account)
R - Reopen a closed account
X - Delete Account (close and delete the account from the database)) (NOTE: must have zero balance to delete)
Q - Quit
Note 1: The Clear Check transaction is only valid for checking accounts. It is like a withdrawal; except, you must also check the date of the check. You may only clear a check if the date on the check is no more than six months ago. No post-dated checks (checks with a future date) may be cleared. Use the DataeInfo class to implement this. In addition, a check will clear only if there is sufficient funds in the account. If the account lacks sufficient funds, the check will not clear and the account will be charged a $2.50 Service Fee for bouncing a check.
Note 2: CD accounts will now contain a Maturity Date. Deposits and Withdrawals will be allowed only on or after the maturity date. When a deposit or withdrawal is made, have the user select a new maturity date fro the CD. the choices are either 6, 12, 18, or 24 months from the date of the deposit or withdrawal. Again, use the DateInfo class to implement this.
Note 3. Use the Calendar class to assist you in implementing the DateInfo class.
Once the user has entered a selection, appropriate methods (in the class that contains the main() method) should be called to perform the specific transaction. These methods will call the class implemented methods as necessary. At the end, before the user quits, the program prints the contents of the final database.
As in previous assignments, make sure to use enough test cases so as to completely test program functionality. Make sure that there is at least one depositor that has multiple accounts at the bank.
Once the user has entered a selection, appropriate methods should be called to perform the specific transaction. At the end, before the user quits, the program prints the contents of the database. You should also print the transaction history for each account in the database.
As in previous assignments, make sure to use enough test cases so as to completely test program functionality
Please refer to the previous assignments as an outline!
Link: Assignment 1
https://www.chegg.com/homework-help/questions-and-answers/hired-programmer-major-bank-first-project-small-banking-transaction-system-account-consist-q34844652?trackid=5RBrq5Bp
Link: Assignment 2
https://www.chegg.com/homework-help/questions-and-answers/java-program-bank-accounts-using-classes-constructors-methods-hired-programmer-major-bank--q35500077?trackid=5RBrq5Bp
Please provide test cases and some of the output as an image, greatly appreciated!
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Minimal Class Requirements:
1a. The Bank class should at least have a default (No-Arg) constructor that would allow statements of the form:
Bank bank = new Bank(); //implements a No-Arg Constructor
1b. The Bank class should have at least have the following methods:
public int openNewAcct(...) (return value indicates success or reason for failure)
public int deleteAcct(...) (return value indicates success or reason for failure)
public int findAcct(...) (return value indicates index of found Account or reason for failure)
public Account getAcct(...) Public int getNumAccts(...) public void setAcct(...)
2a. The Account class should at least have a constructor that would allow statements of the form:
Account account = new Account(...); //implement both a No-Arg and a Parametized Constructor
2b. The Account class should have at least the following methods:
public double getBalance(...)
public int makeDeposit(...) (return value indicates success or reason for failure)
public int makeWithdrawal(...) (return value indicates success or reason for failure)
public int clearCheck(...) (return value indicates success or reason for failure)
public boolean closeAcct() public boolean reopenAcct()
public Depositor getDepositor(...)
public int getAcctNumber(...)
public String getAcctType(...)
public ArrayList getTransactions()
public void setDepositor(...)
public void setAcctNumber(...)
public void setAcctType(...)
public void addTransaction()
3a. The Depositor class should at least have a constructor that would allow statements of the form:
Depositor depositor = new Depositor(...); //implement both a No-Arg and a Parametized Constructor
3b. The Depositor class should at least the following methods:
public Name getName(...)
public String getSSN(...)
public void setName(...)
public void setSSN(...)
4a. The Name class should at least have a constructor that would allow statements of the form:
Name name = new Name(...); //implement both a No-Arg and a Parametized Constructor
4b. The Name class should at least the following methods:
public String getFirstName(...) public String getLastName(...)
public void setFirstName(...)
public void setLastName(...)
5a. The DateInfo class should at least have a constructor that would allow statements of the form:
DateInfo date = new DateInfo(...); //implement both a No-Arg and a Parametized Constructor
5b. The DateInfo class should at least the following methods:
public int compareDate(...) //used to compare two DateInfo objects - returns signal to indicate result of comparison
public int getYear(...)
public int getMonth(...)
public int getDayOfMonth(...)
public void setYear(...)
public void setMonth(...)
public void setDayOfMonth(...)
6a. The Transaction class should at least have a constructor that would allow statements of the form:
Transaction transaction = new Transaction(...); //implement both a No-Arg and a Parametized Constructor
6b. The Transaction class should at least the following methods:
public String getTransactionType(...)
public double getTransactionAmount(...)
public boolean getTransactionSuccessIndicator(...)
public String getTransactionFailureReason(...)
public void setTransactionType(...)
public void setTransactionAmount(...)
public void setTransactionSuccessIndicator(...)
public void setTransactionFailureReason(...)
7. The class containing the main() method should have at least the following methods:
public static void main(String[] args)
public static void readAccts(...)
public static void printAccts(...);
public static void menu(...)
public static void balance(...);
public static void deposit(...);
public static void withdrawal(...);
public static void clearCheck(...);
public static void acctInfo(...);
public static void acctInfoHistory(...);
public static void newAcct(...);
public static void closeAcct(...);
public static void reopenAcct(...);
public static void deleteAcct(...);
8. All I/O should be done only in the methods of the class that contains the main() method
Step by Step Solution
There are 3 Steps involved in it
Step: 1
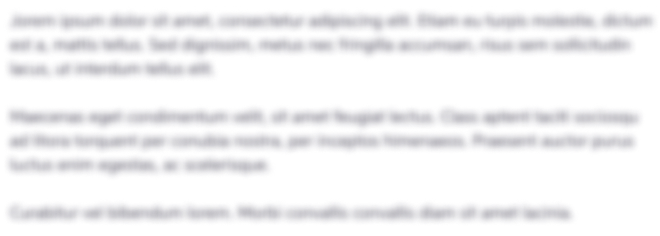
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started