Question
Java Single Task: Please help me with a single class (class MorseCipher) in this program. This program is about Encryption (Hierarchy). All required classes to
Java Single Task:
Please help me with a single class (class MorseCipher) in this program. This program is aboutEncryption(Hierarchy). All required classes to be used to extend will be provided below (links). Tester cases for this class is also at the bottom. Please read and help me. Thank you so much.
- Here is an overview of this program.
Object | +--Alphabet (finished) +--AlphabetException (finished) | | | +--BadIndexAlphabetException (finished) | | | +--MissingCharAlphabetException (finished) | +--Cipher (abstract) --> Provided | +--MorseCipher (HELP !!!) | +--SymmetricCipher (abstract) (Finished) | +--CaesarCipher | +--VigenereCipher
Here is the link to the java codes for class Alphabet, AlphabetException, BadIndexAlphabetExceptionandMissingCharAlphabetException that I have finished (Will be used to inheret for this CaesarCipher class):https://paste.ee/p/19qsM
-------------------Here is the java codes for the abstract class (Class Cipher) that will be used to extend------------
public abstract class Cipher {
public abstract String encrypt(String s);
public abstract String decrypt(String s);
}
----------------------------Here are the task and examples for class MorseCipher------------------
class MorseCipher
Morse code is actually a cipher with encryption and decryption actions. Unusually, the intent is not to hide information, merely to represent it in a form more suited for transit. By representing letters with dashes and dots, it is easy to transmit data through lossy environments such as transmission across wires.
The project pack contains a start for MorseCipher.java which defines the alphabet we will use along with the Morse codes associated with the those letters . You will implement the same encrypt() and decrypt() methods as before. Other than the series of dots and dashes representing each letter, we see three spaces separating each letter of a word and seven spaces separating words. There are no spaces at the end of the message. We must create exactly the correct amount of spacing in our encrypted messages, and we must correctly extract the letters and spaces when decrypting.
Fields-------------
Due to the possibility of transcription errors, these two definitions are provided directly (and in the provided files).
public static final String letters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890"; public static final String[] codes = { ".-", /* A */ "-...", /* B */ "-.-.", /* C */ "-..", /* D */ ".", /* E */ "..-.", /* F */ "--.", /* G */ "....", /* H */ "..", /* I */ ".---", /* J */ "-.-", /* K */ ".-..", /* L */ "--", /* M */ "-.", /* N */ "---", /* O */ ".--.", /* P */ "--.-", /* Q */ ".-.", /* R */ "...", /* S */ "-", /* T */ "..-", /* U */ "...-", /* V */ ".--", /* W */ "-..-", /* X */ "-.--", /* Y */ "--..", /* Z */ ".----", /* 1 */ "..---", /* 2 */ "...--", /* 3 */ "....-", /* 4 */ ".....", /* 5 */ "-....", /* 6 */ "--...", /* 7 */ "---..", /* 8 */ "----.", /* 9 */ "-----", /* 0 */ };
Methods------------------
public MorseCipher(). Initializes your MorseCipher objects as needed, perhaps setting any private variables you choose to have (certainly this is optional).
public String encrypt(String plainText). Converts a string of letters, digits, and spaces to the corresponding morse code representation. All lowercase letters are implicitly converted to uppercase during encryption.
public String decrypt(String cryptText). Converts a string of morse code representation back to a string of uppercase letters, digits, and spaces. Any letters that were originally lowercase will only appear as uppercase.
Strategies for Implementation of Morse Methods
Unlike the other ciphers, the MorseCipher does not involve any secret information. Instead, it is more properly thought of as encoding/decoding based on lookup tables of character equivalences.
In the provided MorseCipher.java file, two tables appear
A String called letters which gives the valid characters in the MorseCode. This table is a good candidate for an Alphabet object to ease looking up characters.
An array of Strings called codes which give the series of dashes and dots equivalent to each character in the allowed set of characters.
The central strategy while encrypting is simple: for each character in the message, determine the index of that character and output the equivalent dash-dot string. Each dash-dot is separated by 3 spaces and each word is separated by 7 spaces
Message: "ALL YOUR BASE" A: ".-" " " L: ".-.." " " L: ".-.." " " _: " " Y: "-.--" " " O: "---" " " U: "..-" " " R: ".-." " " _: " " B: "-..." " " A: ".-" " " S: "..." " " E: "." Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... .
Decrypting is a matter of reading dots/dashes until a space is encountered. The sequence of dashes/dots is looked up in the table of equivalences and the equivalent character is added to the growing message. After this, count how many spaces there are prior to the next dot/dash: 3 spaces means the next character is part of the same word, 7 means the next character starts a new word and a space should be put into the growing decoded message.
Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: "" mesg: "" Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: ".-" -> A mesg: "A" Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: "" -> 3 spaces, same word mesg: "A" Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: ".-.." -> L mesg: "AL" Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: "" -> 3 spaces, same word mesg: "AL" Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: ".-.." -> L mesg: "ALL" Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: "" -> 7 spaces, new word mesg: "ALL " Encrypted: .- .-.. .-.. -.-- --- ..- .-. -... .- ... . position: ^ code: "-.--" -> Y mesg: "ALL Y" ...
The code to accomplish decryption is more intricate than other processes we have previously dealt with. It may be helpful to establish private helper methods to perform tasks such as:
Scan through spaces in a string until the next non-space character and return the number of spaces.
Scan all non-space characters from a given start position and return a string of those non-space characters.
While not strictly necessary, such little methods can simplify your the logic of your decryption routine to make it "prettier". Make sure to amply document any helper methods you use.
------------Java Codes for class MorseCipher-----Please solve from here-------------
class MorseCipher /* extends ??? */ { public static final String letters = "ABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890"; public static final String[] codes = { ".-", /* A */ "-...", /* B */ "-.-.", /* C */ "-..", /* D */ ".", /* E */ "..-.", /* F */ "--.", /* G */ "....", /* H */ "..", /* I */ ".---", /* J */ "-.-", /* K */ ".-..", /* L */ "--", /* M */ "-.", /* N */ "---", /* O */ ".--.", /* P */ "--.-", /* Q */ ".-.", /* R */ "...", /* S */ "-", /* T */ "..-", /* U */ "...-", /* V */ ".--", /* W */ "-..-", /* X */ "-.--", /* Y */ "--..", /* Z */ ".----", /* 1 */ "..---", /* 2 */ "...--", /* 3 */ "....-", /* 4 */ ".....", /* 5 */ "-....", /* 6 */ "--...", /* 7 */ "---..", /* 8 */ "----.", /* 9 */ "-----", /* 0 */ }; }
-----------------------Here is the tester cases-------------------
Tester cases for MorseCipher: https://paste.ee/p/H72le
Please check with this tester cases. Thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
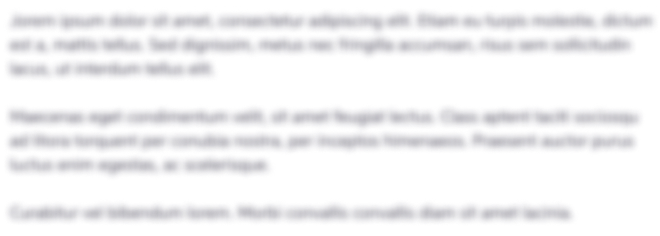
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started