Question
Java Program - GUI help - Create an interface for the user to add, remove, sort, filter, save and load information from our flower pack.
Java Program - GUI help
- Create an interface for the user to add, remove, sort, filter, save and load information from our flower pack. -You should USE ALL of the following at least once in your interface: JFrame JButton JLabel JTextField JCheckBox / JRadioButton Layout (Flow, grid,or border layouts) JMenu JMenuItem
-The flower pack should hold flowers, weeds, fungus, and now herbs. All should be a subclass of a plant class. -Each subclass shares certain qualities (ID, Name, and Color) -Flower traits include (Thorns, and Smell) -Fungus traits include (Poisonous) -Weed traits include (Poisonous, Edible and Medicinal) -Herb traits include (Flavor, Medicinal, Seasonal)
Use the code below:
package flowerpack; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.FileReader; import java.io.FileWriter; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.util.ArrayList; import java.util.Scanner;
public class FlowerPack { public static void main(String[] args) { try{ ArrayList flowerPack= new ArrayList<>(); Scanner input=new Scanner(System.in); int choice; String name, color, ID; boolean poisonous,edible,medicinal,smell,thorns; while(true) { System.out.println(" 1. Add plants"); System.out.println("2. Remove plants"); System.out.println("3. Search plants"); System.out.println("4. Filter plants"); System.out.println("5. Save to file"); System.out.println("6. Load from file"); System.out.println("7. Quit"); System.out.print("Enter your choice: "); choice=input.nextInt(); switch(choice) { case 1: input.nextLine(); System.out.print("You want to add Flower, Fungus or Weed? "); String type=input.nextLine(); if(type.equalsIgnoreCase("Flower")) { System.out.print("Enter name : "); name=input.nextLine(); System.out.print("Enter ID : "); ID=input.nextLine(); System.out.print("Enter color : "); color=input.nextLine(); System.out.print("Has thorns? "); thorns=input.hasNextBoolean(); input.nextLine(); System.out.print("Has smell? "); smell=input.hasNextBoolean(); input.nextLine(); flowerPack.add(new Flower(name,ID,color,thorns,smell)); } else if(type.equalsIgnoreCase("Fungus")) { System.out.print("Enter name : "); name=input.nextLine(); System.out.print("Enter ID : "); ID=input.nextLine(); System.out.print("Enter color : "); color=input.nextLine(); System.out.print("Is it poisonous? "); poisonous=input.hasNextBoolean(); input.nextLine(); flowerPack.add(new Fungus(name,ID,color,poisonous)); } else if(type.equalsIgnoreCase("Weed")) { System.out.print("Enter name : "); name=input.nextLine(); System.out.print("Enter ID : "); ID=input.nextLine(); System.out.print("Enter color : "); color=input.nextLine(); System.out.print("Is it Poisonous? "); poisonous=input.hasNextBoolean(); input.nextLine(); System.out.print("Is it Edible? "); edible=input.hasNextBoolean(); input.nextLine(); System.out.print("Is it Medicinal? "); medicinal=input.hasNextBoolean(); input.nextLine(); flowerPack.add(new Weed(name,ID,color,poisonous,edible,medicinal)); } break; case 2:input.nextLine(); System.out.print("Enter the name of the plant you want to remove : "); name=input.nextLine(); int flag=0; for(Plant plant:flowerPack) { if(plant.getName().equalsIgnoreCase(name)) { System.out.println("plant removed sucessfully") ; flag=1; break; } } if(flag==0) { System.out.println("plant not found") ; } break; case 3: input.nextLine(); System.out.print("Enter the name of the plant you want to search : "); name=input.nextLine(); int f=0; for(Plant plant:flowerPack) { if(plant.getName().equalsIgnoreCase(name)) { System.out.println("plant found sucessfully") ; f=1; break; } } if(f==0) { System.out.println("plant not found") ; } break; case 4: input.nextLine(); System.out.print("Enter the name of the plant you want to filter: "); name=input.nextLine(); f=0; for(Plant plant:flowerPack) { if(plant.getName().equalsIgnoreCase(name)) { System.out.println("Name: " + plant.getName() + "\tID: " + plant.getID()) ; f=1; } } if(f==0) { System.out.println("NO plant of this name in List") ; } break; case 5: File file = new File("Plants.txt"); FileOutputStream fStream = new FileOutputStream(file); ObjectOutputStream stream = new ObjectOutputStream(fStream); stream.writeObject(flowerPack); stream.close(); break; case 6: File file2 = new File("Plants.txt"); FileInputStream fileStream = new FileInputStream(file2); ObjectInputStream oStream = new ObjectInputStream(fileStream); ArrayList readObject = ((ArrayList)oStream.readObject()); flowerPack.addAll(readObject); oStream.close(); break; case 7: System.exit(0); } } }catch(Exception e) { System.out.println(e); } }
}
******* package flowerpack;
import java.io.Serializable;
public class Plant implements Serializable{ String name; String ID; Plant(String name,String ID) { this.name=name; this.ID=ID; } public String getName() { return name; } public String getID() { return ID; }
******** package flowerpack;
class Fungus extends Plant { String Color; boolean Poisonous; public Fungus(String name, String ID,String Color,boolean Poisonous) { super(name, ID); this.Color=Color; this.Poisonous=Poisonous; } } ******* package flowerpack;
class Flower extends Plant { String color; boolean thorns; boolean smell; public Flower(String name, String ID,String color,boolean thorns,boolean smell) { super(name, ID); this.color=color; this.thorns=thorns; this.smell=smell; } } ********* package flowerpack;
class Weed extends Plant { String Color; boolean Poisonous; boolean Edible; boolean Medicinal; public Weed(String name, String ID,String Color,boolean Poisonous,boolean Edible, boolean Medicinal) { super(name, ID); this.Color=Color; this.Poisonous=Poisonous; this.Edible=Edible; this.Medicinal=Medicinal; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
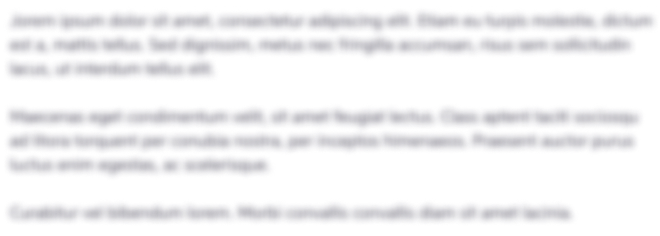
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started