Question
Java Programming In this part, you will define a (simplistic) Dog class that a client program uses to interact with Dog objects. Here are the
Java Programming
-
In this part, you will define a (simplistic) Dog class that a client program uses to interact with Dog objects. Here are the detailed instructions: DogClass.pdf
- Make sure that the client program DogMain.java works well with your class.Place Dog.java file in the same folder as DogMain.java.
Here's a sample run of the client program: sample_dogmain_output.txt.
Submit only Dog.java file for this part.
Warning: You may find solution to this problem online but you must WRITE YOUR OWN CODE. Look at all the example you please, then write your own code. Don't forget // comments, indents, etc: Program Submission Requirements In addition, make sure the Class (data structure) Submission Requirements
are met as well.
in syntheses :
-
In this part, you will define a (simplistic) Dog class that a client program uses to interact with Dog objects. Here are the detailed instructions: DogClass.pdf
Actions
Make sure that the client program DogMain.java works well with your class. Place Dog.java file in the same folder as DogMain.java. Here's a sample run of the client program: sample_dogmain_output.txt. Submit only Dog.java file for this part.
Warning: You may find solution to this problem online but you must WRITE YOUR OWN CODE. Look at all the example you please, then write your own code. Don't forget // comments, indents, etc: Program Submission Requirements In addition, make sure the Class (data structure) Submission Requirements are met as well.
DogClass.pdf (page 1 of 3) @ Q Search Programming Assignment: Dog class and its client code Copyright Statement: Tanuja Joshi, 2021 This assignment is protected by copyright. I am the exclusive owner of copyright in the materials I create Problem Statement: In this assignment you will write a simple Dog class that a DogMain client program can use to interact with a Dog. Specifically, o . 1. Create a simplistic) Dog class with the following requirements: Private fields: o name: name of the dog as a String o color: color of the dog as a String o is Hungry: tells if the dog is hungry as a boolean weight of the dog in KGs as a double Two constructors: O A default constructor: This sets the name field to "Tommy", color field to "Brown", weight to 10 kg. It initializes is Hungry to true. O A constructor with three arguments: name, color and weight. It initializes the three corresponding fields and sets is Hungry to true. o You should avoid code duplication and call one constructor from the other. Methods o Accessors: standard accessors for all the private fields: getName, getColor, get Weight and getIsHungry. setName: a mutator for the name field. o bark: a method that takes no parameters and prints a string of the form "name: Woof Woof" where name is the name of the dog. eat: a method that takes no parameters. This method sets the isHungry field to false and adds 100gms to the weight of the dog. It then prints a string of the form "name: Chomp Chomp". walk: a method that takes no parameters. If the dog is hungry, it just calls the bark() method, otherwise it decreases the weight of the dog by 100gms, sets the is Hungry field to true and prints a string of the form "name: Step Step" where name is the name of the dog. o toString: a method that is called by Java to print the object. This method returns a string of the form: "name: color in color, weighs weight kg and is hungry" when the is Hungry is true or "name: color in color, weighs weight kg and is not hungry" when is Hungry is false. Example: "Willie: Brown in color, weighs 10.2 kg and is not hungry." o o DogClass.pdf (page 2 of 3) Q Search 2. Make sure that attached DogMain.java file containing a client for the Dog class works as expected. This client instantiates two instances of the Dog class and allows user to interact with them. See the output of a sample run shown below: (also found in attached sample_dogmain_output.txt file). Text marked in orange is user input. Enter the command "S' to get status, Il to feed, 'W' to walk, "O' to quit: s Ginger: brown in color weighs 10.0 kgs