Question
JAVA Write a program called ShuffleDeck. This will simulate randomly shuffling a deck of cards. The cards will be represented by strings found in the
JAVA Write a program called ShuffleDeck. This will simulate randomly shuffling a deck of cards. The cards will be represented by strings found in the file deckofcards.txt. Begin by reading these strings into a queue of strings. Call a method with the signature:
public static void shuffle(Queue deck)
This method performs the following steps:
- Compute a value called the shuffle count that is the base 2 log of the size of the deck
- In a loop that executes shuffle count times:
- Create two queues left and right
- While the deck queue is not empty:
- Dequeue a card from the deck
- Flip a coin
- If heads comes up enqueue that card on left otherwise enqueue it on right
- Dequeue every card from left and enqueue it on deck
- Do the same for right
- return
Upon returning from the method, print out the deck, one card to a line.
The queue objects you use should come from one of these two classes:
- Queue in the algs4 package
- LinkedListQueue in the week4inclass package
Do NOT copy the code from those classes into your program. DO declare variables and then call the various instance methods as needed. Here's an example of a declaration:
LinkedListQueue deck = new LinkedListQueue<>();
So I've been working on this and my code is a mess. Lots of errors and I'm missing a few steps. Here is what I have, so if anyone can help get me over the finish line I'd be much obliged.
package assignment4;
import algs4.*;
public class ShuffleDeck { public static void main(String[]args) { //read strings into a queue of strings StdIn.fromFile("data/deckofcards.txt"); String queue=StdIn.readAll(); }
public static void shuffle(Queue deck) {
while (!deck.isEmpty()) { String first = deck.dequeue(); // dequeues from the deck //int flip = r.nextInt(2); if (flip == 1) { leftDeck.enqueue(first); // takes the value from the dequeue & puts on right deck } else { rightDeck.enqueue(first); // takes the value from the dequeue & puts on right deck } } while (!leftDeck.isEmpty()) { // dequeue left putting back to main String next = leftDeck.dequeue(); deck.enqueue(next); } while (!rightDeck.isEmpty()) { // dequeue right putting back to main String last = rightDeck.dequeue(); deck.enqueue(last); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
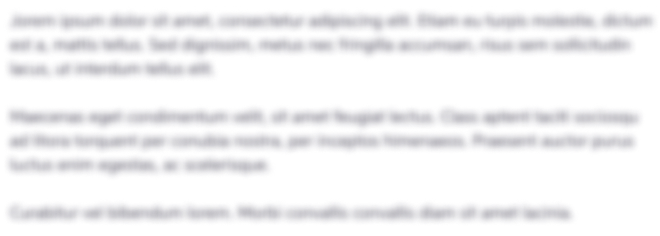
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started