Answered step by step
Verified Expert Solution
Question
1 Approved Answer
(java) Write a class that is called AddressBook, It has 5 methods: constructor, public void addContact(Contact newContact), public void deleteContact(String firstName, String lastName), public Contact[]
(java)
Write a class that is called AddressBook, It has 5 methods: constructor, public void addContact(Contact newContact),
public void deleteContact(String firstName, String lastName), public Contact[] searchByLastName(String lastName), public Contact[] list()
*In addition there will be a need for a called Contact with the following String fields: firstName, lastName, email and phone.
see below for method details.
// code to copy for testing Address class
import java.util.Scanner; import java.io.IOException; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method; /** OVERVIEW: A program that provides user with an interface to use an AddressBook */ public class AddressBookProgram { public static void main(String[] args) { AddressBook book = null; // try to open the address book try { book = new AddressBook(); } catch (IOException e) { System.out.println(e); System.out.println("Unable to read from the contact file. Program stops"); return; } String firstName, lastName, email, phone; int instruction = -1; var in = new Scanner(System.in); Contact[] result = null; Method m = null; do { System.out.println(); System.out.println("******************************************************"); System.out.println("Please select one of the following instructions (0-4):"); System.out.println(" 0. Exit"); System.out.println(" 1. Add a new contact"); System.out.println(" 2. Delete a contact"); System.out.println(" 3. Search for contacts using a last name"); System.out.println(" 33. Search for contacts using a partial last name"); System.out.println(" 4. List all contacts"); System.out.println("******************************************************"); System.out.println(); String line = in.nextLine(); try { instruction = Integer.parseInt(line); } catch (NumberFormatException e) { System.out.println("Invalid instruction!"); continue; } switch (instruction) { case 0: break; case 1: try { m = AddressBook.class.getMethod( "addContact", Contact.class); } catch (NoSuchMethodException e) { System.out.println("addContact not implement in " + "AddressBook!"); continue; } System.out.println(); System.out.println("Please enter the first name of the contact: "); firstName = in.nextLine(); if (firstName.isBlank()) { System.out.println("First name cannot be empty!"); continue; } System.out.println(); System.out.println("Please enter the last name of the contact: "); lastName = in.nextLine(); if (lastName.isBlank()) { System.out.println("Last name cannot be empty!"); continue; } System.out.println(); System.out.println("Please enter the email address of the contact: "); email = in.nextLine(); System.out.println(); System.out.println("Please enter the phone number of the contact: "); phone = in.nextLine(); var c = new Contact(); c.firstName = firstName; c.lastName = lastName; c.email = email; c.phone = phone; System.out.println(); try { try { m.invoke(book, c); } catch (IllegalAccessException | IllegalArgumentException e) { System.out.println(e); System.out.println("Invocation Error: addContact"); } catch (InvocationTargetException e) { Throwable th = e.getCause(); if (th instanceof NullPointerException) throw (NullPointerException) th; else if (th instanceof EmptyNameException) throw (EmptyNameException) th; else if (th instanceof DuplicateContactException) throw (DuplicateContactException) th; else if (th instanceof IOException) throw (IOException) th; else { System.out.println(e); System.out.println("Invocation Error: addContact"); } } //book.addContact(c); } catch (IOException e) { System.out.println(e); System.out.println("Unable to write to disk! New contact is not added."); continue; } catch (DuplicateContactException e) { System.out.println("Contact exists! New contact is not added."); continue; } System.out.println("New contact is added successfully."); break; case 2: try { m = AddressBook.class.getMethod( "deleteContact", String.class, String.class); } catch (NoSuchMethodException e) { System.out.println("deleteContact not implement in " + "AddressBook!"); continue; } System.out.println(); System.out.println("Please enter the first name of the contact to delete: "); firstName = in.nextLine(); if (firstName.isBlank()) { System.out.println("First name cannot be empty!"); continue; } System.out.println(); System.out.println("Please enter the last name of the contact to delete: "); lastName = in.nextLine(); if (lastName.isBlank()) { System.out.println("Last name cannot be empty!"); continue; } System.out.println(); try { try { m.invoke(book, firstName, lastName); } catch (IllegalAccessException | IllegalArgumentException