Question
Java Program(do the Todos in the solarsystem class), the Planet calss is at the bottom ---------------------------------------------------------------------------------------- import java.util.Comparator; import javax.swing.JPanel; import java.awt.Color; import java.awt.FontMetrics; import
Java Program(do the Todos in the solarsystem class), the Planet calss is at the bottom ---------------------------------------------------------------------------------------- import java.util.Comparator; import javax.swing.JPanel; import java.awt.Color; import java.awt.FontMetrics; import java.awt.Graphics; import junit.framework.TestCase; /** * This Solar System is implemented as a doubly linked list. * Planets may be added to or removed from a Solar System. * They may also be sorted by their distance from their sun in AU, * the length of their day in hours, or their number of moons. * * @author scannell * */ public class SolarSystem { private static class Node{ Planet planet; Node before, after; /** *@param p, a planet, must not be null */ Node(Planet p, Node b, Node n){ planet = p; before = b; after = n; } } private Node head, tail; public SolarSystem() { head = tail = null; } /** * Add a planet to the end of the list * * @param p, planet to be added */ public void add(Planet p) { if(head == null) tail = head = new Node(p, null, null); else tail = tail.after = new Node(p, tail, tail.after); } /** * Remove a planet from the Solar System * * @param p, planet to be removed * @return true if the removal occurred, or false otherwise */ public boolean remove(Planet p) { if(p == null || head == null) return false; //TODO //Think of the three cases //1. If the element is at the head //2. If the element is at the tail //3. If the element is anywhere else return true; } private Node getNode(Planet p) { for(Node n = head; n != null; n = n.after) if(n.planet.equals(p)) return n; return null; } /** * Sort using the given comparator * * @param comparator */ public void sort(Comparator comparator) { if(head == null || head.after == null) return; bubbleSort(comparator); } /** * Using bubbleSort, sort the elements by the given comparator * * @precondition assume that there is at least one element * and therefore head is not null * * @param comparator describes how elements should be ordered * Note: Comparator usage * comparator.compare(t1,t2) returns an integer * if t1 comes before t2, returns a negative number * if t1 comes after t2, returns a positive number * if t1 and t2 are interchangeable, returns 0 */ private void bubbleSort(Comparator comparator) { boolean swapped = false; Node current = head; while(current.after != null) { //TODO //compare current and the after element //if they are out of order then swap } if(swapped) bubbleSort(comparator); } /** * Swaps the given current node with the following node * * @param current, current node to swap with current.after */ private void swap(Node current) { Node after = current.after; if (after.after != null) after.after.before = current; if (current.before != null) current.before.after = after; current.after = after.after; after.before = current.before; after.after = current; current.before = after; if (current == head) head = after; if (after == tail) tail = current; } /** * Sorts the planets by their distance from their sun in AU * Note: 1 AU (astronomical unit) is the distance from the Earth to the Sun */ public void sortByDistance() { sort(SolarSystem.distanceComparator()); } private static Comparator distanceComparator() { return new Comparator() { @Override public int compare(Planet p1, Planet p2) { return (int) Math.round(p1.getDistance()*10-p2.getDistance()*10); } }; } /** * Sorts the planets by the length of their day in Earth hours */ public void sortByDayLength() { // TODO // Sort using a comparator set to an anonymous class implementing // the interface Comparator(). You may use the // sortByDistance() as an example, but compare by the // length of the planet's day. // You should use the dayLengthComparator() method to // create the anonymous comparator. } private static Comparator dayLengthComparator(){ return new Comparator() { // TODO // Make this comparator actually compare these planets by // their day length }; } /** * Sorts the planets by their number of moons */ public void sortByNumMoons() { sort(SolarSystem.numMoonsComparator()); } private static Comparator numMoonsComparator() { return new Comparator() { @Override public int compare(Planet p1, Planet p2) { return (int) Math.round(p1.getMoons() - p2.getMoons()); } }; } /** * Creates a canvas to reflect the state of the Solar System * @return */ public JPanel makeCanvas() { return new SolarSystemCanvas(); } private class SolarSystemCanvas extends JPanel { private static final long serialVersionUID = -2226594746308463675L; private static final int BOX_WIDTH = 200, BOX_HEIGHT = 130, SECTION_HEIGHT = 20; private static final int X_SPACE = 30; private static final int Y = 10; private static final int ARROWSIZE = 5; @Override public void paintComponent(Graphics g) { super.paintComponent(g); Node cursor = head; int x = 10; while (cursor != null) { paintBox(g,x,cursor); x+= BOX_WIDTH + X_SPACE; cursor = cursor.after; } } private void paintBox(Graphics g, int x, Node cursor) { paintRectangle(g,x,null); paintText(g, x, Y+ SECTION_HEIGHT - 5, cursor.planet.getName() ); paintText(g, x, Y + SECTION_HEIGHT * 2 - 5, "Distance From Sun (AU): " + cursor.planet.getDistance()); paintText(g, x, Y + SECTION_HEIGHT * 3 - 5, "Day Length (hr): " + cursor.planet.getDayInHours()); paintText(g, x, Y + SECTION_HEIGHT * 4 - 5, "Number of Moons: " + cursor.planet.getMoons()); if (cursor.before != null) paintPrevPointer(g, x); if (cursor.after != null) paintNextPointer(g, x); } private void paintRectangle(Graphics g, int x, Color color) { if (color != null) { Color previous = g.getColor(); g.setColor(color); g.fillRect(x, Y, BOX_WIDTH, BOX_HEIGHT); g.setColor(previous); } g.drawRect(x, Y, BOX_WIDTH, BOX_HEIGHT); for (int y2 = SECTION_HEIGHT; y2 < BOX_HEIGHT; y2 += SECTION_HEIGHT) { g.drawLine(x, Y + y2, x + BOX_WIDTH, Y + y2); } } private void paintText(Graphics g, int x, int y, String text ) { FontMetrics fm = getFontMetrics( getFont() ); int textWidth = fm.stringWidth(text); if (textWidth > BOX_WIDTH) { int textX = x + (BOX_WIDTH - 10) / 2; g.drawString("...", textX, y ); } else { int textX = x + (BOX_WIDTH - textWidth) / 2; g.drawString(text, textX, y ); } } private void paintNextPointer(Graphics g, int x) { int startX = x+BOX_WIDTH/2; int startY = Y+BOX_HEIGHT-SECTION_HEIGHT/2-SECTION_HEIGHT/3; int endX = x+BOX_WIDTH+X_SPACE; int [] pointx = { endX, endX - ARROWSIZE, endX -ARROWSIZE}; int [] pointy = { startY, startY + ARROWSIZE, startY - ARROWSIZE}; g.drawLine(startX, startY, endX, startY); g.fillPolygon(pointx, pointy, pointx.length); } private void paintPrevPointer(Graphics g, int x) { int startX = x-X_SPACE; int startY = Y+BOX_HEIGHT-SECTION_HEIGHT-SECTION_HEIGHT/2-SECTION_HEIGHT/3; int endX = x+BOX_WIDTH/2; int [] pointx = { startX, startX + ARROWSIZE, startX + ARROWSIZE}; int [] pointy = { startY, startY + ARROWSIZE, startY - ARROWSIZE}; g.drawLine(startX, startY, endX, startY); g.fillPolygon(pointx, pointy, pointx.length); } } public static class TestSolarSystem extends TestCase{ private static Planet mercury = new Planet("Mercury", 0.39, 4222.6, 0); private static Planet venus = new Planet("Venus", 0.723, 2802.0, 0); private static Planet earth = new Planet("Earth", 1, 24, 1); private static Planet mars = new Planet("Mars", 1.524, 24.7, 2); private static Planet jupiter = new Planet("Jupiter", 5.203, 9.9, 79); private static Planet saturn = new Planet("Saturn", 9.539, 10.7, 62); private static Planet uranus = new Planet("Uranus", 19.18, 17.2, 27); private static Planet neptune = new Planet("Neptune", 30.06, 16.1, 14); private static Planet pluto = new Planet("Pluto", 39.53, 153.3, 5); private SolarSystem system; public void setUp() { system = new SolarSystem(); system.add(mercury); system.add(venus); system.add(earth); system.add(mars); system.add(jupiter); system.add(saturn); system.add(uranus); system.add(neptune); } ----------------------------------------------------------- The planet class ------------------------------------------------------------ /** * A planet with immutable fields: * name: String representing name of planet, cannot be null * distanceFromSun: planet's distance from its sun in AU, must be positive * dayLength: length of planet's day in Earth hours, must be positive * numberOfMoons: number of moons orbiting planet, must be non-negative * * @author scannell * */ public class Planet { private final String name; private final double distanceFromSun; private final double dayLength; private final int numberOfMoons; public Planet(String n, double d, double l, int num) { if(n == null || d <= 0 || l <= 0 || num < 0) throw new IllegalArgumentException("Invalid arguments"); name = n; distanceFromSun = d; dayLength = l; numberOfMoons = num; } public String getName() { return name; } public double getDistance() { return distanceFromSun; } public double getDayInHours() { return dayLength; } public int getMoons() { return numberOfMoons; } @Override public boolean equals(Object o) { if(o == null) return false; if(!(o instanceof Planet)) return false; Planet p = (Planet) o; return p.name.equals(this.name) && p.distanceFromSun == this.distanceFromSun && p.dayLength == this.dayLength && p.numberOfMoons == this.numberOfMoons; } } -----------------------------------------------------------
What to do:
1.)You need to implement the remove method inSolarSystem.java. A planet may be removed because it is demoted and no longer classified as a planet, or because it is destroyed by the Death Star or some other catastrophe. A correct implementation of remove should allow the node containing the planet argument to be removed, and neighboring nodes adjusted as needed. This means traversal after a removal should be intact, i.e. if removing the middle node, you should be able to traverse from the first to the last element and back. After removal any head and tail pointers should be updated appropriately. You will need to handleall removal cases.
2.)In thesortByDayLengthmethod, create an anonymous class that implementsComparatorthat compareplanets by the length of its day in hours. We make use of a private helper method to create the anonymouscomparator. We will sort them in ascending order.
3.)We have provided the majority of thebubbleSortmethod. You need to implement the comparison and utilize our swap methodthat takes in the current node to be swapped.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
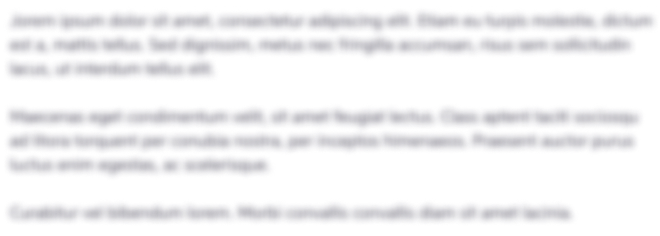
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started