Question
Java programming question Creating a tic tac toe java program. I was provided with some pre-written code that could be useful for creating the program.
Java programming question
Creating a tic tac toe java program.
I was provided with some pre-written code that could be useful for creating the program.
Really sorry for the long post. The highlighted sentences are the part that I need help with.
Below is the pasted the code for Board.java
public class Board {
public GameState getGameState() { GameState current = GameState.ONGOING; current = checkHorizontalWin(); if (current != GameState.ONGOING) { return current; } current = checkVerticalWin(); if (current != GameState.ONGOING) { return current; } current = checkDiagonalWin(); if (current != GameState.ONGOING) { return current; } if (checkTie()) { return GameState.TIE; } return GameState.ONGOING; }
private GameState checkHorizontalWin() { for (int row = 0; row
private GameState checkVerticalWin() { for (int i = 0; i
private GameState checkDiagonalWin() { char boardMiddle = board[1][1]; boolean diagnolOne = board[0][0] == boardMiddle && boardMiddle == board[2][2]; boolean diagnolTwo = board[0][2] == boardMiddle && boardMiddle == board[2][0]; if (diagnolOne || diagnolTwo) { if (boardMiddle == 'X') { return GameState.PLAYER1_WIN; } else if (boardMiddle == 'O') { return GameState.PLAYER2_WIN; } } return GameState.ONGOING; }
private boolean checkTie() { for (int i = 0; i
public String toString() { String boardString = ""; for (int i = 0; i Rules 1. The game is played on a 3x3 grid. 2. Player 1 is the letter X, and Player 2 is the letter 0. Players take turns putting their marks in empty squares. 3. The first player to get 3 marks in a row (vertically across any column, horizontally along any row, or diagonally across any diagonal) is the winner. 4. When all 9 squares are full, the game is over. If no player has won, the game ends in a tie. GameState.java Provided Java file. It is an enum representing the game's state - a win for Player 1, a win for Player 2, a tie, or an ongoing game. Do not modify this file. 1 2 3 public enum GameState { PLAYER1 WIN, PLAYER2_WIN, ONGOING, TIE; - Location.java This Java file represents a given location on the board. It has two instance variables: row and column. For example, a Location object where the row is 2 and the column is 2 would represent the square at the bottom right of a Tic-Tac-Toe board. Because they are private, we cannot access them from outside the Location class. Therefore, implement the following getter methods in Location.java. Make these methods public. a getter for row, properly named (ie, getRow) a getter for column, properly named (ie, getColumn) public class Location { private int row; private int column; Soco van WN public Location(int row, int column) { this.row = row; this.column = column; Il getter for row in this place Il getter for column in this place 12 13 } Board.java This Java file represents our Tic-Tac-Toe board. Create a 2D character array called board to hold the current state of the Tic-Tac-Toe board. This board should be 3x3 and be an instance variable. When the game begins, the board should only hold empty spots. An empty spot is represented with a space character (' '). As players place their tokens, it should begin to fill with Xs and Os. Player 1 is X and Player 2 is 0. You will implement the following public methods for Board. a method that takes in a location and returns a boolean. True if the location is valid (ie, on the board) and the location is an empty spot. False if the location is invalid or non-empty. An example of an invalid location would be the spot 40,2 because there is no row 40 in our 3x3 grid. a method that takes in a location and a letter, and returns a boolean This method places the letter (which should be an X or 0), in the specified location. This should only happen if the location is valid. - Return true if placing the letter is successful, false if not We have provided the following public methods for Board. the getGameState method - Returns a GameState representing the current state of the game Either there was a winner, a tie, or it's still ongoing 1 public class Board { public GameState getGameState() { GameState current = GameState.ONGOING; current = checkHorizontalwin ; if (current != GameState.ONGOING) { return current; current = checkVerticalWin(); if (current != GameState.ONGOING) { return current; No BBURHEBEO von Awn current = checkDiagonalWin(); if (current != GameState.ONGOING) { return current; if (checkTie()) { return GameState.TIE; return GameState.ONGOING; private GameState checkHorizontalwin() { for (int row = 0; row
Step by Step Solution
There are 3 Steps involved in it
Step: 1
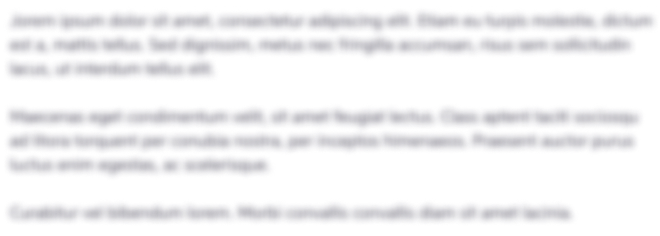
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started