Question
JAVA: This is a very easy problem: You MUST use a foreach loop to iterate throughthe list. You may have to adjust othermethods in class
JAVA: This is a very easy problem:
You MUST use a foreach loop to iterate throughthe list. You may have to adjust othermethods in class PolymorphismPractice but I onlyneed help on STEP 3, the getInfo method.
QUESTION:
Let’s complete the getInfo method. Start by editing this methodheader for getInfo so that takes in an ArrayList of Shape objectsas a parameter. Now we will be iterating over the list and addinginformation to a StringBuilder. For this method use a foreach loopto iterate through the list. For each shape in the array, add theshape information to your StringBuilder HINT: use the toStringmethod for that Object.
Let’s also add the lines “Has area: the area of theshape”, as well as “Has circumference: the circumferenceof the shape”. Please make sure that the area andcircumference found are rounded to two decimal places. Separateevery object with a new line character. Finally, return the Stringof all the information found.
An example of how this should look whenprinted:
Circle with radius: 70.84, Centered at X: 69.27 Y: 89.29Has area: 15764.34Has circumference: 445.08Rectangle with height: 9.34 and width: 70.01, Centered at X: 9.69 Y: 84.71Has area: 654.07Has circumference: 158.70
Testing
Call and print the getInfo method inside your main method.
------------------------------------------------------------------------------------------------------------
CODE TO COPY:
------------------------------------------------------------------------------------------------------------
abstract class Shape{ public double x; public double y; abstract double calculateArea(); abstract double calculateCircumference(); public double getX() { return x; } public double getY() { return y; } public void setX(double x) { this.x = x; } public void setY(double y) { this.y = y; }}
-------------------------------------------------------------------------------------------------------------
------------------------------------------------------------------------------------------------------------
public class Circle extends Shape { public double radius; public Circle(double radius, double X, double Y){ this.radius = radius; this.setX(X); this.setY(Y); } public double getRadius() { return radius; } public void setRadius(double radius) { this.radius = radius; } public double calculateArea(){ return (Math.PI * radius * radius); } public double calculateCircumference(){ return (2 * Math.PI * radius); } public String toString() { return String.format("Circle with radius: %.2f, Centered at X: %.2f Y: %.2f%n", this.getRadius(), this.getX(), this.getY()); }}
------------------------------------------------------------------------------------------------------------------------------------
--------------------------------------------------------------------------------------------------------------------------------
public class Rectangle extends Shape { public double height; public double width; public double getHeight() { return height; } public double getWidth() { return width; } public void setHeight(double height) { this.height = height; } public void setWidth(double width) { this.width = width; } public Rectangle(double height, double width, double X, double Y){ this.height = height; this.width = width; this.setX(X); this.setY(Y); } public double calculateArea(){ return (height*width); } public double calculateCircumference(){ return (height*2) + (width*2); } public String toString() { return String.format("Rectangle with height: %.2f and width: %.2f, " + "Centered at X: %.2f Y: %.2f%n", this.getHeight(), this.getWidth(), this.getX(), this.getY()); }}
---------------------------------------------------------------------------------------------------------------------------
CODE TO EDIT: STEP 3 (getInfo method) using a foreach loop
-------------------------------------------------------------------------------------------------------------------------
import java.util.ArrayList;public class PolymorphismPractice { public static void main(String[] args) { //Step 1 Initialize an Array list of type Shape ArrayList aList = new ArrayList<>(); //Step 2 initialize the ArrayList with 5 circles and squares (10 total) with //random X and Y values and random length width //Hint You can make this easier using a for loop, also try using Math.random() //Let’s now fill the ArrayList with 5 Circles and 5 Rectangles (for a total of 10 objects). //A for loop is definitely helpful in this step, and also consider using Math.random(). for (int i = 0; i < 10; i++) { Shape shape; if (i % 2 == 0) { double radius = Math.random(); double x = Math.random(); double y = Math.random(); shape = new Circle(radius, x, y); } else { double height = Math.random(); double width = Math.random(); double x = Math.random(); double y = Math.random(); shape = new Rectangle(height, width, x, y); } aList.add(shape); } //To test this step, you can use the ArrayList size() method to make sure it has a size of 10, //or try printing out all 10 items in the ArrayList. //Test your methods here! for (int i = 0; i < aList.size(); i++) { System.out.println(aList.get(i)); } System.out.println(findCircles(aList)); System.out.println(getInfo(aList)); } //Step 3 complete getInfo method as shown in instructions public static String getInfo(ArrayList list) { StringBuilder sb = new StringBuilder(); //add your code here } //Step 4 complete findCircles method as shown in instructions //Let’s now complete the findCircles method. //Start by editing this method header for findCircles so that it takes in an ArrayList of Shape objects as a parameter. //Now, using another for each loop, and the instanceof keyword add the information of ONLY Circles to a StringBuilder //(make sure every Circle Object’s info is separated by a new line). //HINT use the toString method //Finally return a string holding all of the different Circle’s information. public static String findCircles(ArrayList list) { StringBuilder strbldr = new StringBuilder(); list.forEach((shape) -> { if (shape instanceof Circle) { strbldr.append(shape).append(""); } } ); return strbldr.toString(); }}
Step by Step Solution
3.44 Rating (154 Votes )
There are 3 Steps involved in it
Step: 1
SAMPLE ...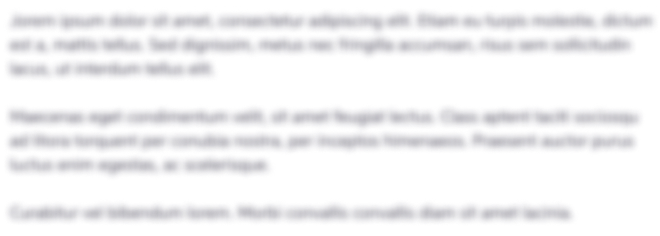
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started