Question
Java program question: (this is a buildup from a previous prommaing assigment but I have the code to the previous one) What I have: import
Java program question: (this is a buildup from a previous prommaing assigment but I have the code to the previous one)
What I have:
import java.util.Random;
public class ArrayBag
{
public static final int DEFAULT_CAPACITY = 10;
private T [] collection;
private int size;
/*
* default constructor * * bag initially set to 10 items */
public ArrayBag()
{
this(DEFAULT_CAPACITY);
}
/*
* argument constructor * size of bag specified by user */
@SuppressWarnings("unchecked")
public ArrayBag(int capacity)
{
if (capacity
{
throw new IllegalArgumentException("bag can't be negative size!");
}
collection = (T []) new Object [capacity];
size = 0;
}
/* * adds item to bag * if at capacity, bag is resized */
public void add (T item)
{
checkForNull(item);
ensureSpace();
collection[size] = item;
size++;
}
/*
* removes item from bag * returns removed item */
public T remove ()
{
if (size == 0)
{
return null;
}
T removed = collection[size-1];
collection[size-1] = null;
size--;
return removed;
}
/* * returns item from bag */
public T get()
{
if (size == 0)
{
return null;
}
return collection[size-1];
}
/* * returns true if item is in bag, false otherwise */
public boolean contains(T item)
{
for (int i = 0; i
{
if (collection[i].equals(item))
{
return true;
}
}
return false;
}
/* * returns size of bag */
public int size()
{
return size;
}
/* * returns string representation of contents of bag */
public String toString()
{
String s = "[";
for (int i = 0; i
{
s+= collection[i];
if (i
{
s+= ", ";
}
}
return s + "]";
}
/* methods to be added */ /* * removes specified item from bag * * returns false if item not present */
public boolean remove (T item)
{
for(int i=0; i { if(item.equals(collection[i])) { for(int j=i; j { collection[j] = collection[j+1]; } collection[size-1] = null; size--; return true; } } return false; } /* * removes random item from bag * returns null if bag is empty */ public T removeRandom () { if(size == 0) return null; T item = collection[new Random().nextInt(size)]; remove(item); return item; } /* * returns random item from bag * returns null if bag is empty */ public T getRandom () { if(size == 0) return null; return collection[new Random().nextInt(size)]; } /* end of added methods */ /* * checks if item is null and throws exception if so */ private void checkForNull (T item) { if (item == null) { throw new IllegalArgumentException("null not a possible value!"); } } /* * doubles size of array if at capacity */ private void ensureSpace() { if (size == collection.length) { @SuppressWarnings("unchecked") T [] larger = (T []) new Object [size * 2]; for (int i = 0; i { larger[i] = collection[i]; } collection = larger; larger = null; System.out.println("Hi"); } } } public interface Bag boolean remove (T item); T removeRandom(); T getRandom(); } public class Bags { public static void main (String [] args) { try { Bag // seeing if bag contains item boolean result = words.contains("kiwi"); System.out.println(" Does our bag contain the word 'kiwi'? " + (result ? "yes" : "no")); result = words.contains("mango"); System.out.println("Does our bag contain the word 'mango'? " + (result ? "yes" : "no")); // retrieving item System.out.println(" Selecting an item (always same)"); System.out.println(words.get()); System.out.println(words.get()); // retrieving random item System.out.println(" Selecting a random item (varies)"); System.out.println(words.getRandom()); System.out.println(words.getRandom()); // removing specific item words.remove("grape"); System.out.println(" Removing 'grape' from the bag " + words); // removing item System.out.println(" Removing an item (always end one)"); System.out.println(words.remove()); System.out.println(words); // removing random item System.out.println(" Removing a random item"); System.out.println(words.removeRandom()); System.out.println(words + " "); // testing methods on empty bag System.out.println("Let's empty the bag"); words.remove(); words.remove(); System.out.println(words); System.out.println("Trying to get a random item " + words.getRandom()); System.out.println("Trying to remove a random item " + words.removeRandom()); System.out.println("Trying to remove 'kiwi' " + words.remove("kiwi")); } catch (IllegalArgumentException e) { System.out.println(e.getMessage()); } } } public class LinkedBag
/* * WordGame * * beginnings of a Scrabble-like word game * * adds the letter tiles from Scrabble to a bag and randomly selects * seven for the user's hand */ public class WordGame { // number of letters in player's hand in Scrabble public static final int LETTERS_PER_TURN = 7; // distribution of Scrabble tiles public static final char [][] ENGLISH_LETTERS = {{'1', 'K', 'J', 'X', 'Q', 'Z'}, {'2', 'B', 'C', 'M', 'P', 'F', 'H', 'V', 'W', 'Y'}, {'3', 'G'}, {'4', 'L', 'S', 'U', 'D'}, {'6', 'N', 'R', 'T'}, {'8', 'O'}, {'9', 'A', 'I'}, {'0', 'E'}}; /* * adds letter tiles to bag according to their distribution */ public static void addLetters(Bag
Implement the three methods you wrote for ArrayBag for LinkedBag: boolean remove (T item); T removeRandomO; T getRandomO Then test them using Bags.java. The output will be similar to that for the ArrayBag (but with the order reversed since operations for the linked bag are performed at the front): Here's what's in our bag [lime, coconut, kiwi, grape, orange] Does our bag contain the word 'kiwi'? yes Does our bag contain the word 'mango'? no Selecting an item (always same) lime lime Selecting a random item (varies) coconut grape Removing grape' from the bag [coconut, kiwi, lime, orange] Removing an item (always front one) coconut [kiwi, lime, orange] Removing a random item kiwi [lime, orange]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
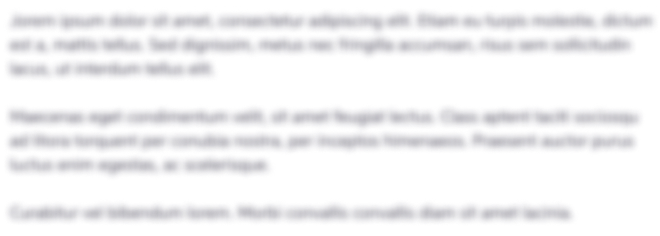
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started