Question
Java: Write a complete program consisting of a Money class and a MoneyTest class. The Money class should contain: Private attributes containing values for dollars,
Java:
Write a complete program consisting of a Money class and a MoneyTest class.
The Money class should contain:
Private attributes containing values for dollars, cents
A default constructor
A second constructor which takes initial values for dollars and cents. You should ensure these numbers are non-negative.
Accessor methods for dollars and cents
A printing method that prints out the current balance (with a $ in front)
An addMoney method that takes in some amount in dollars and cents and adds it to the current amount. Note that the value of cents should be < 100. If cents is > 100 convert 100 cents into an additional dollar.
A subtractMoney that takes in some amount in dollars and cents and removes it from the current amount IF it is less than the current amount available. Otherwise print an error message.
The MoneyTest class should complete the following outline
class MoneyTest {
public static void main(String [] args) {
// Declare a variable of type Money using your default constructor
// Print this variable using your Money printing method
// Declare a second variable of type Money with initial value $1.31
// Add $1.80 into the second Money variable
// Use your accessor method to obtain the cents value for the second
// Money variable
// Try subtracting $5 from the current total (which should give an error)
// Subtract $1.50 from the current total
// Print this variable using your printing method
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
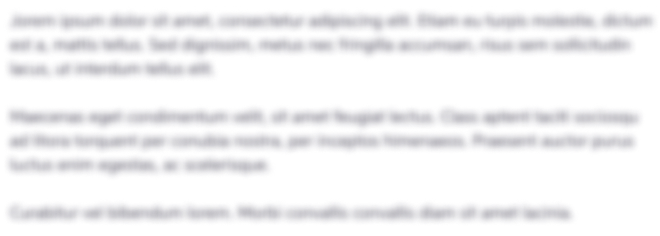
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started