Question
JAVA SinglyLinkedList.java public class SinglyLinkedList { //---------------- nested Node class ---------------- private static class Node { private E element; private Node next; public Node(E e,
JAVA
SinglyLinkedList.java
public class SinglyLinkedList
//---------------- nested Node class ----------------
private static class Node
private E element;
private Node
public Node(E e, Node
element = e;
next = n;
}
public E getElement() {
return element;
}
public Node
return next;
}
public void setNext(Node
next = n;
}
} //----------- end of nested Node class -----------
private Node
private Node
private int size = 0;
public SinglyLinkedList() {
}
public int size() {
return size;
}
public boolean isEmpty() {
return size == 0;
}
public E first() {
if (isEmpty()) {
return null;
}
return head.getElement();
}
public E last() {
if (isEmpty()) {
return null;
}
return tail.getElement();
}
// update methods
public void addFirst(E e) {
head = new Node(e, head);
if (size == 0) {
tail = head;
}
size++;
}
public void addLast(E e) {
Node
if (isEmpty()) {
head = newest;
} else {
tail.setNext(newest);
}
tail = newest;
size++;
}
public E removeFirst() {
if (isEmpty()) {
return null;
}
E answer = head.getElement();
head = head.getNext();
size--;
if (size == 0) {
tail = null;
}
return answer;
}
Lab2_Driver.java
public class Lab2_Driver {
public static void main(String[] args) {
}
Code the SinglyLinkedList class from our class notes (L03_). Override the toString method by using StringBuilder to list every element on a new line, in order from head to tail. In the Lab2_Driver file, create a SinglyLinkedList instance that stores integer values. e.g., SinglyLinkedListStep by Step Solution
There are 3 Steps involved in it
Step: 1
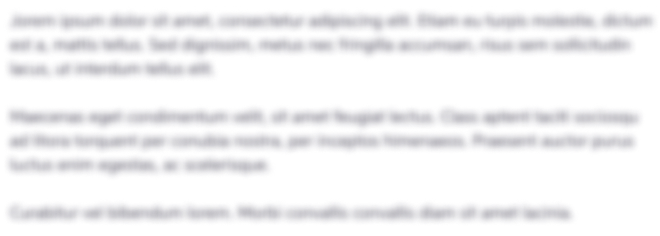
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started