and is hungry. Enter the command 'S' to get status, I to feed, *w to walk, "O' to quit: 9 ----GRASP exec: java DogMain Enter Dog Choice: 1 for Ginger 2 for Pepper (0 to quit) 2 Enter Dog Choice: 1 for Ginger 2 for Pepper 10 to quit) 0 Good bye! Woof woof 'O' to quit: 5 ----GRASP: operation complete. Enter the command "S' to get status, i to feed "W' to walk, ******* Pepper: Black in color weighs 15.0 kgs and is hungry ************************** Enter the command 'S' to get status, F! to feed 'W' to walk, "O' to quit: w Submit the class file Dog.java only. Make sure to add a block-comment at the start of the file that lists assignment title, class name, date, your name, and assignment description. Follow naming conventions. Pepper: Woof Woof Enter the command 'S' to get status, E' to feed 'W' to walk, 'O' to quit: f Pepper: Chomp Chomp Enter the command "S' to get Status, Ft to feed 'W' to walk, "O' to quit: s Pepper: Black in color weighs 15.1 kgs and is not hungry. Enter the command 's' to get status, Ft to feed 'W' to walk, 'O' to quit: w Pepper: Step Step Enter the command "S' to get status, i to feed, W to walk, "O' to quit: s Pepper: Black in color weighs 15.0 kgs and is hungry. Enter the command "S' to get Status, '' to feed, 'W' to walk, 'Q' to quit: Pepper: Chomp Chomp Enter the command 's' to get status, E' to feed W' to walk, 'O' to quit: f Pepper: Chomp Chomp Enter the command 'S' to get status, I to feed W to walk, "O' to quit: s Pepper: Black in color weighs 15.2 kgs and is not hungry. Enter the command "S to get Status 'F' to feed, W to walk, 'Q' to quit: 9 Enter Dog Choice: 1 for Ginger 2 for Pepper 10 to quit) 1 Dog Main.java import java.util.*; T/Client program for the Dog class 1/ Instantiates two Dog objects and helps user interact with them. public class DogMain { public static void main(String[] args) { Dog dog1 = new Dog(); dog1.setName("Ginger"); Dog dog2 = new Dog("Pepper", "Black", 15); String sep = "******************** *****"; Scanner console = new Scanner(System.in); int choice = -1; Dog dogof Choice; // Prompt the user for the choice of the dog System.out.print("Enter Dog Choice: 1 for "+dog1.getName() + " 2 for "+dog2.getName() + " (0 to quit) "); choice = console.nextInt(); while ( choice != 0) { dogof Choice = choice == 1 ? dog1 : dog2; System.out.println(sep); char cmd = 't'; while (cmd != ''){ // Prompt the user for the command System.out.print("Enter the command 's' to get Status,It'F' to feed, It'W' to walk, It'o' to quit: "); cmd = console.next().toLowerCase().charAt(0); System.out.println(sep); // Take appropriate action switch(cmd) { case 's': System.out.println(dogOfChoice); break; case 'f': dogOfChoice.eat(); break; case 'w': dogofChoice.walk(); break; case 'q': break; default: System.out.println("Invalid command"); } System.out.println(sep); } System.out.print("Enter Dog Choice: 1 for "+ dog1.getName() + " 2 for "+dog2.getName() + " (0 to quit) "); choice = console.nextInt(); } System.out. Rrintin("Good bye! Woof woof"); RRRRR sample_dogmain_output.txt ----GRASP exec: java Dogma in Enter Dog Choice: i for Ginger 2 for Pepper (0 to quit) 2 RICERCA Enter the command 'S' to get status, 'F' to feed, 'W' to walk, "O' to quit: s Pepper: Black in color weighs 15.0 kgs and is hungry. ************** ROCK ROCK ROCK Enter the command "S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit: W ******************* Pepper: Woof Woof **************** *************************************** Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit: f ************************************ Pepper: Chomp Chomp Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit : s ************************************************************** Pepper: Black in color weighs 15.1 kgs and is not hungry. Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit: w **************** CRICKER ******* Pepper: Step Step **************** ********* Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit: s ************************************************************* Pepper: Black in color weighs 15.0 kgs and is hungry. *********** Enter the command "S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit:f Pepper: Chomp Chomp ********* Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit: f Pepper: Chomp Chomp ********* Enter the command "S' to get status, 'F' to feed, 'W' to walk, "Q' to quit: s ** KCR Pepper: Black in color weighs 15.2 kgs and is not hungry. KIRKKOK* Enter the command 'S' to get status, 'F' to feed, 'W' to walk, "Q' to quit: 4 KORRIKO ******************************** Enter Dog Choice: 1 for Ginger 2 for Pepper (0 to quit) 1 ********* Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit: s KORRIKKER CRACKER Ginger: brown in color weighs 10.0 kgs and is hungry. ************************************************************ Enter the command 'S' to get status, 'F' to feed, 'W' to walk, 'Q' to quit : 9 ******************** ***** ************************************* Enter Dog Choice: 1 for Ginger 2 for Pepper (0 to quit) Good bye! Woof woof ----GRASP: operation complete. Program Submission Requirements AV These are the requirements for submitting a Java program in this course: 1. The file name matches the Java Class name which starts with an uppercase character. (Note: When you submit the file in Canvas, it is possible that Canvas renames the file by adding a number to your file name. This is unavoidable and you need not worry about it. I will be able to handle it when grading.) 2. The program compiles with one of the Oracle Java compilers (javac.exe) using JDK 8 or later. 3. Import only java. and javax. classes, or other classes specifically covered in the textbook. 4. Never submit specialized IDE-specific code (e.g., from NetBeans) as the instructor might run the program from the command line. 5. Never submit //TODO lines that your IDE (e.g., Eclipse) generates and you failed to do." 6. Put Your name, course, date, and reasons for doing this as comments at the top of the file (/* comments */). This should probably span multiple lines, any style of /** or /* or // is acceptable. Example: /* Your name, CS 210, mm/dd/yyyy, Reasons for doing this */ 7. Using // style, add additional comments to every screen and at the top of every method you write. 8. Properly indent code for Classes, methods, loops, decisions, etc. as described in the textbook, increasing indentation for each set of braces {} meaning only the Class() is on the far left. 9. Use proper naming conventions for objects using reasonably descriptive names. Classes always start with upper case character (like String) while object identifiers always start lower case (line args). ALL CODE FILES you submit MUST follow the nine guidelines listed above, each is crucial!!! Points will be deducted for not meeting the program submission requirements. A grade of ZERO is automatic if something like your name is not even in the code. Class (data structure) Submission Requirements a Whenever you submit a Java data structure (Class) in this course, you need to follow: 1. The Program Submission Requirements (comments, indents, etc.). 2. Data fields listed at the top of the Class, and they are all private. 3. Fields are not initialized in these private declarations (Java defaults prevail). 4. The only exception to requirements 1-3 above are public static final constants, which never change. 5. Constructors (that's plural) follow the data field specifications, and those initialize all the fields. 6. Always have a default constructor that defines the assumed defaults for all fields (verify if needed). 7. There should be no redundant constructor code; so the multiple argument version is used for others with this() calls. 8. There should be no redundant method code; so a detailed math formula is in one place, then used by others. 9. Accessor methods are grouped together. Mutators are grouped together. There should be no random placements in Class. 10. System.out calls (and other extraneous TODO garbage) is removed. A data structure is a data structure, not a program!!! Remember to cite your references, or better yet, write your own code to avoid plagiarism. See the Student Code of Conduct and Academic Integrity section of the course syllabusStep by Step Solution
There are 3 Steps involved in it
Step: 1
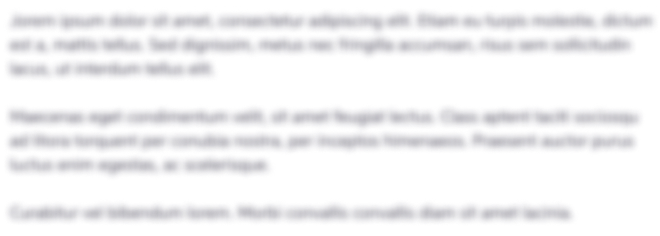
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started