Question
JavaFx program to convert decimal to binary,decimal to hex, hex to decimal, binary to decimal.Recursive methods. Have written the program but keep getting Exceptions. Can
JavaFx program to convert decimal to binary,decimal to hex, hex to decimal, binary to decimal.Recursive methods. Have written the program but keep getting Exceptions. Can anyone help me with this code, as my brainpower is dropping by the second :) Code is rovided below:
public class Converter extends Application{
private double paneWidth=500;
private double paneHeight=100;
@Override // Override startmethod
public void start(Stage primaryStage) {
TextField tfInput = new TextField(); // Inputfield
tfInput.setPromptText("Number to be converted..."); // Prompttext background tf
tfInput.getText();
TextField tfOutput = new TextField(); // Outputfield
Button btdecBin = new Button("Decimal to Binary");
Button btdecHex = new Button("Decimal to Hex");
Button btbinDec = new Button("Binary to Decimal");
Button bthexDec = new Button("Hex to Decimal");
HBox buttonBox = new HBox();
buttonBox.getChildren().addAll(btdecBin, btdecHex,bthexDec,btbinDec);
buttonBox.setAlignment(Pos.CENTER);
BorderPane buttons = new BorderPane();
buttons.setTop(buttonBox);
// New pane, add textfield,textarea and button
GridPane pane = new GridPane();
pane.setAlignment(Pos.CENTER);
pane.add(tfInput, 0, 3);
pane.add(tfOutput, 0, 4);
pane.add(buttons, 0, 0);
// Create a scene and place it in the stage
Scene scene = new Scene(pane, paneWidth, paneHeight);
primaryStage.setTitle("Converter"); // Set the stage title
primaryStage.setResizable(false);// No resizable
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
int value = Integer.parseInt(tfInput.getText());
String hexString = tfInput.getText();
String binary = tfInput.getText();
// Eventhandler for button DECIMAL TO BINARY
btdecBin.setOnAction(new EventHandler
public void handle(ActionEvent event) {
tfOutput.appendText("The Binary equivalent of "+value+" is "+ dec2Bin(value));
}});
// Eventhandler for button DECIMAL TO HEX
btdecHex.setOnAction(new EventHandler
public void handle(ActionEvent event) {
tfOutput.appendText("The HEX equivalent of "+value+" is "+ dec2Hex(value));
}});
// Eventhandler for button BINARY TO DECIMAL
btbinDec.setOnAction(new EventHandler
public void handle(ActionEvent event) {
tfOutput.appendText("The equivalent of "+binary+" is "+ bin2Dec(binary));
}});
//Eventhandler for button HEX TO DECIMAL
bthexDec.setOnAction(new EventHandler
public void handle(ActionEvent event) {
tfOutput.appendText("The Decimal equivalent of "+hexString+" is "+ hex2Dec(hexString));
}});}
//method for converting decimal to binary
private String dec2Bin(int value) {
String result= "";
return dec2Bin(value,result);
}
//Helpermethod
private String dec2Bin(int value,String result){
if (value/2 == 0){ // Basecase
return (value%2)+result;
}
else
return dec2Bin(value/2,(value%2)+result);
}
// Method converting decimal to hex
private String dec2Hex(int value) {
String result = "";
return dec2Hex(value, result);
}
/** Recursive helper method */
private String dec2Hex(int value, String result) {
int r = value % 16; // Remainder
String remainder = r >= 10 ?
String.valueOf((char)('A' + r % 10)) : String.valueOf(r);
if (value / 16 == 0) // Base case
return remainder + result;
else
return dec2Hex(value / 16, remainder + result); // Recursive call
}
/** Method parses a hex number as
* string into a decimal integer */
private int hex2Dec(String hexString) {
int result = 0;
int index = 0;
int n = hexString.length() - 1;
return hex2Dec(hexString, index, n, result);
}
/** Recursive helper method */
private int hex2Dec(String hexString, int index, int n, int result) {
int dec = 0; // Decimal conversion
if (hexString.charAt(index) >= 'A' &&
hexString.charAt(index)
dec = (hexString.charAt(index) - 'A') + 10;
}
else {
dec = Integer.parseInt(String.valueOf(hexString.charAt(index)));
}
dec *= (int)Math.pow(16, n);
if (n == 0) // Base Case
return result + dec;
else // Recursive call
return hex2Dec(hexString, index + 1, n - 1, dec + result);
}
private int bin2Dec(String s) {
int i = (s.charAt(0) == '1') ? 1 : 0;
if (s.length() == 1)
return i;
return (int)Math.pow(2, s.length() - 1) * i + bin2Dec(s.substring(1));
}
public static void main(String[] args) { // Main method
System.out.println("");
launch(args);
}}
Converter Decimal to Binary Decimal to Hex Hex to Decimal Binary to Decimal Number to be convertedStep by Step Solution
There are 3 Steps involved in it
Step: 1
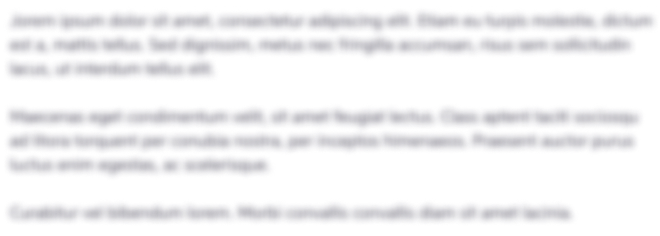
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started