Question
Java question The problem is in the public stat void divide portion of my code. If the user enters a 0 as the second number,
Java question The problem is in the public stat void divide portion of my code. If the user enters a 0 as the second number, the result should be The current value is NaN. I am getting that but I am also getting The current value is 48.
Also, if an incorrect choice is made it should tell the user that. It's in my code but not working.
import java.util.Scanner;
public class Memory {
//Static variable to hold result
private static double currentValue=0;
//Main function
public static void main(String[] args)
{
Scanner choose = new Scanner(System.in);
int choice = 0;
String prompt;
boolean right = false;
double num2;
//Iterate till user wants to quit
do
{
//Displaying current value
System.out.println("The current value is " + currentValue);
//Displaying menu
choice = displayMenu(choose);
//Checking for valid option
if ((choice <= 6) && (choice > 0))
{
//Calling appropriate function
switch(choice)
{
case 1: num2 = getOperand("What is the second number? "); add(num2); break;
case 2: num2 = getOperand("What is the second number? "); subtract(num2); break;
case 3: num2 = getOperand("What is the second number? "); multiply(num2); break;
case 4: num2 = getOperand("What is the second number? "); divide(num2); break;
case 5: case 6: currentValue = 0; System.out.println("Goodbye!"); break;
}
}
else
{
right = true;
System.out.println("I'm sorry, " + choice + " wasn't one of the options.");
}
}while (choice != 6);
}
//Displaying menu
public static int displayMenu(Scanner choose)
{
int choice;
System.out.println(" ");
System.out.println("Menu ");
System.out.println("1. Add");
System.out.println("2. Subtract");
System.out.println("3. Multiply");
System.out.println("4. Divide");
System.out.println("5. Clear");
System.out.println("6. Quit");
System.out.println(" ");
System.out.print("What would you like to do? ");
choice = choose.nextInt();
return choice;
}
public static double getOperand(String prompt)
{
Scanner choose = new Scanner(System.in);
System.out.print(prompt);
double option = choose.nextDouble();
return option;
}
public double getCurrentValue(double currentValue)
{
return currentValue;
}
public static void add(double num2)
{
currentValue += num2;
}
public static void subtract(double num2)
{
currentValue -= num2;
}
public static void multiply(double num2)
{
currentValue *= num2;
}
public static void divide(double num2)
{
if (num2 == 0)
{
System.out.println(" The current value is Nan. ");
}
else
{
currentValue /= num2;
}
}
public void clear()
{
currentValue = 0;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
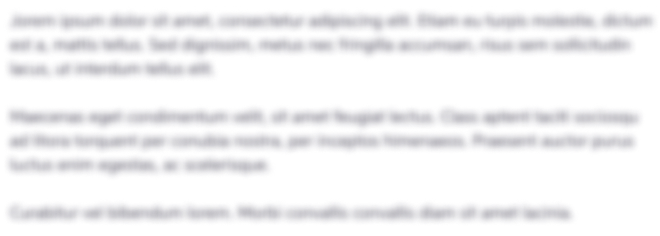
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started