e) { System.out.println(e); System.out.println("Invocation Error: deleteContact"); } catch (InvocationTargetException e) { Throwable th = e.getCause(); if (th instanceof NullPointerException) throw (NullPointerException) th; else if (th instanceof EmptyNameException) throw (EmptyNameException) th; else if (th instanceof ContactNotFoundException) throw (ContactNotFoundException) th; else if (th instanceof IOException) throw (IOException) th; else { System.out.println(e); System.out.println("Invocation Error: deleteContact"); } } //book.deleteContact(firstName, lastName); } catch (IOException e) { System.out.println(e); System.out.println("Unable to write to disk! Contact is not deleted"); continue; } catch (ContactNotFoundException e) { System.out.println("Contact does not exist! Contact is not deleted"); continue; } System.out.println("Contact is deleted successfully"); break; case 3: try { m = AddressBook.class.getMethod( "searchByLastName", String.class); } catch (NoSuchMethodException e) { System.out.println("searchByLastName not implement in " + "AddressBook!"); continue; } System.out.println(); System.out.println("Please enter the last name to search: "); lastName = in.nextLine(); if (lastName.isBlank()) { System.out.println("Last name cannot be empty!"); continue; } System.out.println(); try { result = (Contact[]) m.invoke(book, lastName); } catch (IllegalAccessException | IllegalArgumentException e) { System.out.println(e); System.out.println("Invocation Error: searchByLastName"); } catch (InvocationTargetException e) { Throwable th = e.getCause(); if (th instanceof NullPointerException) throw (NullPointerException) th; else if (th instanceof EmptyNameException) throw (EmptyNameException) th; else { System.out.println(e); System.out.println("Invocation Error: searchByLastName"); } } //result = book.searchByLastName(lastName); if (result.length == 0) System.out.println("There is no contact with the given last name."); else { for (var curContact : result) { System.out.println(curContact.firstName + " " + curContact.lastName); System.out.println("E-mail: " + curContact.email); System.out.println("Phone: " + curContact.phone); System.out.println(); } } break; case 33: try { m = AddressBook.class.getMethod( "searchByLastNamePartial", String.class); } catch (NoSuchMethodException e) { System.out.println("searchByLastNamePartial not implement in " + "AddressBook!"); continue; } System.out.println(); System.out.println("Please enter the partial lastname to search: "); lastName = in.nextLine(); if (lastName.isBlank()) { System.out.println("Lastname cannot be empty!"); continue; } System.out.println(); try { result = (Contact[]) m.invoke(book, lastName); } catch (IllegalAccessException | IllegalArgumentException e) { System.out.println(e); System.out.println("Invocation Error: searchByLastNamePartial"); } catch (InvocationTargetException e) { Throwable th = e.getCause(); if (th instanceof NullPointerException) throw (NullPointerException) th; else if (th instanceof EmptyNameException) throw (EmptyNameException) th; else { System.out.println(e); System.out.println("Invocation Error: searchByLastNamePartial"); } } //result = book.searchByLastNamePartial(lastName); if (result.length == 0) System.out.println("There is no contact with the given partial " + "last name."); else { for (var curContact : result) { System.out.println(curContact.firstName + " " + curContact.lastName); System.out.println("E-mail: " + curContact.email); System.out.println("Phone: " + curContact.phone); System.out.println(); } } break; case 4: try { m = AddressBook.class.getMethod("list"); } catch (NoSuchMethodException e) { System.out.println("list not implement in AddressBook!"); continue; } System.out.println(); try { result = (Contact[]) m.invoke(book); } catch (IllegalAccessException | IllegalArgumentException | InvocationTargetException e) { System.out.println(e); System.out.println("Invocation Error: list"); } //result = book.list(); if (result.length == 0) System.out.println("There is no contact in the address book."); else for (var curContact : result) { System.out.println(curContact.firstName + " " + curContact.lastName); System.out.println("E-mail: " + curContact.email); System.out.println("Phone: " + curContact.phone); System.out.println(); } break; default: System.out.println("Invalid instruction!"); } } while (instruction != 0); } }Unu vor puviiv Luuuuuuu Construct a new address book object. A contact has four fields: first name, last name, email and phone. (There could be more information for a real contact. But these are sufficient for the assignment.) The constructor reads from the disk to retrieve previously entered contacts. If previous contacts exist, the address book will be populated with those contacts retrieved from the disk. Otherwise, the address book will be empty. The constructor should notify the user if any disk I/O error occurs (IOException). Note: Since the AddressBook class should not include the user interface, the way in which the user is notified should be a correspondingly thrown exception object. A System.out.println() is NOT appropriate for this method. 