Question
Java program. Follow the instructions below: Create a class named Person Declare 2 String fields named firstName and middleName Declare a boolean field named isMale
Java program.
Follow the instructions below:
Create a class named Person
Declare 2 String fields named firstName and middleName
Declare a boolean field named isMale
Declare an int field named age
Add a constructor with 4 parameters having the header:
public Person(String firstName, String middleName, boolean isMale, int age)
Using this. in front of field variable names, assign firstName and middleName fields equal to the parameters of the same name above, BUT after first removing leading/trailing spaces and making uppercase (HINT: Use external method calls to String .trim( ) and String .toUp- perCase( ) on the parameters before the assignment operation).
InitializetheisMaleandagefieldsbyalsoassigningthemequaltotheparametersofthesame name and by prefixing this. for the fields
Add an overloaded constructor with only 3 parameters and header:
public Person(String firstName, String middleName, boolean isMale) Initialize all fields in the same manner as the previous constructor, EXCEPT now initialize the age
field to 0 (i.e. baby born at age = 0)
Add accessor getFirstName to return the value of firstName field.
Add accessor getMiddleName to return value of middleName field.
Add accessor getAge to return the value of the age field
Add accessorgetGenderString to return a gender String with header: public String getGenderString( )
(a) if the isMale field is true, then return male (b) else return female
Add accessor getInitialsString to return first letter of first middle names: public String getInitialsString( )
Only 1 statement to return the String concatenation (+ operator) of the 1st letter (using String .substring()) of the firstName and middleName fields
Add mutator incrementAge to increment age field by 1 with header: public void incrementAge( ) Only 1 statement using the unary operator ++ on the age field
Add mutator changeFirstName to modify the firstName field with header: public void changeFirstName(String firstName)
if the firstName parameter is not null,
Declare and initialize local variable newName by assigning it equal to the result of the parameter firstName after .trim() and .uppercase()
if newName is NOT empty (using String .isEmpty()) (a) Assign firstName field (using this.) equal to formatted newName (b) Print First name changed to:
else print New first name does not contain any valid characters else print New first name is null
We have now finished the Person class. Next, we will start on the family class.
Create a class named Family.
Declare a String field named surname.
Declare 3 Person fields named husband, wife and child
Add a constructor with 3 parameters having the header:
public Family(String surname, Person husband, Person wife)
Using this. in front of field variable name surname, initialize the field by assigning it equal to the result of the parameter of the same name above, after first removing leading/trailing spaces and making it uppercase (HINT: Use external method calls to String .trim( ) and String .toUpperCase( ) on the parameter before the assignment operation.
Initialize the husband and wife fields by also assigning them equal to the parameters of the same name and by prefixing this. for the fields
Add accessor getSurname to return the String value of surname field.
Add accessors getHusband, getWife, and getChild to return those field values
AddmutatorhaveChildtoaddachildtothefamilywiththeheader:publicvoidhaveChild(Person child)
(a) Print firstName and middleName of the child with is born. at the end. (b) Assign the field child(usingthis.) equal to the input parameter child
Add overloaded haveChild method with 3 parameters and the header: public void haveChild(String firstName, String middleName, boolean isMale)
Define a local variable named baby of type Person
Create a new Person object by calling the Person constructor with the 3 parameters passed in
and assign the result to the baby local variable
Print the firstName and middleName of the baby with is born. at the end.
Assign the field child equal to the new baby
Add mutator haveFamilyBirthday to increase the age of all family members by a year: public void haveFamilyBirthday( )
Use external method calls to .incrementAge() for both the husband and wife
Print Husband (or Wife) is now <#age> years old for each husband and wife object using
external .getAge() call to get the variable for <#age>.
if child is not null (i.e. exists)
Call .incrementAge() for the child and also print a similar output.
Add print method public void printChild( ) to print the child:
if the child field is not null, then print: The
else print There is no child in the
Add print method public void printFamilyInitials( ) for the entire family:
Print the heading The
Print <.getInitialsString> (<.getAge>) for each husband and wife if the child field is not null
Print <.getInitialsString> (<.getAge>) on next line for child.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
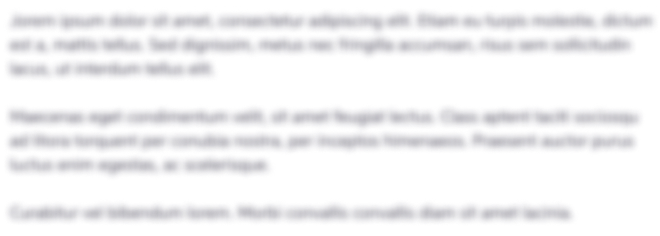
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started