Answered step by step
Verified Expert Solution
Question
1 Approved Answer
//java public class DBConfig { static String db_url = jdbc:mysql://localhost:3306/rubik ; static String user = System. getenv ( MYSQL_USER ); // TODO ensure you've set
//java public class DBConfig { static String db_url = "jdbc:mysql://localhost:3306/rubik"; static String user = System.getenv("MYSQL_USER"); // TODO ensure you've set this environment variable static String password = System.getenv("MYSQL_PASSWORD"); // TODO ensure you've set this environment variable } ==================================================================================================================
class Rubik { RubikGUI gui; public static void main(String[] args) { Rubik rubikProgram = new Rubik(); rubikProgram.start(); } public void start() { gui = new RubikGUI(this); } }
=====================================================================================
/* Talk to your database here. */ import javax.swing.table.AbstractTableModel; public class RubikModel extends AbstractTableModel{ @Override public int getRowCount() { return 0; //TODO } @Override public int getColumnCount() { return 0; // TODO } @Override public Object getValueAt(int rowIndex, int columnIndex) { return null; // TODO } }
===========================================================================================================
public class RubikGUI extends JFrame { private JPanel mainPanel; // TODO configure and implement functionality in your GUI. public RubikGUI(Rubik rubikProgram) { setContentPane(mainPanel); pack(); setVisible(true); setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); // TODO configure and implement functionality in your GUI. } // Use these methods to show dialogs. Or your tests may time out. /* This will return * * JOptionPanel.YES_OPTION if the user clicks 'yes' * JOptionPanel.NO_OPTION if the user clicks 'no'. * * * You can call this method and check for the return type, e.g. * * if (showYesNoDialog("Delete this solver?") == JOptionPane.YES_OPTION) { * // User clicked yes, so go ahead and delete * } else { * // User did not click yes. * } * */ protected int showYesNoDialog(String message) { return JOptionPane.showConfirmDialog(this, message, null, JOptionPane.YES_NO_OPTION); } /* * Call this method to show an alert/message dialog with the message provided. * */ protected void showAlertDialog(String message) { JOptionPane.showMessageDialog(this, message); }}
===============================================================================================
//instruction
GUI and Database Programs: Rubik Cube Solver Database Write an GUI application to manage a database of things that can solve Rubik's cubes, and their fastest time. ### Database setup: You will need to create a database called **rubik**. You will also need to create a test database called **rubik_test** The rubik database should have a table called **cube_records**. The rubik_test database should also have a table called **cube_records**. The cubes table (in both databases) should have three columns, * **id** the primary key column, an auto-incrementing integer * **solver_name** for the name of a thing that can solve Rubiks cubes * **time_seconds** a double number, for the time taken to solve the Rubiks cube. Verify the DB connection URL is correct in DBConfig. Add the following permissions to your user, for both databases. `grant update, select, insert, delete on rubik.cube_records to 'YOURUSER'@'localhost';` Set two environment variables called `MYSQL_USER` and `MYSQL_PASSWORD` to hold your MySQL username and password, respectively. You will need to configure the Run Configuration for both the main program, *and* the test, to use these environment variables. Heres some data: ``` solver_name time_seconds Cubestormer II 5.27 Cubestormer III 3.253 Sub1 0.637 Mats Valk 4.74 Feliks Zemdegs 4.73 Patrick Ponce 4.69 ``` Source: [http://www.recordholders.org/en/list/rubik.html](http://www.recordholders.org/en/list/rubik.html) ### GUI setup Create a GUI to view, edit, add to, and delete, this data. The solversTable should get data from a RubikModel AbstractTableModel. Your GUI should have these components, configured in the following way, **For displaying all current data:** * A JTable called `solversTable` with two columns for solver_name and time_seconds, that displays all of the data from those columns in the database. It should be configured to be non-editable, and single row selection. When the program loads, it will show all (solver_name and time_seconds) data from the database. As the user adds, deletes, or edits data, the table will update. *Keep your data sorted in order of time, with fastest first*. **For adding a new cube solver to the database:** * A JTextField called `newCubeSolverNameText` * A JTextField called `newCubeSolverTimeText` * A JButton called `addNewSolverButton` When the user clicks the `addNewSolverButton` button, validate that a name and a double time have been entered in the appropriate JTextBoxes. The name must be at least one character. Empty names are not acceptable. The time must be positive, and not zero. If so, add the data to the database and update `solversTable`. If not, display an JOptionPane alert dialog, and do not modify the database or JTable. **For modifying a time** * A JButton called `updateTimeButton` * JTextField called `updateTimeText` * JLabel called `updateSolverNameLabel` When the user selects a row in the table, the current name will be shown in updateSolverNameLabel and that solver's time will be shown in updateTimeText. To update a time, the user will select the solver's row in the table, edit the updateTimeText to the new time, and click `updateTimeButton`. The program will validate that the new time is also a non-zero double value. If so, the database table will be updated, and the GUI table will update. If the new text entered is not a double, show a JOptionPane alert dialog and do not modify the DB table or JTable. The current row should remain selected. If no user is selected in the table, show an alert dialog telling the user to select a row. **For deleting a cube solver** * A JButton called `deleteSolverButton` To delete a row, the user will select that row in the table and click `deleteSolverButton`. Show a JOptionPane dialog with 'Yes' and 'No' options to confirm the delete operation. If the user clicks 'Yes' then delete the row from the database and update the JTable. If the user clicks 'No' then do nothing. For all parts of this lab, make sure you add appropriate error handling, and close all resources (ResultSets, PreparedStatements, Statements, Connections) when your program is done with them. Use PreparedStatements for updates, add, delete operations. When showing dialogs, use the methods provided in RubikGUI, or your tests will time out.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
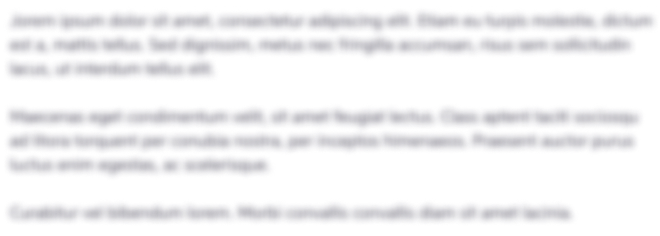
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started