Question
JAVA programming Extend the Number class and implement the Comparable interface to introduce new functionality to your Rational class. The class definition should look like
JAVA programming
Extend the Number class and implement the Comparable interface to introduce new functionality to your Rational class. The class definition should look like this:
public class Rational extends Number implements Comparable{ // code goes here }
In addition a new private method gcd should be defined to ensure that the number is always represented in the lowest terms.
In addition you must override the equals method to allow for equality testing on Rational numbers.
Here is the Main class that I will use to test your Rational classes.
=======================================================================================================================================
=======================================================================================================================================
Here is teacher's Test Main class
public class Main { public static void main(String[] args) { Rational a = new Rational(2, 4); Rational b = new Rational(2, 6); System.out.println(a + " + " + b + " = " + a.add(b)); System.out.println(a + " - " + b + " = " + a.sub(b)); System.out.println(a + " * " + b + " = " + a.mul(b)); System.out.println(a + " / " + b + " = " + a.div(b)); System.out.println(a + " = " + a + " is " + a.equals(new Rational(1, 2))); System.out.println(a + " = " + b + " is " + a.equals(new Rational(1, 3))); Rational[] arr = {new Rational(7, 1), new Rational(6, 1), new Rational(5, 1), new Rational(4, 1), new Rational(3, 1), new Rational(2, 1), new Rational(1, 1), new Rational(1, 2), new Rational(1, 3), new Rational(1, 4), new Rational(1, 5), new Rational(1, 6), new Rational(1, 7), new Rational(1, 8), new Rational(1, 9), new Rational(0, 1)}; selectSort(arr); for (Rational r : arr) { System.out.println(r); } Number n = new Rational(3, 2); System.out.println(n.doubleValue()); System.out.println(n.floatValue()); System.out.println(n.intValue()); System.out.println(n.longValue()); } public static> void selectSort(T[] array) { T temp; int mini; for (int i = 0; i < array.length - 1; ++i) { mini = i; for (int j = i + 1; j < array.length; ++j) { if (array[j].compareTo(array[mini]) < 0) { mini = j; } } if (i != mini) { temp = array[i]; array[i] = array[mini]; array[mini] = temp; } } } }
=======================================================================================================================================
=======================================================================================================================================
Here is my old Rational class
public class Rational {
static int num1;
static int den1;
static int num2;
static int den2;
public static void Rational1(int a,int b) {
if (b == 0) {
System.out.println("denominator is zero, the result is not correct, please rerun the program and enter a non-zero integer!");
}
num1 = a;
den1 = b;
}
public static void Rational2(int a,int b) {
if (b == 0) {
System.out.println("denominator is zero, the result is not correct, please rerun the program and enter a non-zero integer!");
}
num2 = a;
den2 = b;
}
public static void add() {
int resultNum =num1*den2 + den1*num2;
int resultDen =den1*den2;
System.out.println(resultNum+"/"+resultDen);
}
public static void sub() {
int resultNum =num1*den2 - den1*num2;
int resultDen =den1*den2;
System.out.println(resultNum+"/"+resultDen);
}
public static void mul() {
int resultNum =num1*num2;
int resultDen =den1*den2;
System.out.println(resultNum+"/"+resultDen);
}
public static void div() {
int resultNum =num1*den2;
int resultDen =den1*num2;
System.out.println(resultNum+"/"+resultDen);
}
public static void main(String[] args) {
Rational a = new Rational();
Rational b = new Rational();
Rational.Rational1(2,3);
Rational.Rational2(3,4);
System.out.print(Rational.num1 + "/" + Rational.den1 + "+" + Rational.num2 + "/" + Rational.den2 + " = ");
Rational.add();
System.out.println();
System.out.print(Rational.num1 + "/" + Rational.den1 + "-" + Rational.num2 + "/" + Rational.den2 + " = ");
Rational.sub();
System.out.println();
System.out.print(Rational.num1 + "/" + Rational.den1 + "*" + Rational.num2 + "/" + Rational.den2 + " = ");
Rational.mul();
System.out.println();
System.out.print(Rational.num1 + "/" + Rational.den1 + "/" + Rational.num2 + "/" + Rational.den2 + " = ");
Rational.div();
System.out.println();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
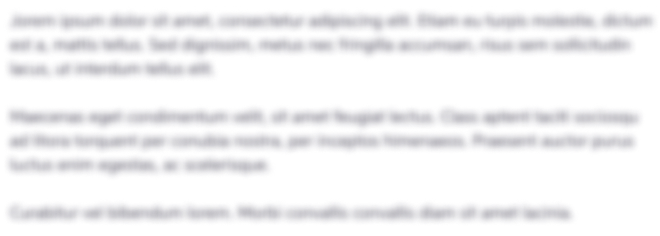
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started