Question
JAVA Programming The second argument to the constructors is the ordering key. For Dog use the positive value, for Cat use the negative value. This
JAVA Programming
The second argument to the constructors is the ordering key. For Dog use the positive value, for Cat use the negative value. This defines your compareTo()
I'm struggling with adding a compareTo() into dog and cat, dog needs to return 1 and cat needs to return -1
the orderingKey defines the compareTo()
This is the Desired output:
ABSOLUTELY DO NOT MODIFY THE AssignmentTwo Class, this class must be used as is
AssignmentTwo:
import java.util.ArrayList; import java.util.Collections; import java.util.List;
public class AssignmentTwo {
public static void main(String[] args) { Cat cat1 = new Cat("Fluffy", 150); Cat cat2 = new Cat("Sweety", 200, "yellow"); Dog dog1 = new Dog("Duke", 10, 500); Dog dog2 = new Dog("Jason", 5); List
}
Animal:
public abstract class Animal implements Storable, Talkable {
String name;
int orderingKey; public int getKey() { return orderingKey; }
Animal(String name, int orderingKey) {
this.name = name;
this.orderingKey = orderingKey;
} }
Dog:
class Dog extends Animal implements Talkable, Storable { double weight; Dog(String name, int orderingKey) { super(name, orderingKey); this.weight = 100.0; } Dog(String name, int orderingKey, float weight) { super(name, orderingKey); this.weight = weight; }
public String getType() { return "Doggie"; }
public String speaksBy() { return "barking"; }
public void store() { System.out.println(this + "being stored in coach"); }
public void load() { System.out.println(this + "being loaded from kennel"); }
}
Cat:
class Cat extends Animal implements Talkable, Storable {
String color;
Cat(String name, int orderingKey) { super(name, orderingKey); this.color = "pink"; } Cat(String name, int orderingKey, String color) { super(name, orderingKey); this.color = color; }
public void store() { System.out.println(this + "being stored in first class"); }
public void load() { System.out.println(this + "being loaded into wooden box"); }
public String getType() { return "Kitty"; }
public String speaksBy() { return "meowing"; }
}
Storable:
interface Storable {
void store();
void load();
}
Talkable:
interface Talkable {
String getType();
String speaksBy();
}
output v Before Sort (Fluffy, 150), color = pink (Sweety, 200), color = yellow (Duke, 10), weight = 500.0 (Jason,5), weight = 100.0 After Sort (Sweety, 200), color = yellow (Fluffy, 150), color = pink (Jason,5), weight = 100.0 (Duke, 10), weight = 500.0 Animal (Sweety, 200), color = yellow is a kitty speaks by meowing (Sweety, 200), color = yellow being stored in first class (Sweety, 200), color = yellow being loaded into wooden box Animal (Fluffy, 150), color = pink is a kitty speaks by meowing (Fluffy, 150), color = pink being stored in first class (Fluffy, 150), color = pink being loaded into wooden box Animal (Jason,5), weight = 100.0 is a Doggie speaks by barking (Jason,5), weight = 100.0 being stored in coach (Jason,5), weight = 100.0 being loaded from kennel Animal (Duke, 10), weight = 500.0 is a Doggie speaks by barking (Duke, 10), weight = 500.0 being stored in coach (Duke, 10), weight = 500.0 being loaded from kennel output v Before Sort (Fluffy, 150), color = pink (Sweety, 200), color = yellow (Duke, 10), weight = 500.0 (Jason,5), weight = 100.0 After Sort (Sweety, 200), color = yellow (Fluffy, 150), color = pink (Jason,5), weight = 100.0 (Duke, 10), weight = 500.0 Animal (Sweety, 200), color = yellow is a kitty speaks by meowing (Sweety, 200), color = yellow being stored in first class (Sweety, 200), color = yellow being loaded into wooden box Animal (Fluffy, 150), color = pink is a kitty speaks by meowing (Fluffy, 150), color = pink being stored in first class (Fluffy, 150), color = pink being loaded into wooden box Animal (Jason,5), weight = 100.0 is a Doggie speaks by barking (Jason,5), weight = 100.0 being stored in coach (Jason,5), weight = 100.0 being loaded from kennel Animal (Duke, 10), weight = 500.0 is a Doggie speaks by barking (Duke, 10), weight = 500.0 being stored in coach (Duke, 10), weight = 500.0 being loaded from kennelStep by Step Solution
There are 3 Steps involved in it
Step: 1
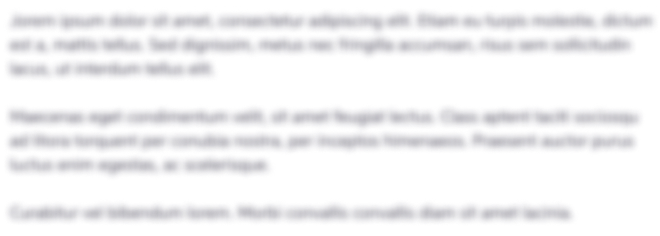
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started