Question
Java. Trouble with NullPointerException error when creating the tmpNode. I can't figure out why its Null when I have integer values for the Node. This
Java. Trouble with NullPointerException error when creating the tmpNode. I can't figure out why its Null when I have integer values for the Node. This is the line that errors tmpNode = cardList.newNode(Integer.valueOf(tokens[0]),Integer.valueOf(tokens[1]));
public static void main(String[] args) throws FileNotFoundException { Integer amount; LinkedList cardList = null; Node temp = null; Node tmpNode = null; Integer num1; Integer num2; File file = new File("input.txt"); Scanner input; input = new Scanner(file); amount = input.nextInt(); System.out.println(amount); while(input.hasNextLine()) { String value = input.nextLine(); System.out.println(value); if(value.isEmpty()) continue; String tokens[] = value.split("\\s+"); num1 = Integer.valueOf(tokens[0]); num2 = Integer.valueOf(tokens[1]); System.out.println(num1); System.out.println(num2); System.out.println(tokens[0]); System.out.println(tokens[1]); tmpNode = cardList.newNode(Integer.valueOf(tokens[0]),Integer.valueOf(tokens[1])); System.out.println(tmpNode); //cardList.InsertSortedManner(temp); } //cardList.printList(); input.close(); }
public class LinkedList {
Node headref;
class Node {
int name;
int data;
Node next;
Node(int n, int d) {
name = n;
data = d;
next = null;
}
}
//Method to insert node in Sorted Order
void InsertSortedManner(Node temp) {
Node current;
//Check if head is null or head data is less than new node data
if (headref == null || headref.data <= temp.data) {
temp.next = headref;
headref = temp;
} else {
current = headref;
//Iterate till we reach last node and my new node data is less than curr data
while (current.next != null && current.next.data >= temp.data)
current = current.next;
temp.next = current.next;
current.next = temp;
}
}
//Method that returns NewNode
Node newNode(int n, int data) {
Node x = new Node(n, data);
return x;
}
//Print list method that writes to file output.txt
void printList() {
Node temp = headref;
//buffered writer
BufferedWriter bw = null;
//FileWriter initialised to null
FileWriter fw = null;
try {
//Output will be written in output.txt
fw = new FileWriter("output.txt");
bw = new BufferedWriter(fw);
try{
while (temp != null) {
//Print name in console
System.out.println("Number: " + temp.name);
//Print Priority in console
System.out.println("Cost: " + temp.data);
//Name in file
bw.write("Name: " + temp.name);
bw.write(" ");
//Priority in file
bw.write("Priority: " + temp.data);
bw.write(" ");
temp = temp.next;
}
}catch(InputMismatchException e){
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (bw != null)
bw.close();
if (fw != null)
fw.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
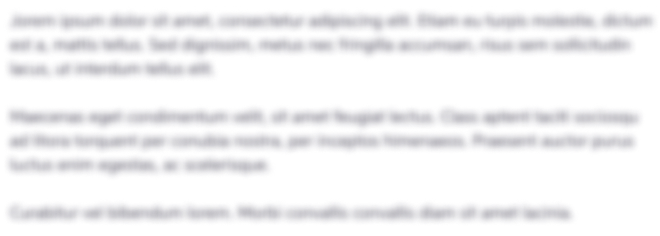
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started