Question
JAVA programming !! ------------------------------------- Your assignment is to write a grade book for a teacher. The teacher has a file, which includes student's name, and
JAVA programming !!
-------------------------------------
Your assignment is to write a grade book for a teacher. The teacher has a file, which includes student's name, and students test grades. There are four test scores for each student. One of the lowest test scores will be dropped. The teacher uses the following grading scale to assign a letter grade to a student: average between 90 to 100 is an A, 80 to 89 is a B, 70 to 79 is a C, 60 to 69 is a D, and lower than 59 is E. The average is based on the average of his or her three highest exam scores. For each student, your class should find the average test score, and the letter grade based on the average of the three highest test scores.
Write a class called GradeBook that uses the following instance variables (attributes):
An array of Strings to hold name of students. It will be sized based on the number of students in the file
An array of characters to hold the final letter grade of each student. It will be the same size as array above.
A two-dimensional array of type doubles consisting of test scores for each student. It is of size number of students by NUM_TESTS (there are 4 tests)
A public constant, NUM_TEST that contains the number of test scores. The number of students in the file might vary. You should check the file and count the number of records before instantiating an object of type GradeBook.
The number of students in the file might vary. You should check the file and count the number of records before instantiating an object of type GradeBook.
sample of input :
Sally 78 84 79 86 Rachel 68 76 87 76 Melba 87 78 98 88 Grace 76 67 89 0 Lisa 68 76 65 87
\\---------------------------------------------
import java.text.NumberFormat; import java.util.*; import java.io.*; public class Assignment { public static void main (String[] args) throws IOException { // BufferedReader stdin = new BufferedReader(new InputStreamReader (System.in)); // StringTokenizer tokenizer; Scanner stdin = new Scanner(System.in); int count = 0; System.out.println("Please enter the filename: "); String fileName = stdin.nextLine(); FileReader fr = new FileReader(fileName); BufferedReader inFile = new BufferedReader(fr); String line = inFile.readLine(); while (line != null) { count++; line = inFile.readLine(); } inFile.close(); System.out.println("Please enter a command or type \"?\" to see the menu:"); String command = stdin.nextLine(); GradeBook grades = new GradeBook(count); // Read the file and build grade book grades.fillGradeBook(fileName); // Calculate the letter grades grades.assignGrades(); while (command.charAt(0) != 'q') { switch (command.charAt(0)) { case 'a': // display all System.out.println(grades.toString()); break; case 'b' : // search System.out.println("Type student name? "); String name = stdin.nextLine(); int index = grades.search(name); char grade = grades.getLetterGrade(index); if (index != -1) System.out.println(name + " " + grade); else System.out.println("Student doesn't exist!"); break; case '?' : // help menu System.out.println("a: Display "); System.out.println("b: Search "); System.out.println("?: Help menu "); System.out.println("q: Stop the program "); break; case 'q': // stop the program break; default: System.out.println("Illegal cammand "); } // end of switch System.out.println(); System.out.println("Please enter a command or type \"?\" to see the menu:"); command = stdin.nextLine(); } // end of while }// end main } // end Assignment8
Sample Output
---------------------------
Method Description constructor to instantiate all the instance variables. The size is the number of students in the file. The number of test scores is a constant. radeBook (int size) public void fillGradeBook (String fileName) method to fill the array student name and test scores from the file. The file name is ssed as a string. Since this method is doing IO operation you should specify about IO exception; For example throws IOException. helper method that calculates the average of the three highest exam scores; returns average score if the parameter is a valid index number. Return -1 otherwise. private double getStudentAverage (int index) lates the average score and assigns a letter grade as follows: 90-100 lic void assignGrades () ? 80-89 70-79 D 60-69 E 0-59 public String getStudentName int Returns the student name if the parameter is a valid index number; return null index) ise. public char index) getLetterGrade (int Returns the letter grade of the student that corresponds to the parameter index if the r is a valid index number. Returns a blank otherwise Returns the index of the student whose name matches the parameter target; returns -1 if the target is not found public int search (String target) Returns a String, which contains students' names, averages, and the final letter grades for each student in the array. (Please see the sample output) lic String toString ) Note: You may add more methods to the class if it makes it easier to implement
Step by Step Solution
There are 3 Steps involved in it
Step: 1
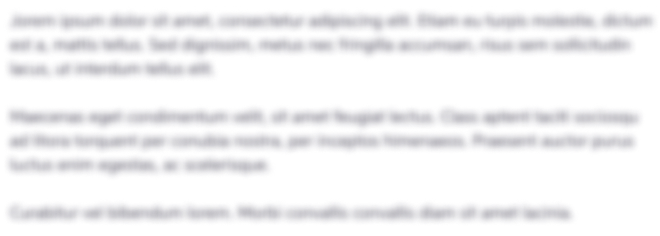
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started