Answered step by step
Verified Expert Solution
Question
1 Approved Answer
JAVA SocketManager Ask all the following of the user: A port to run its server on (something like 8800 or 8888) An IP of another
- JAVA
- SocketManager
- Ask all the following of the user:
- A port to run its server on (something like 8800 or 8888)
- An IP of another server (local computer is 127.0.0.1)
- NOTE: If running on two computers, to get the IP address of a computer, in a command prompt or powershell, simply type ipconfig and look for the IPV4 address or IPV6 whichever comes first. It will probably look something like this: 192.168.0.3
- The port of that other server.
- After starting up the server, it should enter a never-ending loop that does the following:
- Asks the user for three numbers separated by commas. So the user would enter something like this:
- SocketManager
- Ask all the following of the user:
- A port to run its server on (something like 8800 or 8888)
- An IP of another server (local computer is 127.0.0.1)
- NOTE: If running on two computers, to get the IP address of a computer, in a command prompt or powershell, simply type ipconfig and look for the IPV4 address or IPV6 whichever comes first. It will probably look something like this: 192.168.0.3
- The port of that other server.
- After starting up the server, it should enter a never-ending loop that does the following:
- Asks the user for three numbers separated by commas. So the user would enter something like this:
- Ask all the following of the user:
4,7,22
- Sends that raw text to the server using the SocketClient class as we did in class.
- IMPORTANT: The items sent should be comma-delimited all concatenated as one string.
- SocketServer
- When the server receives a message from a client, it should try to parse the comma-delimited message into an array.
- HINT: Use the sReceivedMessage.split(",") to get a String array of the comma-delimited items.
- Then turn each array string into three int variables.
- Use Integer.parseInt() to do this on each item in the array.
- Then sum these numbers into a result.
- When the server receives a message from a client, it should try to parse the comma-delimited message into an array.
- Reply with the sum of these numbers.
import java.util.Scanner; public class SocketManager { public static void main(String[] args){ //get port for this server to listen on. System.out.print("Enter port for this server to listen on: "); int iThisServerPort = new Scanner(System.in).nextInt(); SocketServer oServer = new SocketServer(iThisServerPort); System.out.println(""); Thread oServerThread = new Thread(oServer); //calling run method oServerThread.start(); //get details of server to connect to. System.out.print("Enter IP address of server to connect to: "); System.out.println(""); String sOtherServerIP = new Scanner(System.in).nextLine(); System.out.print("Enter port of server to connect to: "); int iOtherServerPort = new Scanner(System.in).nextInt(); while(true) { System.out.print("Enter message to sent to server: "); String sMessage = new Scanner(System.in).nextLine(); SocketClient oClient = new SocketClient(); String sReply = oClient.connectForOneMessage(sOtherServerIP, iOtherServerPort, sMessage); System.out.println("[client] Remote server reply: " +sReply); } } }
import java.io.*; import java.net.ServerSocket; import java.net.Socket; public class SocketServer implements Runnable { private int thisServerPort; public SocketServer(int iPort){ thisServerPort = iPort; } //constructor public void run(){ try(ServerSocket oServerSocket = new ServerSocket(thisServerPort)){ System.out.println("Server is listening on port " + thisServerPort); while(true){ Socket oSocket = oServerSocket.accept(); System.out.println("[server]: new client connected: " + oSocket.getRemoteSocketAddress()); oSocket.sReceivedMessage.split(","); InputStream input = oSocket.getInputStream(); BufferedReader reader = new BufferedReader(new InputStreamReader(input)); OutputStream output = oSocket.getOutputStream(); PrintWriter writer = new PrintWriter(output, true); //Get one time message from client. String sReceivedMessage = reader.readLine(); System.out.println("[server]: Server received message: " + sReceivedMessage); //Send message back to calling client writer.println("Server received message: "+ sReceivedMessage); writer.flush(); } }catch (IOException ex){ System.out.println("[server]: Server exception: " + ex.getMessage()); //ex.printStackTrace(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
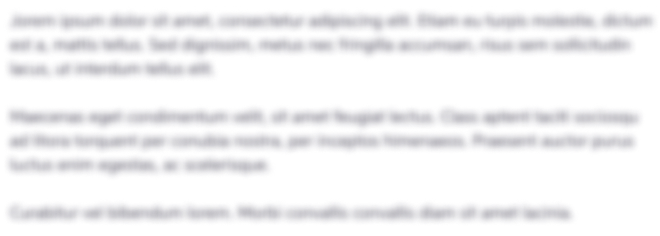
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started