2. Method: public void addContact (Contact newContact) Add a new contact into the address book: . Neither the first name nor the last name in newContact can be null or empty (NullPointerException and EmptyNameException, respectively). The method should notify the user if a contact with the same first name and last name is already in the address book (DuplicateContactException). Therefore, newContact will not be inserted. When a new contact is added into the address book, the content of the address book should be saved to the disk. The method should notify the user if any disk I/O error occurs (IOException). If a disk error does occur, the addition of the new contact should be cancelled, that is, even if the new contact was added into the address book, it should be removed from the address book upon an I/O error so that consistency is maintained between the contacts in memory and those on the disk. 3. Method: public void delete Contact (String firstName, String lastName) Delete from the address book a contact whose first name and last name are the same as the input parameters. The user needs to provide both the first name and the last name of the contact to be deleted. Neither the first name nor the last name can be null or empty (NullPointerException and EmptyNameException, respectively). The method should notify the user if a contact with the same first name and last name cannot be found in the address book ContactNotFoundException). Thus, deletion does not happen. When a contact is deleted from the address book, the content of the address book should be saved to the disk. The method should notify the user if any disk I/O error occurs (IOException). If a disk error does occur, the deletion of the contact should be cancelled, that is, even if the contact was deleted from the address book, it should be recovered in the address book upon an I/O error so that consistency is maintained between the contacts in memory and those on the disk. 4. Method: public Contact[] searchByLastName (String lastName) Search all contacts by the last name and return matching contacts as an array of contacts. There might be zero or more than one contact returned Ti The last name provided cannot be null or empty (NullPointerException and EmptyNameException, respectively). The user should be notified if the lastName argument is null or empty. 5. Method: public Contact[] list() Return all the contacts in the address book as an array of contacts. In your implementation, the contacts in the address book should be read from and written into a file named "contacts.dat". When the address book is opened again, the contacts previously added should be loaded into the address book from the file. Your code should provide specifications for all classes, constructors and methods based on the templates given in the lecture notes and the textbook (OVERVIEW, REQUIRES, MODIFIES, EFFECTS, etc.). Note that the method headers provided above do not include any throws clause. If you decide a certain exception object may be thrown from the method, the header should be modified accordingly. Whenever an exception is needed, if there is no applicable built-in Java API exception, you need to define your own exception and decide whether the exception should be checked or unchecked. Apparently, you need to define a record class called Contact with the following String fields: firstName, lastName, email and phone. Two tester programs are provided: 1. "AddressBookProgram.java" provides a command-line user interface to test your AddressBook program. You can run this tester for Address Book as you continue developing it. 2. "AddressBookTester.java" tests all methods of AddressBook without a user interface. You can run this program to test AddressBook when you finish developing all methods of AddressBook. Note: If you use NetBeans, you can add both testers to your project. To run a tester, you can right- click on the tester in the Projects view at the upper-left corner and choose "Run File" in the short- cut menu. Hints 1. Always write the specifications first. By completing a specification, you will be very clear on what to do. While writing the specification, be careful about the side effects of the methods, e.g., writing to the contact file. Pay attention to the use of exceptions and its interactions with the side effects. While you may choose either a Contact array or an ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
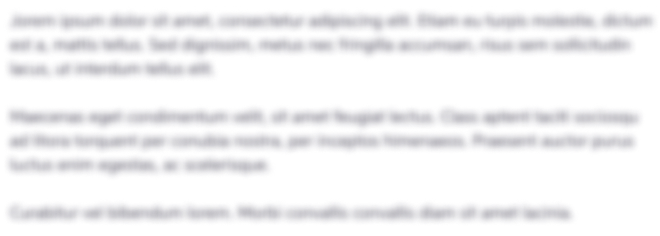
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started