Question
Java Project Requirements: The program will Accept as input information about a song. This information will include the name of the song and the notes
Java
Project Requirements:
- The program will
- Accept as input information about a song. This information will include the name of the song and the notes for the song.
- Run in two modes of operation, one is for listening and the other is for interactive game play
- Note Class
- This class should be used to store each note and to convert from a textual note, such as Db0 (D flat 0) to a frequency, 17.32. Additionally, all notes will need to be played for the duration specified in the input.
- Instance variables
- String note text representation of note
- double frequency frequency of note
- double duration length of time a note played
- boolean badNote indicates note is not valid (see table below for valid notes)
- Constructor
- Takes in a note and durations as Strings
- Sets the note instance variable
- Calls getHz which uses the note to set the frequency
- Converts the duration parameter to a double and sets the duration(use Double.parseDouble API method) .
- Catches BadNoteExceptions which prints the appropriate message and sets the badNote instance variable to true
- Methods
- Accessor method for note, frequency, duration and badNote(should be called isBadNote)
- setFrequency -private helper method
- sets the frequency value
- Uses the octave and the key to calculate the notes frequency
- Calculation is notes base frequency * 2 (octave). See Notes Base Frequency chart below. Example A4 would be 27.5 * 2 4 or 440 hz.
- Throws BadNoteException (See below)if Note key is invalid.
-
- playSound plays a sound.
- Calls the Tone classs static method playNote(provided)
- Passing the Notes frequency and duration to this static method.
- equals(String anotherNote)
- Takes in a String representing another Notes tone(A,B, C etc)
- Compares the two Notes tones
- Returns true if they match, false is they do not match.
- playSound plays a sound.
- Abstract Song Class
- Instance variables
- Array of Notes holds the note object needed to play the song
- nameOfSong String
- noteNumber int representing the current note being played.
- Constructor
- Takes in the name of the song and a String array of Notes, (A4, G3 etc)
- Sets the nameOfSong instance variable
- Uses the String array of Notes to fill the Array of Notes with Note objects.
- Methods
- getNameOfSong accessor method for name of song
- getNextNote returns the next Note object in the array.
- isEndOfSong returns true if end of song, false if not
- toString returns all the Notes in the array in a neatly formatted String
- Instance variables
- DemoSong class
- Extends Song Class no instance variables
- Constructor
- Takes in the name of the song and a String array of Notes, (A4, G3 etc)
- Pass parameters to super class.
- Methods
- playDemo
- Plays each note in the Song Array of notes.
- Remember playSong method is in the Song class.
- playDemo
- SongGame class
- Extends Song class
- The class must
- Provide the name of the song
- Provide and play the first note
- Ask the user to guess the next note
- If the user is correct play the note and ask for the next note
- If incorrect print an error message and ask again for the next note
- Provide the number of incorrectly guessed notes upon completion of the game.
- Much of the details of implementation of this class is left up to the developers. Use good coding practices.
- BadNoteException
- Extends the Exception class
- User defined exception
- Thrown if invalid note used.
- Constructor takes in a String representing an appropriate error message.
- Sample runs
First Run
Welcome to Glockenspiel Hero, the funnest game you'll build all week!
Enter 'd' to play a demo of the song, of 'p' to play the game: p
Guess the notes of the song "Mary Had a Little Lamb"
The first note is A, which sounds like this...
Next note: g
Next note: f
Great job! You got a perfect score!
Second Run
Welcome to Glockenspiel Hero, the funnest game you'll build all week!
Enter 'd' to play a demo of the song, of 'p' to play the game: p
Guess the notes of the song "Mary Had a Little Lamb"
The first note is A, which sounds like this...
Next note: e
Sorry, that is not correct. Try again.
Next note: f
Sorry, that is not correct. Try again.
Next note: d
Sorry, that is not correct. Try again.
Next note: g
Next note: f
You got 3 notes incorrect.
- Create UML Class Diagram for the final version of your project. The diagram should include:
- All instance variables, including type and access specifier (+, -);
- All methods, including parameter list, return type and access specifier (+, -);
- Include Generalization and Aggregation where appropriate.
- The Driver does not need to be included.
- Refer to the UML Distilled pdf on the content page as a reference for creating class diagrams.
- A Link to a drawing program, Dia, is also posted on the content page.
Use the command line argument feature of Eclipse to pass in the song data.
I have the files for Driver.java, StdAudio.java, and Tone.java but they are fairly lengthy. If needed just write a comment.
Here is the .txt file
twinkle_twinkle.java
"Twinkle, Twinkle Little Star" C5 0.5 C5 0.5 G5 0.5 G5 0.5 A5 0.5 A5 0.5 G5 1 F5 0.5 F5 0.5 E5 0.5 E5 0.5 D5 0.5 D5 0.5 C5 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
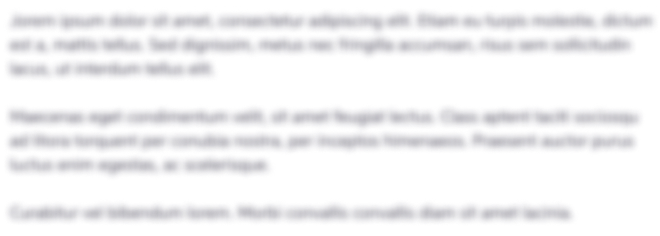
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started