Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Java Question Provided Junit Test Class import static org.junit.jupiter.api.Assertions.*; import java.util.ArrayList; import java.util.Scanner; import org.junit.jupiter.api.Test; class RouteRecommenderTest { private static String testInput0 = route 2
Java Question Provided Junit Test Class
import static org.junit.jupiter.api.Assertions.*; import java.util.ArrayList; import java.util.Scanner; import org.junit.jupiter.api.Test; class RouteRecommenderTest { private static String testInput0 = "route 2 15 " + "A3 4 " + "B3 3 " + "C3 5 " + "D3 2 " + "end " + "end " + "A3 D3 1 " + "end "; private static String [] testOutput0 = { "At stop A3 take bus #2", "Get off at stop D3" }; private static String testInput1 = "route 2 15 " + "A3 4 " + "B3 3 " + "C3 5 " + "D3 2 " + "end " + "route 4 10 " + "B1 3 " + "B2 1 " + "B3 6 " + "B4 2 " + "B5 3 " + "end " + "end " + "A3 B5 1 " + "end "; private static String [] testOutput1 = { "At stop A3 take bus #2", "At stop B3 switch to bus #4", "Get off at stop B5" }; private static String testInput2 = "route 1 15 " + "A3 4 " + "B3 3 " + "C3 5 " + "D3 2 " + "end " + "route 2 15 " + "A3 4 " + "D3 2 " + "end " + "end " + "A3 D3 1 " + "end " + ""; private static String [] testOutput2 = { "At stop A3 take bus #2", "Get off at stop D3" }; private static String testInput3 = "route 1 15 " + "A3 4 " + "B3 3 " + "C3 5 " + "D3 2 " + "end " + "route 2 15 " + "A3 0 " + "D3 2 " + "end " + "end " + "A3 D3 1 " + "end " + ""; private static String [] testOutput3 = { "At stop A3 take bus #1", "Get off at stop D3" }; private static String testInput4 = "route 2 15 " + "A3 4 " + "B3 3 " + "C3 5 " + "D3 2 " + "end " + "route 4 10 " + "B1 3 " + "B2 1 " + "B3 6 " + "B4 3 " + "end " + "route 3 20 " + "D3 3 " + "D4 1 " + "C4 6 " + "B4 4 " + "A4 3 " + "end " + "end " + "A3 A4 1 " + "end " + ""; private static String [] testOutput4 = { "At stop A3 take bus #2", "At stop B3 switch to bus #4", "At stop B4 switch to bus #3", "Get off at stop A4" }; private static String testInput5 = "route 2 15 " + "A3 4 " + "B3 3 " + "C3 5 " + "D3 2 " + "end " + "route 4 10 " + "B1 3 " + "B2 1 " + "B3 6 " + "B4 3 " + "end " + "route 3 20 " + "D3 3 " + "D4 1 " + "C4 6 " + "B4 4 " + "A4 3 " + "end " + "end " + "A3 A4 1 " + "B1 C4 2 " + "end " + ""; private static String [] testOutput5 = { "At stop A3 take bus #2", "At stop B3 switch to bus #4", "At stop B4 switch to bus #3", "Get off at stop A4", "At stop B1 take bus #4", "At stop B3 switch to bus #2", "At stop D3 switch to bus #3", "Get off at stop C4" }; private static Scanner mkTest( String input ) { return new Scanner( input ); } private static ArrayList mkOutput( String [] output ) { ArrayList al = new ArrayList(); for( String s : output ) { al.add( s ); } return al; } private static boolean doTest( String input, String [] output ) { Tester t = new RouteRecommender(); ArrayList al = t.compute( mkTest( input ) ); System.out.println( "Input: " ); System.out.println( input ); System.out.println( "Generated output" ); for( String s : al ) { System.out.println( s ); } System.out.println( "Expected output" ); for( String s : output ) { System.out.println( s ); } System.out.println( "---------------------------------------------------" ); return al != null && al.equals( mkOutput( output ) ); } @Test void testEmpty() { assertTrue( doTest( testInput0, testOutput0 ), "Basic route" ); } @Test void test1() { assertTrue( doTest( testInput1, testOutput1 ), "One switch route" ); } @Test void test2() { assertTrue( doTest( testInput2, testOutput2 ), "Take the shorter route" ); } @Test void test3() { assertTrue( doTest( testInput3, testOutput3 ), "Waiting for bus affects schedule" ); } @Test void test4() { assertTrue( doTest( testInput4, testOutput4 ), "Multiple bus switches" ); } @Test void test5() { assertTrue( doTest( testInput5, testOutput5 ), "Multiple queries" ); } }
Java Tester Interface Class
import java.util.ArrayList; import java.util.Scanner; public interface Tester { // This method takes a Scanner object contianing the input // and returns an ArrayList object containing the output. // // Parameters: // Scanner input: is a Scanner object that is a stream of text // containing the input to your program. // // Returns: an ArrayList of Strings. Each string is a line of output // from your program. public ArrayList compute( Scanner log ); }Problem:Recommend a Bus Route Given a transit network, the start and end bus stops of the trip, and the riders expected arrival at a bus stop, provide a trip itinerary to get the rider to their destination as quickly as possible. For example, given the following transit network, followed by a trip query the route 2 15 ?? 4 B3 3 C3 5 D3 2 end route 4 10 B1 3 B2 1 B3 6 B4 3 end route 3 20 D3 3 D4 1 C4 6 B4 4 A4 3 end end A3 A4 1 end Figure 2: Sample transit network and directions query resulting recommendations would be At stop A3 take bus #2 At stop B3 switch to bus #4 At stop B4 switch to bus #3 Get off at stop A4 Figure 3: Recommended bus route based on input in Figure 2 Problem:Recommend a Bus Route Given a transit network, the start and end bus stops of the trip, and the riders expected arrival at a bus stop, provide a trip itinerary to get the rider to their destination as quickly as possible. For example, given the following transit network, followed by a trip query the route 2 15 ?? 4 B3 3 C3 5 D3 2 end route 4 10 B1 3 B2 1 B3 6 B4 3 end route 3 20 D3 3 D4 1 C4 6 B4 4 A4 3 end end A3 A4 1 end Figure 2: Sample transit network and directions query resulting recommendations would be At stop A3 take bus #2 At stop B3 switch to bus #4 At stop B4 switch to bus #3 Get off at stop A4 Figure 3: Recommended bus route based on input in Figure 2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
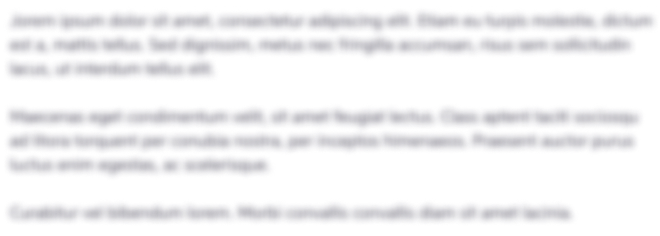
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started