Question
(Java) Use and test two array-based sorting algorithms selection and insertion sort. You will need System.nanoTime() to measure running time. Specific Guidelines 1) Create a
(Java)
Use and test two array-based sorting algorithms selection and insertion sort. You will need System.nanoTime() to measure running time.
Specific Guidelines
1) Create a new project that contains the following classes:
a) Thing class: should implement Comparable interface.
b) XSort>: includes insertion, selection sort and binary search algorithms
c) Driver: for testing
2) XSort: two main methods should be implemented: selection, insertion sort and binary search. Add any necessary private methods that are needed to help the two sort algorithms.
3) Thing class: in compareTo method, use the value as the basis of comparison
4) The Driver class should provide a menu-based interaction with the user. Menu items should be at least:
a) Generate random array of Thing objects
b) Print array
c) Insertion sort the array
d) Selection sort the array
e) Binary search the array for an element
f) Timing ON/OFF: enable/disable timing
If timing is ON, always measure time and display it after each sort option.
Please test the program and upload a screenshot of a sample test. Thanks in advance!!
Thing class which is needed for part 1:
import java.util.ArrayList; import java.util.Random; public class Thing implements ArrayBag{ private int value; private String id, color, material; //constructor public Thing( String id, int value, String color, String material) { this.id = id; this.value = value; this.color = color; this.material = material; }
//default constructor Thing(){ value = -1; id = ""; color = ""; material = ""; } //rand() - generates random number and selects values from provided list in ArrayBag public void rand(){ Random rand = new Random(); this.id = alphabetID[rand.nextInt(5)] + (rand.nextInt(1000) + 1000); this.value = rand.nextInt(1000); this.color = COLOR[rand.nextInt(5)]; this.material = MATERIAL[rand.nextInt(5)]; } @Override public void print() { System.out.println(id + " " + value + " " + color + " " + material); } @Override public String toString() { // TODO Auto-generated method stub return id + " " + value + " " + color + " " + material; } //created list and returns it public ArrayList list(){ ArrayList list = new ArrayList(); for(int i=0; i<5; i++) { Thing thing = new Thing(); thing.rand(); list.add(thing); } return list; } }
Class ArrayBag:
public interface ArrayBag { String COLOR[] = {"Blue", "Red", "Yellow", "Green", "White"}; String MATERIAL[] = {"Leather", "Wood", "Polyester", "Plastic", "Iron"}; String alphabetID[]= {"A", "B", "C", "D", "E"}; void print(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
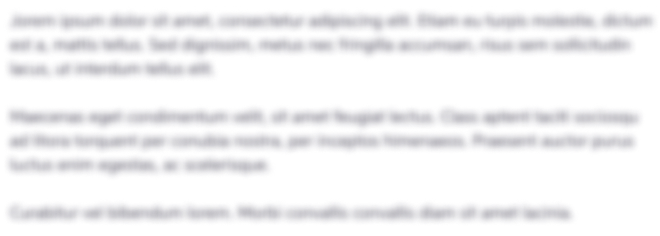
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started